지구정복
[JSP] 1/7 | MVC Model - URL로 컨트롤러 구현하기(예제, 우편번호검색기, 기본게시판, 페이지있고 이모티콘 포함된 게시판), 표현언어(EL개념 및 기본사용, 자료실게시판만들기) 본문
[JSP] 1/7 | MVC Model - URL로 컨트롤러 구현하기(예제, 우편번호검색기, 기본게시판, 페이지있고 이모티콘 포함된 게시판), 표현언어(EL개념 및 기본사용, 자료실게시판만들기)
nooh._.jl 2021. 1. 7. 17:15복습
Servlet 활용방안
=> MVC Model2 Pattern
M - beans가 처리
V - jsp(데이터와는 직접연결이 없고 beans에서 처리된 결과만 받는다.)
C - controller
(요청받으면 m/v에게 넘겨주고 결과를 받아서 사용자에게 전달)
게시판만들기
- Controller
- BoardAction
ListAction
~
DeleteOkAction
- beans(모델)
BoardDAO
BoardTO
컨트롤러 구현방식
1. 파라메터 방식
controller?파라메터=기능
2. url 방식
기능.확장자
*.do - do를 많이 사용한다.
*.naver
와 같이 확장자를 정해줘야 한다.
view1.do를 요청하면 view1.jsp를 보여준다.
view2.do를 요청하면 view2.jsp를 보여준다.
ㅇ컨트롤러 구현방식 ( URL이용 )
새로운 다이나믹웹프로젝트 만든다.
WEB-INF안에 views폴더만들고 view1.jsp, view2.jsp 파일을 만든다.
그리고 자바리소스에서 servlet 패키지를 만들고 그 안에 서블릿클래스를 만드는데 이때 URL 매핑을 아래와같이
*.do 로 만들어준다.
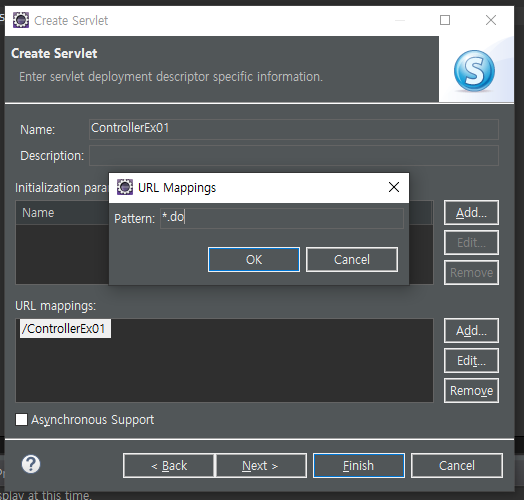
-ControllerEx01.java
package servlet;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("*.do")
public class ControllerEx01 extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
this.doProcess(request, response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
this.doProcess(request, response);
}
protected void doProcess(HttpServletRequest request, HttpServletResponse response) {
//브라우저에서 실행예시
//http://loaclhost:8080/프로젝트명/view1.do - view1.jsp 출력
//http://loaclhost:8080/프로젝트명/view2.do - view2.jsp 출력
//System.out.println( request.getRequestURI() );
//System.out.println( request.getContextPath() );
try {
request.setCharacterEncoding( "utf-8" );
String path = request.getRequestURI().replaceAll( request.getContextPath(), "" );
String url = "";
if ( path.equals( "/*.do" ) || path.equals( "/view1.do" ) ) {
url = "/WEB-INF/views/view1.jsp";
} else if ( path.equals( "/view2.do" ) ) {
url = "/WEB-INF/views/view2.jsp";
} else {
}
RequestDispatcher dispatcher = request.getRequestDispatcher( url );
dispatcher.forward( request, response );
} catch (UnsupportedEncodingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (ServletException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
이제 브라우저에서 view1.do 또는 *.do를 쓰면 view1.jsp를, view2.do를 쓰면 view2.jsp를 출력하게 된다.



ㅇ우편번호검색기 url로 바꾸기
-ControllerEx01.java
package servlet;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import model2.ActionModel;
import model2.ZipcodeAction;
import model2.ZipcodeOkAction;
@WebServlet("*.do")
public class ControllerEx01 extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
this.doProcess(request, response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
this.doProcess(request, response);
}
protected void doProcess(HttpServletRequest request, HttpServletResponse response) {
try {
request.setCharacterEncoding( "utf-8" );
String action= request.getRequestURI().replaceAll( request.getContextPath(), "" );
String url = "";
ActionModel model = null;
if ( action.equals("/*.do") || action.equals( "/zipcode.do" ) ) {
model = new ZipcodeAction();
model.execute(request, response);
url = "/WEB-INF/views/zipcode.jsp";
} else if ( action.equals( "/zipcode_ok.do" ) ) {
model = new ZipcodeOkAction();
model.execute(request, response);
url = "/WEB-INF/views/zipcode_ok.jsp";
}
RequestDispatcher dispatcher = request.getRequestDispatcher( url );
dispatcher.forward(request, response);
} catch (UnsupportedEncodingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (ServletException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
-zipcode.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="model1.ZipcodeTO" %>
<%@ page import="model1.ZipcodeDAO" %>
<%@ page import="java.util.ArrayList" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script type="text/javascript">
const checkfrm = function() {
if( document.frm.dong.value.trim() == '' ) {
alert( '동이름을 입력해 주세요' );
return;
}
document.frm.submit();
};
</script>
</head>
<body>
<form action="./zipcode_ok.do" method="post" name="frm">
<input type="hidden" name="action" value="zipcode_ok" />
동이름 <input type="text" name="dong" />
<input type="button" value="동이름 검색" onclick="checkfrm()" />
</form>
</body>
</html>
ㅇ게시판 만들기 (URL 방식)
게시판
list.do board_list1.jsp
ListAction
view.do board_view1.jsp
ViewAction
write.do board_write1.jsp
WriteAction
write_ok.do board_write1_ok.jsp
WriteOkAction
modify.do board_modify1.jsp
ModifyAction
modify_ok.do board_modify1_ok.jsp
ModifyOkAction
delete.do board_delete1.jsp
DeleteAction
delete_ok.do board_delete1_ok.jsp
DeleteOkAction
BoardTO와 BoardDAO는 어제 코드와 같다.
-controllerEx01.java
package servlet;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import model2.BoardAction;
import model2.DeleteAction;
import model2.DeleteOkAction;
import model2.ListAction;
import model2.ModifyAction;
import model2.ModifyOkAction;
import model2.ViewAction;
import model2.WriteAction;
import model2.WriteOkAction;
/**
* Servlet implementation class ControllerEx01
*/
@WebServlet("*.do")
public class controllerEx01 extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
this.doProcess(request, response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
this.doProcess(request, response);
}
protected void doProcess(HttpServletRequest request, HttpServletResponse response) {
try {
request.setCharacterEncoding( "utf-8" );
String action= request.getRequestURI().replaceAll( request.getContextPath(), "" );
String url = "";
BoardAction boardAction = null;
if ( action == null || action.equals("/*.do") || action.equals( "/list.do" ) ) {
boardAction = new ListAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_list1.jsp";
} else if ( action.equals( "/view.do" ) ) {
boardAction = new ViewAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_view1.jsp";
} else if ( action.equals( "/write.do" ) ) {
boardAction = new WriteAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_write1.jsp";
} else if ( action.equals( "/write_ok.do" ) ) {
boardAction = new WriteOkAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_write1_ok.jsp";
} else if ( action.equals( "/modify.do" ) ) {
boardAction = new ModifyAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_modify1.jsp";
} else if ( action.equals( "/modify_ok.do" ) ) {
boardAction = new ModifyOkAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_modify1_ok.jsp";
} else if ( action.equals( "/delete.do" ) ) {
boardAction = new DeleteAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_delete1.jsp";
} else if ( action.equals( "/delete_ok.do" ) ) {
boardAction = new DeleteOkAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_delete1_ok.jsp";
}
RequestDispatcher dispatcher = request.getRequestDispatcher( url );
dispatcher.forward(request, response);
} catch (UnsupportedEncodingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (ServletException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
-board_list1.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="model1.BoardTO" %>
<%@ page import="model1.BoardDAO" %>
<%@ page import="java.util.ArrayList" %>
<%
ArrayList<BoardTO> lists = (ArrayList)request.getAttribute( "lists" );
int totalRecord = lists.size();
StringBuffer sbHtml = new StringBuffer();
for( BoardTO to : lists ) {
String seq = to.getSeq();
String subject = to.getSubject();
String writer = to.getWriter();
String wdate = to.getWdate();
String hit = to.getHit();
int wgap = to.getWgap();
sbHtml.append( " <tr> " );
sbHtml.append( " <td> </td> " );
sbHtml.append( " <td>" + seq + "</td> " );
sbHtml.append( " <td class='left'> ");
sbHtml.append( " <a href='./view.do?seq=" + seq + "'>" + subject + "</a> ");
if( wgap == 0 ) {
sbHtml.append( " <img src='./images/icon_hot.gif' alt='HOT'> ");
}
sbHtml.append( " </td> " );
sbHtml.append( " <td>" + writer + "</td> " );
sbHtml.append( " <td>" + wdate + "</td> " );
sbHtml.append( " <td>" + hit + "</td> " );
sbHtml.append( " <td> </td> " );
sbHtml.append( " </tr> " );
}
%>
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0,minimum-scale=1.0,maximum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<title>Insert title here</title>
<link rel="stylesheet" type="text/css" href="./css/board_list.css">
</head>
<body>
<!-- 상단 디자인 -->
<div class="con_title">
<h3>게시판</h3>
<p>HOME > 게시판 > <strong>게시판</strong></p>
</div>
<div class="con_txt">
<div class="contents_sub">
<div class="board_top">
<div class="bold">총 <span class="txt_orange"><%= totalRecord %></span>건</div>
</div>
<!--게시판-->
<div class="board">
<table>
<tr>
<th width="3%"> </th>
<th width="5%">번호</th>
<th>제목</th>
<th width="10%">글쓴이</th>
<th width="17%">등록일</th>
<th width="5%">조회</th>
<th width="3%"> </th>
</tr>
<%= sbHtml %>
</table>
</div>
<!--//게시판-->
<div class="align_right">
<input type="button" value="쓰기" class="btn_write btn_txt01" style="cursor: pointer;" onclick="location.href='./write.do'" />
</div>
</div>
</div>
<!--//하단 디자인 -->
</body>
</html>
-board_write1.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0,minimum-scale=1.0,maximum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<title>Insert title here</title>
<link rel="stylesheet" type="text/css" href="./css/board_write.css">
<script type="text/javascript">
window.onload = function() {
document.getElementById( 'submit1' ).onclick = function() {
if ( document.wfrm.info.checked == false ) {
alert( '동의를 해주세요' );
return false;
}
if ( document.wfrm.writer.value.trim() == '' ) {
alert( '글쓴이를 입력해주세요' );
return false;
}
if ( document.wfrm.subject.value.trim() == '' ) {
alert( '제목을 입력해주세요' );
return false;
}
if ( document.wfrm.password.value.trim() == '' ) {
alert( '비밀번호를 입력해주세요' );
return false;
}
document.wfrm.submit();
}
}
</script>
</head>
<body>
<!-- 상단 디자인 -->
<div class="con_title">
<h3>게시판</h3>
<p>HOME > 게시판 > <strong>게시판</strong></p>
</div>
<div class="con_txt">
<form action="./write_ok.do" method="post" name="wfrm">
<!-- <input type="hidden" name="action" value="/write_ok.do" /> -->
<div class="contents_sub">
<!--게시판-->
<div class="board_write">
<table>
<tr>
<th class="top">글쓴이</th>
<td class="top" colspan="3"><input type="text" name="writer" value="" class="board_view_input_mail" maxlength="5" /></td>
</tr>
<tr>
<th>제목</th>
<td colspan="3"><input type="text" name="subject" value="" class="board_view_input" /></td>
</tr>
<tr>
<th>비밀번호</th>
<td colspan="3"><input type="password" name="password" value="" class="board_view_input_mail"/></td>
</tr>
<tr>
<th>내용</th>
<td colspan="3"><textarea name="content" class="board_editor_area"></textarea></td>
</tr>
<tr>
<th>이메일</th>
<td colspan="3"><input type="text" name="mail1" value="" class="board_view_input_mail"/> @ <input type="text" name="mail2" value="" class="board_view_input_mail"/></td>
</tr>
</table>
<table>
<tr>
<br />
<td style="text-align:left;border:1px solid #e0e0e0;background-color:f9f9f9;padding:5px">
<div style="padding-top:7px;padding-bottom:5px;font-weight:bold;padding-left:7px;font-family: Gulim,Tahoma,verdana;">※ 개인정보 수집 및 이용에 관한 안내</div>
<div style="padding-left:10px;">
<div style="width:97%;height:95px;font-size:11px;letter-spacing: -0.1em;border:1px solid #c5c5c5;background-color:#fff;padding-left:14px;padding-top:7px;">
1. 수집 개인정보 항목 : 회사명, 담당자명, 메일 주소, 전화번호, 홈페이지 주소, 팩스번호, 주소 <br />
2. 개인정보의 수집 및 이용목적 : 제휴신청에 따른 본인확인 및 원활한 의사소통 경로 확보 <br />
3. 개인정보의 이용기간 : 모든 검토가 완료된 후 3개월간 이용자의 조회를 위하여 보관하며, 이후 해당정보를 지체 없이 파기합니다. <br />
4. 그 밖의 사항은 개인정보취급방침을 준수합니다.
</div>
</div>
<div style="padding-top:7px;padding-left:5px;padding-bottom:7px;font-family: Gulim,Tahoma,verdana;">
<input type="checkbox" name="info" value="1" class="input_radio"> 개인정보 수집 및 이용에 대해 동의합니다.
</div>
</td>
</tr>
</table>
</div>
<div class="btn_area">
<div class="align_left">
<input type="button" value="목록" class="btn_list btn_txt02" style="cursor: pointer;" onclick="location.href='./list.do'" />
</div>
<div class="align_right">
<input type="button" id="submit1" value="쓰기" class="btn_write btn_txt01" style="cursor: pointer;" />
</div>
</div>
<!--//게시판-->
</div>
</form>
</div>
<!-- 하단 디자인 -->
</body>
</html>
-board_write1_ok.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="model1.BoardTO" %>
<%@ page import="model1.BoardDAO" %>
<%
request.setCharacterEncoding("utf-8");
int flag = (Integer)request.getAttribute( "flag" );
out.println( " <script type='text/javascript'> " );
if( flag == 0 ) {
out.println( " alert('글쓰기에 성공했습니다.'); " );
out.println( " location.href='./list.do' " );
} else {
out.println( " alert('글쓰기에 실패했습니다.'); " );
out.println( " history.back(); " );
}
out.println( " </script> " );
%>
-board_view1.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="model1.BoardTO" %>
<%@ page import="model1.BoardDAO" %>
<%
request.setCharacterEncoding( "utf-8" );
BoardTO to = (BoardTO)request.getAttribute( "to" );
%>
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0,minimum-scale=1.0,maximum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<title>Insert title here</title>
<link rel="stylesheet" type="text/css" href="./css/board_view.css">
</head>
<body>
<!-- 상단 디자인 -->
<div class="con_title">
<h3>게시판</h3>
<p>HOME > 게시판 > <strong>게시판</strong></p>
</div>
<div class="con_txt">
<div class="contents_sub">
<!--게시판-->
<div class="board_view">
<table>
<tr>
<th width="10%">제목</th>
<td width="60%"><%= to.getSubject() %></td>
<th width="10%">등록일</th>
<td width="20%"><%= to.getWdate() %></td>
</tr>
<tr>
<th>글쓴이</th>
<td><%= to.getWriter() %>(<%= to.getMail() %>)(<%= to.getWip() %>)</td>
<th>조회</th>
<td><%= to.getHit() %></td>
</tr>
<tr>
<td colspan="4" height="200" valign="top" style="padding: 20px; line-height: 160%"><%= to.getContent() %></td>
</tr>
</table>
</div>
<div class="btn_area">
<div class="align_left">
<input type="button" value="목록" class="btn_list btn_txt02" style="cursor: pointer;" onclick="location.href='./list.do'" />
</div>
<div class="align_right">
<input type="button" value="수정" class="btn_list btn_txt02" style="cursor: pointer;" onclick="location.href='./modify.do?seq=<%= to.getSeq() %>'" />
<input type="button" value="삭제" class="btn_list btn_txt02" style="cursor: pointer;" onclick="location.href='./delete.do?seq=<%= to.getSeq() %>'" />
<input type="button" value="쓰기" class="btn_write btn_txt01" style="cursor: pointer;" onclick="location.href='./write.do'" />
</div>
</div>
<!--//게시판-->
</div>
</div>
<!-- 하단 디자인 -->
</body>
</html>
-board_delete1.jsp
<%@page import="model1.BoardDAO"%>
<%@page import="model1.BoardTO"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="model1.BoardTO" %>
<%@ page import="model1.BoardDAO" %>
<%
request.setCharacterEncoding( "utf-8" );
BoardTO to = (BoardTO)request.getAttribute( "to" );
String seq = to.getSeq();
String subject = to.getSubject();
String writer = to.getWriter();
%>
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0,minimum-scale=1.0,maximum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<title>Insert title here</title>
<link rel="stylesheet" type="text/css" href="./css/board_write.css">
<script type="text/javascript">
window.onload = function() {
document.getElementById( 'submit1' ).onclick = function() {
if( document.dfrm.password.value.trim() == '' ) {
alert( '비밀번호를 입력해주세요' );
return false;
}
document.dfrm.submit();
}
}
</script>
</head>
<body>
<!-- 상단 디자인 -->
<div class="con_title">
<h3>게시판</h3>
<p>HOME > 게시판 > <strong>게시판</strong></p>
</div>
<div class="con_txt">
<form action="./delete_ok.do" method="post" name="dfrm">
<!-- <input type="hidden" name="action" value="delete_ok" /> -->
<input type="hidden" name="seq" value="<%= seq %>">
<div class="contents_sub">
<!--게시판-->
<div class="board_write">
<table>
<tr>
<th class="top">글쓴이</th>
<td class="top" colspan="3"><input type="text" name="writer" value="<%= writer %>" class="board_view_input_mail" maxlength="5" readonly/></td>
</tr>
<tr>
<th>제목</th>
<td colspan="3"><input type="text" name="subject" value="<%= subject %>" class="board_view_input" readonly/></td>
</tr>
<tr>
<th>비밀번호</th>
<td colspan="3"><input type="password" name="password" value="" class="board_view_input_mail"/></td>
</tr>
</table>
</div>
<div class="btn_area">
<div class="align_left">
<input type="button" value="목록" class="btn_list btn_txt02" style="cursor: pointer;" onclick="location.href='./list.do'" />
<input type="button" value="보기" class="btn_list btn_txt02" style="cursor: pointer;" onclick="location.href='./view.do?seq=<%= seq %>'" />
</div>
<div class="align_right">
<input type="button" id="submit1" value="삭제" class="btn_write btn_txt01" style="cursor: pointer;" />
</div>
</div>
<!--//게시판-->
</div>
</form>
</div>
<!-- 하단 디자인 -->
</body>
</html>
-board_delete1_ok.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="model1.BoardTO" %>
<%@ page import="model1.BoardDAO" %>
<%
request.setCharacterEncoding( "utf-8" );
int flag = (Integer)request.getAttribute( "flag" );
out.println( " <script type='text/javascript'> " );
if( flag == 0 ) {
out.println( " alert('글삭제에 성공했습니다.'); " );
out.println( " location.href='./list.do' " );
} else if ( flag == 1 ) {
out.println( " alert('비밀번호가 틀립니다.'); " );
out.println( " history.back(); " );
} else {
out.println( " alert('글삭제에 실패했습니다.'); " );
out.println( " history.back(); " );
}
out.println( " </script> " );
%>
-board_modify1.jsp
<%@page import="model1.BoardDAO"%>
<%@page import="model1.BoardTO"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="model1.BoardTO" %>
<%@ page import="model1.BoardDAO" %>
<%
request.setCharacterEncoding( "utf-8" );
BoardTO to = (BoardTO)request.getAttribute( "to" );
String seq = to.getSeq();
String writer = to.getWriter();
String subject = to.getSubject();
String content = to.getContent();
String mail[] = null;
if ( to.getMail().equals("") ) {
mail = new String[] { "", "" };
} else {
mail = to.getMail().split( "@" );
}
%>
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0,minimum-scale=1.0,maximum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<title>Insert title here</title>
<link rel="stylesheet" type="text/css" href="./css/board_write.css">
<script type="text/javascript">
window.onload = function() {
document.getElementById( 'submit2' ).onclick = function() {
if ( document.mfrm.subject.value.trim() == '' ) {
alert( '제목을 입력해주세요' );
return false;
}
if ( document.mfrm.password.value.trim() == '' ) {
alert( '비밀번호를 입력해주세요' );
return false;
}
if ( document.mfrm.content.value.trim() == '' ) {
alert( '내용을 입력해주세요' );
return false;
}
document.mfrm.submit();
}
}
</script>
</head>
<body>
<!-- 상단 디자인 -->
<div class="con_title">
<h3>게시판</h3>
<p>HOME > 게시판 > <strong>게시판</strong></p>
</div>
<div class="con_txt">
<form action="./modify_ok.do" method="post" name="mfrm">
<!-- <input type="hidden" name="action" value="modify_ok" /> -->
<input type="hidden" name="seq" value="<%= seq %>">
<div class="contents_sub">
<!--게시판-->
<div class="board_write">
<table>
<tr>
<th class="top">글쓴이</th>
<td class="top" colspan="3"><input type="text" name="writer" value="<%= writer %>" class="board_view_input_mail" maxlength="5" readonly/></td>
</tr>
<tr>
<th>제목</th>
<td colspan="3"><input type="text" name="subject" value="<%= subject %>" class="board_view_input" /></td>
</tr>
<tr>
<th>비밀번호</th>
<td colspan="3"><input type="password" name="password" value="" class="board_view_input_mail"/></td>
</tr>
<tr>
<th>내용</th>
<td colspan="3"><textarea name="content" class="board_editor_area"><%= content %></textarea></td>
</tr>
<tr>
<th>이메일</th>
<td colspan="3"><input type="text" name="mail1" value="<%= mail[0] %>" class="board_view_input_mail"/> @ <input type="text" name="mail2" value="<%= mail[1] %>" class="board_view_input_mail"/></td>
</tr>
</table>
</div>
<div class="btn_area">
<div class="align_left">
<input type="button" value="목록" class="btn_list btn_txt02" style="cursor: pointer;" onclick="location.href='./list.do'" />
<input type="button" value="보기" class="btn_list btn_txt02" style="cursor: pointer;" onclick="location.href='./view.do?seq=<%=seq %>'" />
</div>
<div class="align_right">
<input type="button" id="submit2" value="수정" class="btn_write btn_txt01" style="cursor: pointer;" />
</div>
</div>
<!--//게시판-->
</div>
</form>
</div>
<!-- 하단 디자인 -->
</body>
</html>
-board_modify1_ok.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="model1.BoardTO" %>
<%@ page import="model1.BoardDAO" %>
<%
request.setCharacterEncoding( "utf-8" );
int flag = (Integer)request.getAttribute( "flag" );
String seq = (String)request.getAttribute( "seq" );
out.println( " <script type='text/javascript'> " );
if( flag == 0 ) {
out.println( " alert('글수정에 성공했습니다.'); " );
out.println( " location.href='./view.do?seq=" + seq + "' " );
} else if ( flag == 1 ) {
out.println( " alert('비밀번호가 틀립니다.'); " );
out.println( " history.back(); " );
} else {
out.println( " alert('글수정에 실패했습니다.'); " );
out.println( " history.back(); " );
}
out.println( " </script> " );
%>
ㅇ페이지있고 이모티콘있는 게시판 만들기(Model2 url이용)
-BoardTO.java
package model1;
public class BoardTO {
private String seq;
private String subject;
private String writer;
private String mail;
private String password;
private String content;
private String hit;
private String wip;
private String wdate;
private String emot;
private int wgap;
public String getSeq() {
return seq;
}
public String getSubject() {
return subject;
}
public String getWriter() {
return writer;
}
public String getMail() {
return mail;
}
public String getPassword() {
return password;
}
public String getContent() {
return content;
}
public String getHit() {
return hit;
}
public String getWip() {
return wip;
}
public String getWdate() {
return wdate;
}
public String getEmot() {
return emot;
}
public int getWgap() {
return wgap;
}
public void setSeq(String seq) {
this.seq = seq;
}
public void setSubject(String subject) {
this.subject = subject;
}
public void setWriter(String writer) {
this.writer = writer;
}
public void setMail(String mail) {
this.mail = mail;
}
public void setPassword(String password) {
this.password = password;
}
public void setContent(String content) {
this.content = content;
}
public void setHit(String hit) {
this.hit = hit;
}
public void setWip(String wip) {
this.wip = wip;
}
public void setWdate(String wdate) {
this.wdate = wdate;
}
public void setEmot(String emot) {
this.emot = emot;
}
public void setWgap(int wgap) {
this.wgap = wgap;
}
}
-BoardListTO.java
package model1;
import java.util.ArrayList;
public class BoardListTO {
private int cpage;
private int recordPerPage;
private int blockPerPage;
private int totalPage;
private int totalRecord;
private int startBlock;
private int endBlock;
private int blockRecord;
private ArrayList<BoardTO> boardLists;
public BoardListTO() {
this.cpage = 1;
this.recordPerPage = 10;
this.blockPerPage = 5;
this.totalPage = 1;
this.totalRecord = 0;
this.blockRecord = 0;
}
public int getBlockRecord() {
return blockRecord;
}
public int getCpage() {
return cpage;
}
public int getRecordPerPage() {
return recordPerPage;
}
public int getBlockPerPage() {
return blockPerPage;
}
public int getTotalPage() {
return totalPage;
}
public int getTotalRecord() {
return totalRecord;
}
public int getStartBlock() {
return startBlock;
}
public int getEndBlock() {
return endBlock;
}
public ArrayList<BoardTO> getBoardLists() {
return boardLists;
}
public void setCpage(int cpage) {
this.cpage = cpage;
}
public void setRecordPerPage(int recordPerPage) {
this.recordPerPage = recordPerPage;
}
public void setBlockPerPage(int blockPerPage) {
this.blockPerPage = blockPerPage;
}
public void setTotalPage(int totalPage) {
this.totalPage = totalPage;
}
public void setTotalRecord(int totalRecord) {
this.totalRecord = totalRecord;
}
public void setStartBlock(int startBlock) {
this.startBlock = startBlock;
}
public void setEndBlock(int endBlock) {
this.endBlock = endBlock;
}
public void setBlockRecord(int blockRecord) {
this.blockRecord = blockRecord;
}
public void setBoardLists(ArrayList<BoardTO> boardLists) {
this.boardLists = boardLists;
}
}
-BoardDAO.java
package model1;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import javax.naming.Context;
import javax.naming.InitialContext;
import javax.naming.NamingException;
import javax.sql.DataSource;
public class BoardDAO {
private DataSource dataSource;
public BoardDAO() {
try {
Context initCtx = new InitialContext();
Context envCtx = (Context)initCtx.lookup( "java:comp/env" );
this.dataSource = (DataSource)envCtx.lookup( "jdbc/mariadb2" );
} catch (NamingException e) {
System.out.println( "[error] : " + e.getMessage() );
}
}
//write
public void boardWrite() {
}
//write_ok
public int boardWriteOk( BoardTO to ) {
Connection conn = null;
PreparedStatement pstmt = null;
//정상처리 또는 비정상처리 변수
int flag = 1;
try {
conn = dataSource.getConnection();
//데이터베이스에 데이터 집어넣기
String sql = "insert into board2 values (0, ?, ?, ?, ?, ?, 0, ?, now(), ?)";
pstmt = conn.prepareStatement(sql);
pstmt.setString( 1, to.getSubject() );
pstmt.setString( 2, to.getWriter() );
pstmt.setString( 3, to.getMail() );
pstmt.setString( 4, to.getPassword() );
pstmt.setString( 5, to.getContent() );
pstmt.setString( 6, to.getWip() );
pstmt.setString( 7, to.getEmot() );
int result = pstmt.executeUpdate();
if ( result == 1 ) {
flag = 0;
}
} catch (SQLException e) {
System.out.println( "error : " + e.getMessage() );
} finally {
if ( pstmt != null ) try { pstmt.close(); } catch ( SQLException e ) {}
if ( conn != null ) try { conn.close(); } catch ( SQLException e ) {}
}
return flag;
}
//list
public ArrayList<BoardTO> boardList() {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
ArrayList<BoardTO> lists = new ArrayList<BoardTO>();
try {
conn = dataSource.getConnection();
String sql = "select emot, seq, subject, writer, date_format(wdate, '%Y-%m-%d') wdate, hit, datediff(now(), wdate) wgap from board2 order by seq desc";
pstmt = conn.prepareStatement( sql, ResultSet.TYPE_SCROLL_INSENSITIVE, ResultSet.CONCUR_READ_ONLY );
rs = pstmt.executeQuery();
//데이터베이스에서 글목록 가져와서 리스트 나타내기
while( rs.next() ) {
BoardTO to = new BoardTO();
String emot = rs.getString( "emot" );
String seq = rs.getString( "seq" );
String subject = rs.getString( "subject" );
String writer = rs.getString( "writer" );
String wdate = rs.getString( "wdate" );
String hit = rs.getString( "hit" );
int wgap = rs.getInt( "wgap" );
to.setEmot(emot);
to.setSeq(seq);
to.setSubject(subject);
to.setWriter(writer);
to.setWdate(wdate);
to.setHit(hit);
to.setWgap(wgap);
lists.add( to );
}
} catch (SQLException e) {
System.out.println( "error : " + e.getMessage() );
} finally {
if ( rs != null ) try { rs.close(); } catch ( SQLException e ) {}
if ( pstmt != null ) try { pstmt.close(); } catch ( SQLException e ) {}
if ( conn != null ) try { conn.close(); } catch ( SQLException e ) {}
}
return lists;
}
//paging list
public BoardListTO boardList( BoardListTO listTO) {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
// 페이지를 위한 기본 요소
int cpage = listTO.getCpage();
int recordPerPage = listTO.getRecordPerPage();
int BlockPerPage = listTO.getBlockPerPage();
try {
conn = dataSource.getConnection();
String sql = "select emot, seq, subject, writer, date_format(wdate, '%Y-%m-%d') wdate, hit, datediff(now(), wdate) wgap from board2 order by seq desc";
pstmt = conn.prepareStatement( sql, ResultSet.TYPE_SCROLL_INSENSITIVE, ResultSet.CONCUR_READ_ONLY );
rs = pstmt.executeQuery();
rs.last();
listTO.setTotalRecord( rs.getRow() );
rs.beforeFirst();
listTO.setTotalPage( ( (listTO.getTotalRecord() - 1) / recordPerPage ) + 1 );
int skip = (cpage -1) * recordPerPage;
if (skip != 0) rs.absolute( skip );
ArrayList<BoardTO> lists = new ArrayList<BoardTO>();
for( int i=0; i<recordPerPage && rs.next(); i++) {
BoardTO to = new BoardTO();
String emot = rs.getString( "emot" );
String seq = rs.getString( "seq" );
String subject = rs.getString( "subject" );
String writer = rs.getString( "writer" );
String wdate = rs.getString( "wdate" );
String hit = rs.getString( "hit" );
int wgap = rs.getInt( "wgap" );
to.setEmot(emot);
to.setSeq(seq);
to.setSubject(subject);
to.setWriter(writer);
to.setWdate(wdate);
to.setHit(hit);
to.setWgap(wgap);
lists.add( to );
}
listTO.setBoardLists( lists );
listTO.setStartBlock( ( (cpage-1)/BlockPerPage ) * BlockPerPage + 1 );
listTO.setEndBlock( ( (cpage-1)/BlockPerPage ) * BlockPerPage + BlockPerPage );
if ( listTO.getEndBlock() >= listTO.getTotalPage() ) {
listTO.setEndBlock( listTO.getTotalPage() );
}
} catch (SQLException e) {
System.out.println( "error : " + e.getMessage() );
} finally {
if ( rs != null ) try { rs.close(); } catch ( SQLException e ) {}
if ( pstmt != null ) try { pstmt.close(); } catch ( SQLException e ) {}
if ( conn != null ) try { conn.close(); } catch ( SQLException e ) {}
}
return listTO;
}
//view
public BoardTO boardView( BoardTO to ) {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
conn = dataSource.getConnection();
//조회수 증가시키기
String sql = "update board2 set hit = hit+1 where seq = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setString( 1, to.getSeq() );
rs = pstmt.executeQuery();
//데이터베이스에서 해당 글 내용 가져오기
sql = "select subject, writer, mail, content, hit, wip, wdate, emot from board2 where seq = ?";
pstmt = conn.prepareStatement( sql );
pstmt.setString( 1, to.getSeq() );
rs = pstmt.executeQuery();
//데이터베이스에서 sql실행문의 각 컬럼을 가져와서 변수에 저장
if ( rs.next() ) {
String subject = rs.getString( "subject" );
String writer = rs.getString( "writer" );
String mail = rs.getString( "mail" );
String content = rs.getString( "content" );
String hit = rs.getString( "hit" );
String wip = rs.getString( "wip" );
String wdate = rs.getString( "wdate" );
String emot = rs.getString( "emot" );
to.setSubject(subject);
to.setWriter(writer);
to.setMail(mail);
to.setContent(content);
to.setHit(hit);
to.setWip(wip);
to.setWdate(wdate);
to.setEmot(emot);
}
} catch (SQLException e) {
System.out.println( "error : " + e.getMessage() );
} finally {
if ( rs != null ) try { rs.close(); } catch ( SQLException e ) {}
if ( pstmt != null ) try { pstmt.close(); } catch ( SQLException e ) {}
if ( conn != null ) try { conn.close(); } catch ( SQLException e ) {}
}
return to;
}
//delete
public BoardTO boardDelete( BoardTO to ) {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
conn = dataSource.getConnection();
//데이터베이스에서 해당 글 내용 가져오기
String sql = "select subject, writer from board2 where seq = ?";
pstmt = conn.prepareStatement( sql );
pstmt.setString( 1, to.getSeq() );
rs = pstmt.executeQuery();
//데이터베이스에서 sql실행문의 각 컬럼을 가져와서 변수에 저장
if ( rs.next() ) {
String subject = rs.getString( "subject" );
String writer = rs.getString( "writer" );
to.setSubject(subject);
to.setWriter(writer);
}
} catch (SQLException e) {
System.out.println( "error : " + e.getMessage() );
} finally {
if ( rs != null ) try { rs.close(); } catch ( SQLException e ) {}
if ( pstmt != null ) try { pstmt.close(); } catch ( SQLException e ) {}
if ( conn != null ) try { conn.close(); } catch ( SQLException e ) {}
}
return to;
}
//delete_ok
public int boardDeleteOk( BoardTO to ) {
Connection conn = null;
PreparedStatement pstmt = null;
int flag = 2;
try {
conn = dataSource.getConnection();
//데이터베이스에서 해당 글 내용 가져오기
String sql = "delete from board2 where seq = ? and password = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setString( 1, to.getSeq() );
pstmt.setString( 2, to.getPassword() );
int result = pstmt.executeUpdate();
if ( result == 0 ) {
flag = 1;
} else if ( result ==1 ) {
flag = 0;
}
} catch (SQLException e) {
System.out.println( "error : " + e.getMessage() );
} finally {
if ( pstmt != null ) try { pstmt.close(); } catch ( SQLException e ) {}
if ( conn != null ) try { conn.close(); } catch ( SQLException e ) {}
}
return flag;
}
//modify
public BoardTO boardModify( BoardTO to ) {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
conn = dataSource.getConnection();
//데이터베이스에서 해당 글 내용 가져오기
String sql = "select writer, subject, content, mail, emot from board2 where seq = ?";
pstmt = conn.prepareStatement( sql );
pstmt.setString( 1, to.getSeq() );
rs = pstmt.executeQuery();
//데이터베이스에서 sql실행문의 각 컬럼을 가져와서 변수에 저장
if ( rs.next() ) {
String subject = rs.getString( "subject" );
String writer = rs.getString( "writer" );
String content = rs.getString( "content" );
String mail = rs.getString( "mail" );
String emot = rs.getString( "emot" );
to.setSubject(subject);
to.setWriter(writer);
to.setContent(content);
to.setMail(mail);
to.setEmot(emot);
}
} catch (SQLException e) {
System.out.println( "error : " + e.getMessage() );
} finally {
if ( rs != null ) try { rs.close(); } catch ( SQLException e ) {}
if ( pstmt != null ) try { pstmt.close(); } catch ( SQLException e ) {}
if ( conn != null ) try { conn.close(); } catch ( SQLException e ) {}
}
return to;
}
//modify_ok
public int boardModifyOk( BoardTO to ) {
Connection conn = null;
PreparedStatement pstmt = null;
int flag = 2;
try {
conn = dataSource.getConnection();
//데이터베이스에서 해당 글 내용 가져오기
String sql = "update board2 set subject = ?, content = ?, mail = ?, emot = ? where seq = ? and password = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setString( 1, to.getSubject() );
pstmt.setString( 2, to.getContent() );
pstmt.setString( 3, to.getMail() );
pstmt.setString( 4, to.getEmot() );
pstmt.setString( 5, to.getSeq() );
pstmt.setString( 6, to.getPassword() );
int result = pstmt.executeUpdate();
if ( result == 0 ) {
flag = 1;
} else if ( result ==1 ) {
flag = 0;
}
} catch (SQLException e) {
System.out.println( "error : " + e.getMessage() );
} finally {
if ( pstmt != null ) try { pstmt.close(); } catch ( SQLException e ) {}
if ( conn != null ) try { conn.close(); } catch ( SQLException e ) {}
}
return flag;
}
}
model2 패키지
-BoardAction.java (인터페이스)
package model2;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public interface BoardAction {
public void execute( HttpServletRequest request, HttpServletResponse response );
}
-ListAction.java
package model2;
import java.util.ArrayList;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import model1.BoardDAO;
import model1.BoardListTO;
import model1.BoardTO;
public class ListAction implements BoardAction {
@Override
public void execute(HttpServletRequest request, HttpServletResponse response) {
System.out.println( "ListAction 호출" );
int cpage = 1;
if ( request.getParameter( "cpage" ) != null && !request.getParameter( "cpage" ).equals("") ) {
cpage = Integer.parseInt( request.getParameter( "cpage" ) );
}
request.setAttribute( "cpage", cpage );
BoardListTO listTO = new BoardListTO();
listTO.setCpage(cpage);
BoardDAO dao = new BoardDAO();
listTO = dao.boardList(listTO);
request.setAttribute( "listTO", listTO );
ArrayList<BoardTO> lists = listTO.getBoardLists();
request.setAttribute( "lists", lists );
}
}
-WriteAction.java
package model2;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class WriteAction implements BoardAction {
@Override
public void execute(HttpServletRequest request, HttpServletResponse response) {
System.out.println( "WriteAction 호출" );
}
}
-WriteOkAction.java
package model2;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import model1.BoardDAO;
import model1.BoardTO;
public class WriteOkAction implements BoardAction {
@Override
public void execute(HttpServletRequest request, HttpServletResponse response) {
System.out.println( "WriteOkAction 호출" );
BoardTO to = new BoardTO();
to.setWriter( request.getParameter( "writer" ) );
to.setSubject( request.getParameter( "subject" ) );
to.setPassword( request.getParameter( "password" ) );
to.setContent( request.getParameter( "content" ) );
to.setWip( request.getRemoteAddr() );
to.setMail( "" );
if ( !request.getParameter( "mail1" ).equals("") && !request.getParameter( "mail2" ).equals("") ) {
to.setMail( request.getParameter( "mail1" ) + "@" + request.getParameter( "mail2" ) );
}
to.setEmot( request.getParameter( "emot" ) );
BoardDAO dao = new BoardDAO();
int flag = dao.boardWriteOk( to );
request.setAttribute( "flag", flag );
}
}
-ViewAction.java
package model2;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import model1.BoardDAO;
import model1.BoardTO;
public class ViewAction implements BoardAction {
@Override
public void execute(HttpServletRequest request, HttpServletResponse response) {
System.out.println( "ViewAction 호출" );
String seq = request.getParameter( "seq" );
BoardTO to = new BoardTO();
to.setSeq( seq );
BoardDAO dao = new BoardDAO();
to = dao.boardView( to );
request.setAttribute( "to", to );
}
}
-DeleteAction.java
package model2;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import model1.BoardDAO;
import model1.BoardTO;
public class DeleteAction implements BoardAction {
@Override
public void execute(HttpServletRequest request, HttpServletResponse response) {
System.out.println( "DeleteAction 호출" );
String seq = request.getParameter( "seq" );
BoardTO to = new BoardTO();
to.setSeq(seq);
BoardDAO dao = new BoardDAO();
to = dao.boardDelete(to);
request.setAttribute( "to", to );
}
}
-DeleteOkAction.java
package model2;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import model1.BoardDAO;
import model1.BoardTO;
public class DeleteOkAction implements BoardAction {
@Override
public void execute(HttpServletRequest request, HttpServletResponse response) {
System.out.println( "DeleteOkAction 호출" );
String seq = request.getParameter( "seq" );
String password = request.getParameter( "password" );
BoardTO to = new BoardTO();
to.setSeq(seq);
to.setPassword(password);
BoardDAO dao = new BoardDAO();
int flag = dao.boardDeleteOk(to);
request.setAttribute( "flag", flag );
}
}
-ModifyAction.java
package model2;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import model1.BoardDAO;
import model1.BoardTO;
public class ModifyAction implements BoardAction {
@Override
public void execute(HttpServletRequest request, HttpServletResponse response) {
System.out.println( "ModifyAction 호출" );
String seq = request.getParameter( "seq" );
BoardTO to = new BoardTO();
to.setSeq(seq);
BoardDAO dao = new BoardDAO();
to = dao.boardModify(to);
request.setAttribute( "to", to );
}
}
-ModifyOkAction.java
package model2;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import model1.BoardDAO;
import model1.BoardTO;
public class ModifyOkAction implements BoardAction {
@Override
public void execute(HttpServletRequest request, HttpServletResponse response) {
System.out.println( "ModifyOkAction 호출" );
String seq = request.getParameter( "seq" );
String password = request.getParameter( "password" );
String subject = request.getParameter( "subject" );
String content = request.getParameter( "content" );
String mail = "";
if ( !request.getParameter( "mail1" ).equals("") && !request.getParameter( "mail2" ).equals("") ) {
mail = request.getParameter( "mail1" ).equals("") + "@" + request.getParameter( "mail2" ).equals("");
}
String emot = request.getParameter( "emot1" );
BoardTO to = new BoardTO();
to.setSeq(seq);
to.setPassword(password);
to.setSubject(subject);
to.setContent(content);
to.setMail(mail);
to.setEmot(emot);
BoardDAO dao = new BoardDAO();
int flag = dao.boardModifyOk(to);
request.setAttribute( "flag", flag );
request.setAttribute( "seq", to.getSeq() );
}
}
servlet 패키지
-controllerEx01.java
package servlet;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import model2.BoardAction;
import model2.DeleteAction;
import model2.DeleteOkAction;
import model2.ListAction;
import model2.ModifyAction;
import model2.ModifyOkAction;
import model2.ViewAction;
import model2.WriteAction;
import model2.WriteOkAction;
@WebServlet("*.do")
public class controllerEx01 extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
this.doProcess(request, response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
this.doProcess(request, response);
}
protected void doProcess(HttpServletRequest request, HttpServletResponse response) {
try {
request.setCharacterEncoding( "utf-8" );
String action = request.getRequestURI().replaceAll( request.getContextPath(), "" );
String url = "";
BoardAction boardAction = null;
if ( action.equals( "/*.do" ) || action.equals( "/list.do" ) ) {
boardAction = new ListAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_list1.jsp";
} else if ( action.equals( "/view.do" ) ) {
boardAction = new ViewAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_view1.jsp";
} else if ( action.equals( "/write.do" ) ) {
boardAction = new WriteAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_write1.jsp";
} else if ( action.equals( "/write_ok.do" ) ) {
boardAction = new WriteOkAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_write1_ok.jsp";
} else if ( action.equals( "/delete.do" ) ) {
boardAction = new DeleteAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_delete1.jsp";
} else if ( action.equals( "/delete_ok.do" ) ) {
boardAction = new DeleteOkAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_delete1_ok.jsp";
} else if ( action.equals( "/modify.do" ) ) {
boardAction = new ModifyAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_modify1.jsp";
} else if ( action.equals( "/modify_ok.do" ) ) {
boardAction = new ModifyOkAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_modify1_ok.jsp";
}
RequestDispatcher dispatcher = request.getRequestDispatcher( url );
dispatcher.forward(request, response);
} catch (UnsupportedEncodingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (ServletException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
views폴더의 jsp파일들
-Board_list1.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="model1.BoardListTO" %>
<%@ page import="model1.BoardTO" %>
<%@ page import="model1.BoardDAO" %>
<%@ page import="java.util.ArrayList" %>
<%
request.setCharacterEncoding( "utf-8" );
int cpage = (Integer)request.getAttribute( "cpage" );
BoardListTO listTO = (BoardListTO)request.getAttribute( "listTO" );
int recordPerPage = listTO.getRecordPerPage();
int totalRecord = listTO.getTotalRecord();
int totalPage = listTO.getTotalPage();
int blockPerPage = listTO.getBlockPerPage();
int blockRecord = listTO.getBlockRecord();
int startBlock = listTO.getStartBlock();
int endBlock = listTO.getEndBlock();
ArrayList<BoardTO> lists = (ArrayList)request.getAttribute( "lists" );
StringBuffer sbHtml = new StringBuffer();
for ( BoardTO to : lists ) {
blockRecord++;
String emot = to.getEmot();
String seq = to.getSeq();
String subject = to.getSubject();
String writer = to.getWriter();
String wdate = to.getWdate();
String hit = to.getHit();
int wgap = to.getWgap();
sbHtml.append(" <tr> ");
sbHtml.append(" <td><img src='./images/emoticon/" + emot + ".png' width='15'/></td> ");
sbHtml.append(" <td>" + seq + "</td> ");
sbHtml.append(" <td class='left'><a href='view.do?cpage=" + cpage + "&seq=" + seq + "'>" + subject + "</a>  ");
if ( wgap == 0 ) {
sbHtml.append(" <img src='./images/icon_hot.gif' alt='HOT'></td> ");
}
sbHtml.append(" </td> ");
sbHtml.append(" <td>" + writer + "</td> ");
sbHtml.append(" <td>" + wdate + "</td> ");
sbHtml.append(" <td>" + hit + "</td> ");
sbHtml.append(" <td> </td> ");
sbHtml.append(" </tr> ");
}
%>
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0,minimum-scale=1.0,maximum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<title>Insert title here</title>
<link rel="stylesheet" type="text/css" href="./css/board_list.css">
</head>
<body>
<!-- 상단 디자인 -->
<div class="con_title">
<h3>게시판</h3>
<p>HOME > 게시판 > <strong>게시판</strong></p>
</div>
<div class="con_txt">
<div class="contents_sub">
<div class="board_top">
<div class="bold">총 <span class="txt_orange"><%= blockRecord %></span>건</div>
</div>
<!--게시판-->
<div class="board">
<table>
<tr>
<th width="3%"> </th>
<th width="5%">번호</th>
<th>제목</th>
<th width="10%">글쓴이</th>
<th width="17%">등록일</th>
<th width="5%">조회</th>
<th width="3%"> </th>
</tr>
<%= sbHtml %>
</table>
</div>
<div class="align_right">
<input type="button" value="쓰기" class="btn_write btn_txt01" style="cursor: pointer;" onclick="location.href='write.do?cpage=<%=cpage %>'" />
</div>
<!--//게시판-->
</div>
</div>
<!--//하단 디자인 -->
<!--페이지넘버-->
<div class="paginate_regular">
<div align="absmiddle">
<%
if ( startBlock == 1 ) {
out.println(" <span><a><<</a></span> ");
} else {
out.println(" <span><a href='list.do?cpage="+ (startBlock-blockPerPage) +"'><<</a></span> ");
}
out.println(" ");
if ( cpage == 1 ) {
out.println(" <span><a><</a></span> ");
} else {
out.println(" <span><a href='list.do?cpage="+ (cpage-1) +"'><</a></span> ");
}
out.println(" ");
for ( int i=startBlock; i<=endBlock; i++ ) {
if ( cpage == i ) {
out.println(" <span>[" + i + "]</span> ");
} else {
out.println(" <span><a href='list.do?cpage=" + i + "'>" + i + "</a></span> ");
}
}
out.println(" ");
if ( cpage == totalPage ) {
out.println(" <span><a>></a></span> ");
} else {
out.println(" <span><a href='list.do?cpage="+ (cpage+1) +"'>></a></span> ");
}
out.println(" ");
if ( endBlock == totalPage ) {
out.println(" <span><a>>></a></span> ");
} else {
out.println(" <span><a href='list.do?cpage="+ (startBlock+blockPerPage) +"'>>></a></span> ");
}
out.println(" ");
%>
</div>
</div>
<!--//페이지넘버-->
</body>
</html>
-Board_write1.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
request.setCharacterEncoding( "utf-8" );
String cpage = request.getParameter( "cpage" );
%>
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0,minimum-scale=1.0,maximum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<title>Insert title here</title>
<link rel="stylesheet" type="text/css" href="./css/board_write.css">
<script type="text/javascript">
window.onload = function() {
document.getElementById( 'submit1' ).onclick = function() {
if ( document.wfrm.info.checked == false ) {
alert( '개인정보 동의를 해주세요' );
return false;
}
if ( document.wfrm.writer.value.trim() == '' ) {
alert( '글쓴이를 입력해주세요' );
return false;
}
if ( document.wfrm.subject.value.trim() == '' ) {
alert( '제목을 입력해주세요' );
return false;
}
if ( document.wfrm.password.value.trim() == '' ) {
alert( '비밀번호를 입력해주세요' );
return false;
}
document.wfrm.submit();
}
}
</script>
</head>
<body>
<!-- 상단 디자인 -->
<div class="con_title">
<h3>게시판</h3>
<p>HOME > 게시판 > <strong>게시판</strong></p>
</div>
<div class="con_txt">
<form action="write_ok.do" method="post" name="wfrm">
<div class="contents_sub">
<!--게시판-->
<div class="board_write">
<table>
<tr>
<th class="top">글쓴이</th>
<td class="top" colspan="3"><input type="text" name="writer" value="" class="board_view_input_mail" maxlength="5" /></td>
</tr>
<tr>
<th>제목</th>
<td colspan="3"><input type="text" name="subject" value="" class="board_view_input" /></td>
</tr>
<tr>
<th>비밀번호</th>
<td colspan="3"><input type="password" name="password" value="" class="board_view_input_mail"/></td>
</tr>
<tr>
<th>내용</th>
<td colspan="3"><textarea name="content" class="board_editor_area"></textarea></td>
</tr>
<tr>
<th>이메일</th>
<td colspan="3"><input type="text" name="mail1" value="" class="board_view_input_mail"/> @ <input type="text" name="mail2" value="" class="board_view_input_mail"/></td>
</tr>
<tr>
<th>이모티콘</th>
<td colspan="3" align="center">
<table>
<tr>
<td>
<img src="./images/emoticon/emot01.png" width="25"/><br />
<input type="radio" name="emot" value="emot01" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot02.png" width="25" /><br />
<input type="radio" name="emot" value="emot02" class="input_radio" checked="checked"/>
</td>
<td>
<img src="./images/emoticon/emot03.png" width="25" /><br />
<input type="radio" name="emot" value="emot03" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot04.png" width="25" /><br />
<input type="radio" name="emot" value="emot04" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot05.png" width="25" /><br />
<input type="radio" name="emot" value="emot05" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot06.png" width="25" /><br />
<input type="radio" name="emot" value="emot06" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot07.png" width="25" /><br />
<input type="radio" name="emot" value="emot07" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot08.png" width="25" /><br />
<input type="radio" name="emot" value="emot08" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot09.png" width="25" /><br />
<input type="radio" name="emot" value="emot09" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot10.png" width="25" /><br />
<input type="radio" name="emot" value="emot10" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot11.png" width="25"/><br />
<input type="radio" name="emot" value="emot11" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot12.png" width="25" /><br />
<input type="radio" name="emot" value="emot12" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot13.png" width="25" /><br />
<input type="radio" name="emot" value="emot13" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot14.png" width="25" /><br />
<input type="radio" name="emot" value="emot14" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot15.png" width="25" /><br />
<input type="radio" name="emot" value="emot15" class="input_radio" />
</td>
</tr>
<tr>
<td>
<img src="./images/emoticon/emot16.png" width="25"/><br />
<input type="radio" name="emot" value="emot16" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot17.png" width="25" /><br />
<input type="radio" name="emot" value="emot17" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot18.png" width="25" /><br />
<input type="radio" name="emot" value="emot18" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot19.png" width="25" /><br />
<input type="radio" name="emot" value="emot19" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot20.png" width="25" /><br />
<input type="radio" name="emot" value="emot20" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot21.png" width="25" /><br />
<input type="radio" name="emot" value="emot21" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot22.png" width="25" /><br />
<input type="radio" name="emot" value="emot22" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot23.png" width="25" /><br />
<input type="radio" name="emot" value="emot23" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot24.png" width="25" /><br />
<input type="radio" name="emot" value="emot24" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot25.png" width="25"/><br />
<input type="radio" name="emot" value="emot25" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot26.png" width="25" /><br />
<input type="radio" name="emot" value="emot26" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot27.png" width="25" /><br />
<input type="radio" name="emot" value="emot27" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot28.png" width="25" /><br />
<input type="radio" name="emot" value="emot28" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot29.png" width="25" /><br />
<input type="radio" name="emot" value="emot29" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot30.png" width="25" /><br />
<input type="radio" name="emot" value="emot30" class="input_radio" />
</td>
</tr>
<tr>
<td>
<img src="./images/emoticon/emot31.png" width="25"/><br />
<input type="radio" name="emot" value="emot31" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot32.png" width="25" /><br />
<input type="radio" name="emot" value="emot32" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot33.png" width="25" /><br />
<input type="radio" name="emot" value="emot33" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot34.png" width="25" /><br />
<input type="radio" name="emot" value="emot34" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot35.png" width="25" /><br />
<input type="radio" name="emot" value="emot35" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot36.png" width="25" /><br />
<input type="radio" name="emot" value="emot36" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot37.png" width="25" /><br />
<input type="radio" name="emot" value="emot37" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot38.png" width="25" /><br />
<input type="radio" name="emot" value="emot38" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot39.png" width="25" /><br />
<input type="radio" name="emot" value="emot39" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot40.png" width="25"/><br />
<input type="radio" name="emot" value="emot40" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot41.png" width="25" /><br />
<input type="radio" name="emot" value="emot41" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot42.png" width="25" /><br />
<input type="radio" name="emot" value="emot42" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot43.png" width="25" /><br />
<input type="radio" name="emot" value="emot43" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot44.png" width="25" /><br />
<input type="radio" name="emot" value="emot44" class="input_radio" />
</td>
<td>
<img src="./images/emoticon/emot45.png" width="25" /><br />
<input type="radio" name="emot" value="emot45" class="input_radio" />
</td>
</tr>
</table>
</td>
</tr>
</table>
<table>
<tr>
<br />
<td style="text-align:left;border:1px solid #e0e0e0;background-color:f9f9f9;padding:5px">
<div style="padding-top:7px;padding-bottom:5px;font-weight:bold;padding-left:7px;font-family: Gulim,Tahoma,verdana;">※ 개인정보 수집 및 이용에 관한 안내</div>
<div style="padding-left:10px;">
<div style="width:97%;height:95px;font-size:11px;letter-spacing: -0.1em;border:1px solid #c5c5c5;background-color:#fff;padding-left:14px;padding-top:7px;">
1. 수집 개인정보 항목 : 회사명, 담당자명, 메일 주소, 전화번호, 홈페이지 주소, 팩스번호, 주소 <br />
2. 개인정보의 수집 및 이용목적 : 제휴신청에 따른 본인확인 및 원활한 의사소통 경로 확보 <br />
3. 개인정보의 이용기간 : 모든 검토가 완료된 후 3개월간 이용자의 조회를 위하여 보관하며, 이후 해당정보를 지체 없이 파기합니다. <br />
4. 그 밖의 사항은 개인정보취급방침을 준수합니다.
</div>
</div>
<div style="padding-top:7px;padding-left:5px;padding-bottom:7px;font-family: Gulim,Tahoma,verdana;">
<input type="checkbox" name="info" value="1" class="input_radio"> 개인정보 수집 및 이용에 대해 동의합니다.
</div>
</td>
</tr>
</table>
</div>
<div class="btn_area">
<div class="align_left">
<input type="button" value="목록" class="btn_list btn_txt02" style="cursor: pointer;" onclick="location.href='list.do?cpage=<%=cpage %>'" />
</div>
<div class="align_right">
<input type="button" id="submit1" value="쓰기" class="btn_write btn_txt01" style="cursor: pointer;" />
</div>
</div>
<!--//게시판-->
</div>
</form>
</div>
<!-- 하단 디자인 -->
</body>
</html>
-Board_write1_ok.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="model1.BoardTO" %>
<%@page import="model1.BoardDAO"%>
<%@ page import="javax.naming.Context" %>
<%@ page import="javax.naming.InitialContext" %>
<%@ page import="javax.naming.NamingException" %>
<%@ page import="javax.sql.DataSource" %>
<%@ page import="java.sql.Connection" %>
<%@ page import="java.sql.PreparedStatement" %>
<%@ page import="java.sql.SQLException" %>
<%
request.setCharacterEncoding( "utf-8" );
int flag = (Integer)request.getAttribute( "flag" );
out.println(" <script type='text/javascript'> ");
if ( flag == 0 ) {
out.println(" alert( '글쓰기에 성공했습니다.' ); ");
out.println(" location.href='./list.do' ");
} else {
out.println(" alert( '글쓰기에 실패했습니다.' ); ");
out.println(" history.back(); ");
}
out.println(" </script> ");
%>
-Board_view1.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="model1.BoardTO" %>
<%@ page import="javax.naming.Context" %>
<%@ page import="javax.naming.InitialContext" %>
<%@ page import="javax.naming.NamingException" %>
<%@ page import="javax.sql.DataSource" %>
<%@ page import="java.sql.Connection" %>
<%@ page import="java.sql.PreparedStatement" %>
<%@ page import="java.sql.ResultSet" %>
<%@ page import="java.sql.SQLException" %>
<%
request.setCharacterEncoding( "utf-8" );
String cpage = request.getParameter( "cpage" );
BoardTO to = (BoardTO)request.getAttribute( "to" );
String seq = to.getSeq();
String subject = to.getSubject();
String writer = to.getWriter();
String mail = to.getMail();
String content = to.getContent();
String hit = to.getHit();
String wip = to.getWip();
String wdate = to.getWdate();
String emot = to.getEmot();
%>
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0,minimum-scale=1.0,maximum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<title>Insert title here</title>
<link rel="stylesheet" type="text/css" href="./css/board_view.css">
</head>
<body>
<!-- 상단 디자인 -->
<div class="con_title">
<h3>게시판</h3>
<p>HOME > 게시판 > <strong>게시판</strong></p>
</div>
<div class="con_txt">
<div class="contents_sub">
<!--게시판-->
<div class="board_view">
<table>
<tr>
<th width="10%">제목</th>
<td width="60%">(<img src="./images/emoticon/<%=emot %>.png" width="15"/>) <%=subject %></td>
<th width="10%">등록일</th>
<td width="20%"><%=wdate %></td>
</tr>
<tr>
<th>글쓴이</th>
<td><%=writer %>(<%=mail %>)(<%=wip %>)</td>
<th>조회</th>
<td><%=hit %></td>
</tr>
<tr>
<td colspan="4" height="200" valign="top" style="padding: 20px; line-height: 160%"><%= content %></td>
</tr>
</table>
</div>
<div class="btn_area">
<div class="align_left">
<input type="button" value="목록" class="btn_list btn_txt02" style="cursor: pointer;" onclick="location.href='./list.do?cpage=<%=cpage %>'" />
</div>
<div class="align_right">
<input type="button" value="수정" class="btn_list btn_txt02" style="cursor: pointer;" onclick="location.href='./modify.do?cpage=<%=cpage %>&seq=<%=seq %>'" />
<input type="button" value="삭제" class="btn_list btn_txt02" style="cursor: pointer;" onclick="location.href='./delete.do?cpage=<%=cpage %>&seq=<%=seq %>'" />
<input type="button" value="쓰기" class="btn_write btn_txt01" style="cursor: pointer;" onclick="location.href='./write.do'" />
</div>
</div>
<!--//게시판-->
</div>
</div>
<!-- 하단 디자인 -->
</body>
</html>
-Board_delete1.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="model1.BoardTO" %>
<%@ page import="javax.naming.Context" %>
<%@ page import="javax.naming.InitialContext" %>
<%@ page import="javax.naming.NamingException" %>
<%@ page import="javax.sql.DataSource" %>
<%@ page import="java.sql.Connection" %>
<%@ page import="java.sql.PreparedStatement" %>
<%@ page import="java.sql.ResultSet" %>
<%@ page import="java.sql.SQLException" %>
<%
request.setCharacterEncoding( "utf-8" );
String cpage = request.getParameter( "cpage" );
BoardTO to = (BoardTO)request.getAttribute( "to" );
String seq = to.getSeq();
String subject = to.getSubject();
String writer = to.getWriter();
%>
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0,minimum-scale=1.0,maximum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<title>Insert title here</title>
<link rel="stylesheet" type="text/css" href="./css/board_write.css">
<script type="text/javascript">
window.onload = function() {
document.getElementById('submit1').onclick = function() {
if ( document.dfrm.password.value.trim() == '' ) {
alert('비밀번호를 입력해주세요.');
return false;
}
document.dfrm.submit();
}
}
</script>
</head>
<body>
<!-- 상단 디자인 -->
<div class="con_title">
<h3>게시판</h3>
<p>HOME > 게시판 > <strong>게시판</strong></p>
</div>
<div class="con_txt">
<form action="./delete_ok.do" method="post" name="dfrm">
<input type="hidden" name="seq" value="<%=seq%>">
<input type="hidden" name="cpage" value="<%=cpage%>">
<div class="contents_sub">
<!--게시판-->
<div class="board_write">
<table>
<tr>
<th class="top">글쓴이</th>
<td class="top" colspan="3"><input type="text" name="writer" value="<%=writer %>" class="board_view_input_mail" maxlength="5" readonly/></td>
</tr>
<tr>
<th>제목</th>
<td colspan="3"><input type="text" name="subject" value="<%=subject %>" class="board_view_input" readonly/></td>
</tr>
<tr>
<th>비밀번호</th>
<td colspan="3"><input type="password" name="password" value="" class="board_view_input_mail"/></td>
</tr>
</table>
</div>
<div class="btn_area">
<div class="align_left">
<input type="button" value="목록" class="btn_list btn_txt02" style="cursor: pointer;" onclick="location.href='./list.do?cpage=<%=cpage %>'" />
<input type="button" value="보기" class="btn_list btn_txt02" style="cursor: pointer;" onclick="location.href='./view.do?seq=<%=seq %>&cpage=<%=cpage %>'" />
</div>
<div class="align_right">
<input type="button" id="submit1" value="삭제" class="btn_write btn_txt01" style="cursor: pointer;" />
</div>
</div>
<!--//게시판-->
</div>
</form>
</div>
<!-- 하단 디자인 -->
</body>
</html>
-Board_delete1_ok.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="javax.naming.Context" %>
<%@ page import="javax.naming.InitialContext" %>
<%@ page import="javax.naming.NamingException" %>
<%@ page import="javax.sql.DataSource" %>
<%@ page import="java.sql.Connection" %>
<%@ page import="java.sql.PreparedStatement" %>
<%@ page import="java.sql.SQLException" %>
<%
request.setCharacterEncoding( "utf-8" );
String cpage = request.getParameter( "cpage" );
int flag = (Integer)request.getAttribute( "flag" );
out.println(" <script type='text/javascript'> ");
if ( flag == 0 ) {
out.println(" alert( '글삭제에 성공했습니다.' ); ");
out.println(" location.href='./list.do?cpage="+cpage+"'");
} else if ( flag == 1 ) {
out.println(" alert( '비밀번호가 틀립니다.' ); ");
out.println(" history.back(); ");
} else {
out.println(" alert( '글삭제에 실패했습니다.' ); ");
out.println(" history.back(); ");
}
out.println(" </script> ");
%>
-Board_modify1.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="model1.BoardTO" %>
<%@ page import="javax.naming.Context" %>
<%@ page import="javax.naming.InitialContext" %>
<%@ page import="javax.naming.NamingException" %>
<%@ page import="javax.sql.DataSource" %>
<%@ page import="java.sql.Connection" %>
<%@ page import="java.sql.PreparedStatement" %>
<%@ page import="java.sql.ResultSet" %>
<%@ page import="java.sql.SQLException" %>
<%
request.setCharacterEncoding( "utf-8" );
String cpage = request.getParameter( "cpage" );
BoardTO to = (BoardTO)request.getAttribute( "to" );
String seq = to.getSeq();
String subject = to.getSubject();
String writer = to.getWriter();
String content = to.getContent();
String mail[] = to.getMail().split( "@" );
String emot = to.getEmot();
StringBuffer sbEmot = new StringBuffer();
for ( int row=1, i=1; row<=3; row++ ) {
sbEmot.append(" <tr> ");
for ( int col=1; col<=15; col++ ) {
sbEmot.append(" <td> ");
if ( i <= 9 ) {
if ( emot.equals( "emot0"+i ) ) {
sbEmot.append(" <img src='./images/emoticon/emot0" + i +".png' width='25'/> ");
sbEmot.append(" <input type='radio' name='emot1' value='emot0" + i + "' class='input_radio' checked='checked' /> ");
} else {
sbEmot.append(" <img src='./images/emoticon/emot0" + i +".png' width='25'/> ");
sbEmot.append(" <input type='radio' name='emot1' value='emot0" + i + "' class='input_radio' /> ");
}
} else {
if ( emot.equals( "emot"+i ) ) {
sbEmot.append(" <img src='./images/emoticon/emot" + i +".png' width='25'/> ");
sbEmot.append(" <input type='radio' name='emot1' value='emot" + i + "' class='input_radio' checked='checked' /> ");
} else {
sbEmot.append(" <img src='./images/emoticon/emot" + i +".png' width='25'/> ");
sbEmot.append(" <input type='radio' name='emot1' value='emot" + i + "' class='input_radio' /> ");
}
}
sbEmot.append(" </td> ");
i++;
}
sbEmot.append(" </tr> ");
}
%>
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0,minimum-scale=1.0,maximum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<title>Insert title here</title>
<link rel="stylesheet" type="text/css" href="./css/board_write.css">
<script type="text/javascript">
window.onload = function() {
document.getElementById('submit1').onclick = function() {
if ( document.mfrm.subject.value.trim() == '' ) {
alert( '제목을 입력해주세요.' );
return false;
}
if ( document.mfrm.password.value.trim() == '' ) {
alert( '비밀번호를 입력해주세요.' );
return false;
}
if ( document.mfrm.content.value.trim() == '' ) {
alert( '내용을 입력해주세요.' );
return false;
}
document.mfrm.submit();
}
}
</script>
</head>
<body>
<!-- 상단 디자인 -->
<div class="con_title">
<h3>게시판</h3>
<p>HOME > 게시판 > <strong>게시판</strong></p>
</div>
<div class="con_txt">
<form action="./modify_ok.do" method="post" name="mfrm">
<input type="hidden" name="seq" value="<%= seq%>">
<input type="hidden" name="cpage" value="<%=cpage%>">
<div class="contents_sub">
<!--게시판-->
<div class="board_write">
<table>
<tr>
<th class="top">글쓴이</th>
<td class="top" colspan="3"><input type="text" name="writer" value="<%=writer %>" class="board_view_input_mail" maxlength="5" readonly/></td>
</tr>
<tr>
<th>제목</th>
<td colspan="3"><input type="text" name="subject" value="<%=subject %>" class="board_view_input" /></td>
</tr>
<tr>
<th>비밀번호</th>
<td colspan="3"><input type="password" name="password" value="" class="board_view_input_mail"/></td>
</tr>
<tr>
<th>내용</th>
<td colspan="3"><textarea name="content" class="board_editor_area"><%=content %></textarea></td>
</tr>
<tr>
<th>이메일</th>
<td colspan="3"><input type="text" name="mail1" value="<%=mail[0] %>" class="board_view_input_mail"/> @ <input type="text" name="mail2" value="<%=mail[1] %>" class="board_view_input_mail"/></td>
</tr>
<tr>
<th>이모티콘</th>
<td colspan="3" align="center">
<table>
<%=sbEmot %>
</table>
</td>
</tr>
</table>
</div>
<div class="btn_area">
<div class="align_left">
<input type="button" value="목록" class="btn_list btn_txt02" style="cursor: pointer;" onclick="location.href='./list.do?cpage=<%=cpage %>'" />
<input type="button" value="보기" class="btn_list btn_txt02" style="cursor: pointer;" onclick="location.href='./view.do?cpage=<%=cpage %>&seq=<%=seq %>'" />
</div>
<div class="align_right">
<input type="button" id="submit1" value="수정" class="btn_write btn_txt01" style="cursor: pointer;" />
</div>
</div>
<!--//게시판-->
</div>
</form>
</div>
<!-- 하단 디자인 -->
</body>
</html>
-Board_modify1_ok.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="javax.naming.Context" %>
<%@ page import="javax.naming.InitialContext" %>
<%@ page import="javax.naming.NamingException" %>
<%@ page import="javax.sql.DataSource" %>
<%@ page import="java.sql.Connection" %>
<%@ page import="java.sql.PreparedStatement" %>
<%@ page import="java.sql.SQLException" %>
<%
request.setCharacterEncoding( "utf-8" );
String cpage = request.getParameter( "cpage" );
String seq = (String)request.getAttribute( "seq" );
int flag = (Integer)request.getAttribute( "flag" );
out.println(" <script type='text/javascript'> ");
if ( flag == 0 ) {
out.println(" alert( '글수정에 성공했습니다.' ); ");
out.println(" location.href='./view.do?cpage=" +cpage+ "&seq="+seq+"' ");
} else if ( flag == 1 ) {
out.println(" alert( '비밀번호가 틀립니다.' ); ");
out.println(" history.back(); ");
} else {
out.println(" alert( '글수정에 실패했습니다.' ); ");
out.println(" history.back(); ");
}
out.println(" </script> ");
%>
11. 표현 언어(Expression Language)
p250
JSP의 스크립트 요소를 보완하는 역할을 한다.
표현 언어는 JSP의 표현식보다 간결하고 편리하기 때문에 많이 사용된다.
JSP의 표현식 : <%= member.getAddress().getZipcode() %>
표현 언어 : ${ member.address.zipcode }
위와 같이 나타낸다.
-산술연산자 및 출력, 비교연산자
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8" isELIgnored="false"%>
<!-- isELIgnored="false"면 사용, isELIgnored="true"면 사용하지 않는다는 의미 -->
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<table border='1' width='500'>
<tr>
<td>표현식</td>
<td>값</td>
</tr>
<tr>
<td>표현식</td>
<td><%=2 %></td>
</tr>
<tr>
<td>표현 언어</td>
<td>${ "3" }</td><!-- 를 사용하고 출력하고자하는 문자는 ""안에 넣는다. -->
</tr>
<tr>
<td>표현 언어</td>
<td>\${ "2" }</td><!-- 를 사용하기 싫다면 슬래쉬\를 사용한다. -->
</tr>
<tr>
<td>표현 언어</td>
<td>${ 2 }</td><!-- 를 사용하여 숫자를 표현 -->
</tr>
<tr>
<td>\${ 2+5 }</td>
<td>${ 2+5 }</td>
</tr>
<tr>
<td>\${ 2 div 5 }</td>
<td>${ 2 div 5 }</td> <!-- 소수점으로 결과가 나온다. -->
</tr>
<tr>
<td>\${ 2 mod 5 }</td>
<td>${ 2 mod 5 }</td>
</tr>
<tr>
<td>\${ "10"+1 }</td>
<td>${ "10"+1 }</td> <!-- 연산자 중심이기때문에 양쪽 모두 정수가 된다. -->
</tr>
<tr>
<td>\${ "10"+"1" }</td>
<td>${ "10"+"1" }</td> <!-- 연산자 중심이기때문에 양쪽 모두 정수가 된다. -->
</tr>
<tr>
<td>\${ 2<3 }</td>
<td>${ 2<3 }</td> <!-- 연산자 중심이기때문에 양쪽 모두 정수가 된다. -->
</tr>
<tr>
<td>\${ 2 lt 3 }</td>
<td>${ 2 lt 3 }</td> <!-- 연산자 중심이기때문에 양쪽 모두 정수가 된다. -->
</tr>
<tr>
<td>\${ empty data }</td>
<td>${ empty data }</td> <!-- 연산자 중심이기때문에 양쪽 모두 정수가 된다. -->
</tr>
<tr>
<td>\${ (2<3) ? "작다":"크다" }</td>
<td>${ (2<3) ? "작다":"크다" }</td> <!-- 연산자 중심이기때문에 양쪽 모두 정수가 된다. -->
</tr>
</table>
</body>
</html>
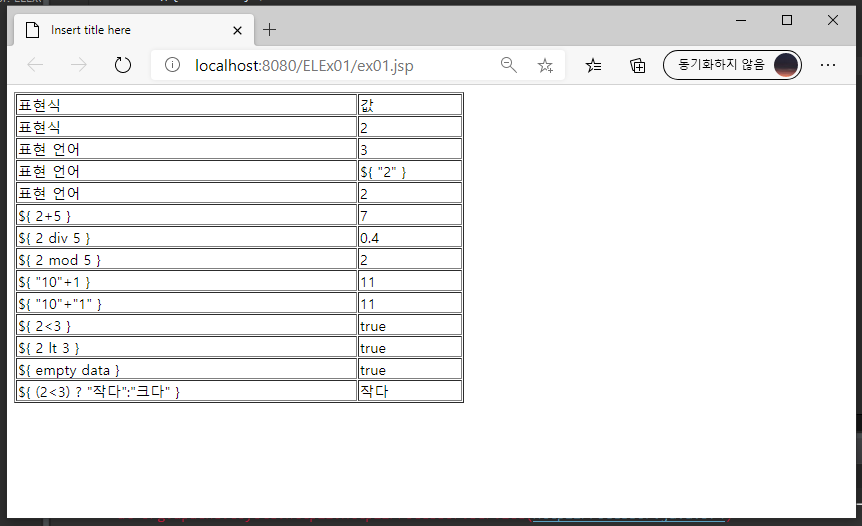
-같은 페이지에서 데이터 가져와서 출력하기
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
String name1 = "홍길동";
// pageContext, request, session, application을 사용하면 데이터에 접근할 수 있다.
pageContext.setAttribute( "name2", "박문수" );
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<%
out.println( name1 + "<br>" );
%>
<!-- 기존쓰던 방식 -->
name1 : <%= name1 %><br>
name2 : <%= pageContext.getAttribute("name2") %><br>
<!-- EL을 이용한 방식 -->
name2 : ${ name2 }<br>
name2 : ${ pageScope.name2 }<br>
name2 : ${ pageScope['name2'] }<br>
</body>
</html>
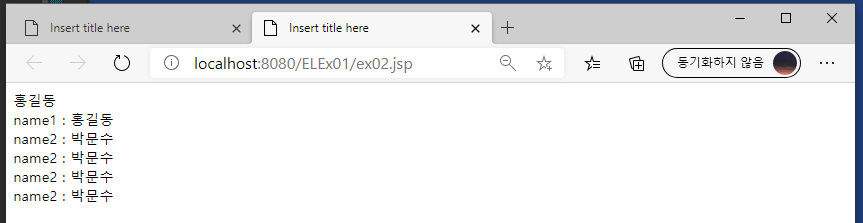
-같은 페이지에서 데이터 가져와서 출력하기(pageContext, request, session, application 사용)
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
pageContext.setAttribute( "name1", "홍길동" );
request.setAttribute( "name2", "박문수" );
session.setAttribute( "name3", "정몽주" );
application.setAttribute( "name4", "이몽룡" );
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
${ name1 }<br>
${ name2 }<br>
${ name3 }<br>
${ name4 }<br>
</body>
</html>
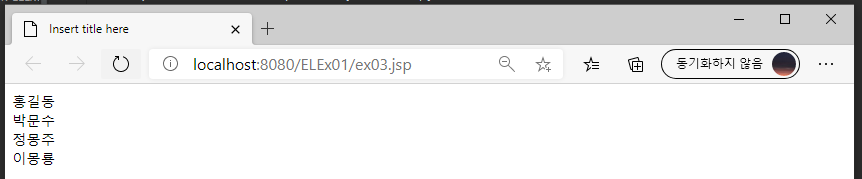
이름을 통일해서 사용하기 name으로 출력하면 가장가까운 데이터가 출력된다.(홍길동)
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
pageContext.setAttribute( "name1", "홍길동" );
request.setAttribute( "name2", "박문수" );
session.setAttribute( "name3", "정몽주" );
application.setAttribute( "name4", "이몽룡" );
pageContext.setAttribute( "name", "홍길동" );
request.setAttribute( "name", "박문수" );
session.setAttribute( "name", "정몽주" );
application.setAttribute( "name", "이몽룡" );
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
${ name1 }<br>
${ name2 }<br>
${ name3 }<br>
${ name4 }<br>
<hr>
${ name }<br>
</body>
</html>
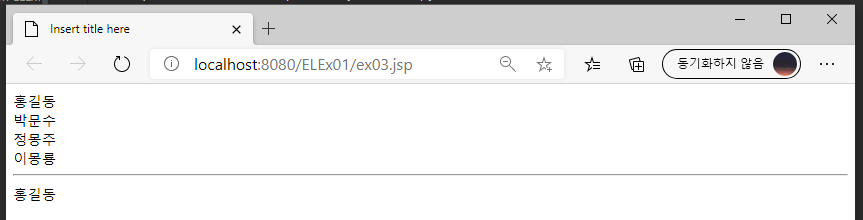
이름은 통일되어있지만 각각의 데이터를 받아와서 출력해보자.
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
pageContext.setAttribute( "name1", "홍길동" );
request.setAttribute( "name2", "박문수" );
session.setAttribute( "name3", "정몽주" );
application.setAttribute( "name4", "이몽룡" );
pageContext.setAttribute( "name", "홍길동" );
request.setAttribute( "name", "박문수" );
session.setAttribute( "name", "정몽주" );
application.setAttribute( "name", "이몽룡" );
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
${ name1 }<br>
${ name2 }<br>
${ name3 }<br>
${ name4 }<br>
<hr>
${ name }<br>
<hr>
${ pageScope.name }<br>
${ requestScope.name }<br>
${ sessionScope.name }<br>
${ applicationScope.name }<br>
</body>
</html>
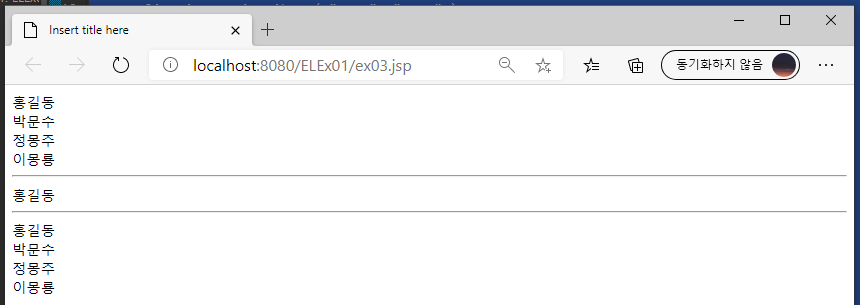
-jsp 및 브라우저 정보 받아오기
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="java.util.Enumeration" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<%
Enumeration e = request.getHeaderNames();
while ( e.hasMoreElements() ) {
String name = (String)e.nextElement();
String value = request.getHeader(name);
out.println(name + "/" + value );
}
%>
<br><br><hr>
${ header['host'] }<br><br>
${ header['user-agent'] }<br><br>
${ header.host }<br><br>
header['user-agent'] 이거는 -와 같이 특수기호가 있어서 .(닷)을 사용할 수 없다.
</body>
</html>
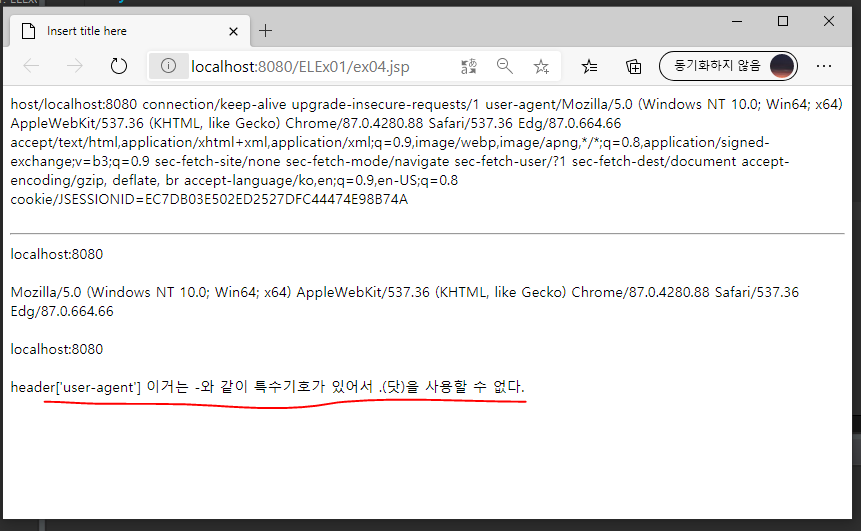
-다른jsp페이지에서 데이터 주고받기
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
request.setCharacterEncoding( "utf-8" );
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
-점사용<br>
아이디 : ${ param.id }<br>
비밀번호 : ${ param.password }<br>
${ paramValues.language[0] }<br>
${ paramValues.language[1] }<br>
${ paramValues.language[2] }<br>
<hr>
-대괄호사용<br>
아이디 : ${ param['id'] }<br>
비밀번호 : ${ param['password'] }<br>
</body>
</html>
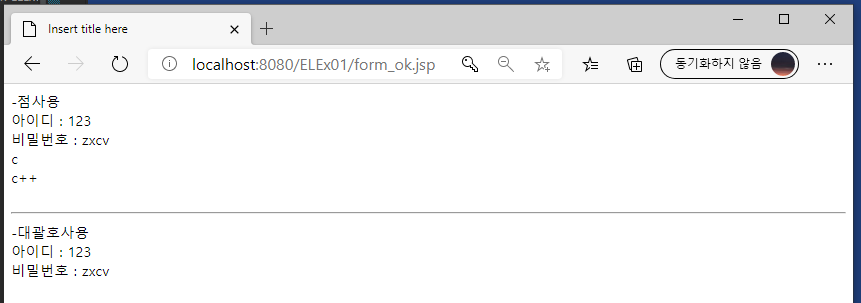
-TO를 만들고 같은 페이지에서 TO데이터를 받아와서 출력하기
BoardTO.java
package model1;
public class BoardTO {
private String subject;
private String writer;
public void setSubject(String subject) {
this.subject = subject;
}
public void setWriter(String writer) {
this.writer = writer;
}
public String getSubject() {
System.out.println( "getSubject() 호출" );
return subject;
}
public String getWriter() {
System.out.println( "getWriter() 호출" );
return writer;
}
}
ex05.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="model1.BoardTO" %>
<%
BoardTO to = new BoardTO();
to.setSubject( "제목1" );
to.setWriter( "작성자1" );
pageContext.setAttribute( "to", to );
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
제목 : ${ to.subject }<br>
작성자 : ${ to.writer }<br>
</body>
</html>

이번에는 여러 개의 to를 처리해보자.
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="model1.BoardTO" %>
<%
BoardTO to1 = new BoardTO();
to1.setSubject( "제목1" );
to1.setWriter( "작성자1" );
BoardTO to2 = new BoardTO();
to2.setSubject( "제목2" );
to2.setWriter( "작성자2" );
BoardTO[] lists = { to1, to2 };
//pageContext.setAttribute( "to", to );
request.setAttribute( "lists", lists );
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
제목 : ${ lists[0].subject }<br>
작성자 : ${ lists[0].writer }<br>
제목 : ${ lists[1].subject }<br>
작성자 : ${ lists[1].writer }<br>
</body>
</html>
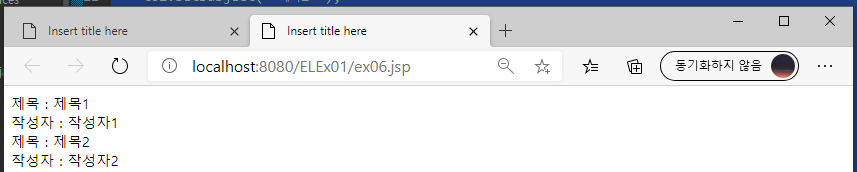
이때 특이한게 EL은 배열의 없는 인덱스를 가져와도 에러가 뜨지 않고 값이 공백으로 나온다.
이번에는 ArrayList에 데이터를 넣어서 가져와보자.
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="model1.BoardTO" %>
<%@ page import="java.util.ArrayList" %>
<%
BoardTO to1 = new BoardTO();
to1.setSubject( "제목1" );
to1.setWriter( "작성자1" );
BoardTO to2 = new BoardTO();
to2.setSubject( "제목2" );
to2.setWriter( "작성자2" );
ArrayList<BoardTO> lists = new ArrayList();
lists.add( to1 );
lists.add( to2 );
//pageContext.setAttribute( "to", to );
request.setAttribute( "lists", lists );
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
제목 : ${ lists[0].subject }<br>
작성자 : ${ lists[0].writer }<br>
제목 : ${ lists[1].subject }<br>
작성자 : ${ lists[1].writer }<br>
</body>
</html>
이제 응용해보면 게시판에 자바소스 없이 EL로만 표현이 가능하다.
이번에는 HashMap에 저장하고 출력해보자.
HashMap은 ArrayList와 다르게 순서가 없는 객체의 나열이므로 바로 키값을 사용해서 데이터를 불러온다.
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="model1.BoardTO" %>
<%@ page import="java.util.ArrayList" %>
<%@ page import="java.util.HashMap" %>
<%
BoardTO to1 = new BoardTO();
to1.setSubject( "제목1" );
to1.setWriter( "작성자1" );
BoardTO to2 = new BoardTO();
to2.setSubject( "제목2" );
to2.setWriter( "작성자2" );
HashMap<String, BoardTO> lists = new HashMap();
lists.put( "to1", to1 );
lists.put( "to2", to2 );
//pageContext.setAttribute( "to", to );
request.setAttribute( "lists", lists );
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
제목 : ${ lists.to1.subject }<br>
작성자 : ${ lists.to1.writer }<br>
제목 : ${ lists.to2.subject }<br>
작성자 : ${ lists.to2.writer }<br>
<hr>
-배열로 나타내기<br>
제목 : ${ lists['to2'].subject }<br>
작성자 : ${ lists['to2']['writer'] }<br>
</body>
</html>
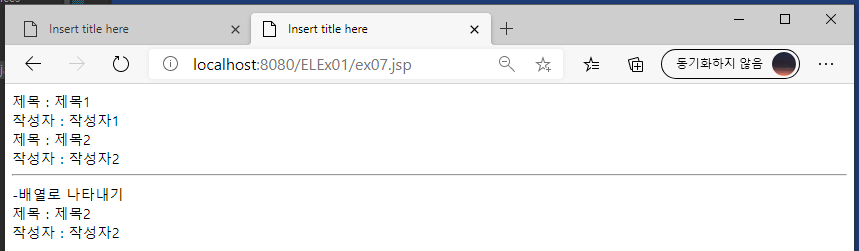
-이번에는 자바클래스에 숫자를 특정형태로 바꿔주는 패턴화 메서드를 만들고 EL을 이용해서 출력해보자.
FormatUtil.java
package util;
import java.text.DecimalFormat;
public class FormatUtil {
//데이터를 숫자로 받아서 패턴화하기(컴마를 찍는다든지 등등)
public static String changeFormat( long number, String pattern ) {
DecimalFormat format = new DecimalFormat( pattern );
return format.format( number );
}
}
ex08.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="util.FormatUtil" %>
<%
pageContext.setAttribute( "price", 12345L );
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
가격 : ${ price }<br>
가격 : ${ FormatUtil.changeFormat( price, '#,##0' ) }<br>
</body>
</html>

ㅇ자료실 게시판을 Model2로 만들기
model1 패키지
-BoardTO.java
package model1;
public class BoardTO {
private String seq;
private String subject;
private String writer;
private String mail;
private String password;
private String content;
private String filename;
private long filesize;
private String file;
private String hit;
private String wip;
private String wdate;
private int wgap;
public String getSeq() {
return seq;
}
public String getSubject() {
return subject;
}
public String getWriter() {
return writer;
}
public String getMail() {
return mail;
}
public String getPassword() {
return password;
}
public String getContent() {
return content;
}
public String getFilename() {
return filename;
}
public long getFilesize() {
return filesize;
}
public String getHit() {
return hit;
}
public String getWip() {
return wip;
}
public String getWdate() {
return wdate;
}
public int getWgap() {
return wgap;
}
public String getFile() {
return file;
}
public void setSeq(String seq) {
this.seq = seq;
}
public void setSubject(String subject) {
this.subject = subject;
}
public void setWriter(String writer) {
this.writer = writer;
}
public void setMail(String mail) {
this.mail = mail;
}
public void setPassword(String password) {
this.password = password;
}
public void setContent(String content) {
this.content = content;
}
public void setFilename(String filename) {
this.filename = filename;
}
public void setFilesize(long filesize) {
this.filesize = filesize;
}
public void setHit(String hit) {
this.hit = hit;
}
public void setWip(String wip) {
this.wip = wip;
}
public void setWdate(String wdate) {
this.wdate = wdate;
}
public void setWgap(int wgap) {
this.wgap = wgap;
}
public void setFile(String file) {
this.file = file;
}
}
-BoardDAO.java
package model1;
import java.io.File;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import javax.naming.Context;
import javax.naming.InitialContext;
import javax.sql.DataSource;
public class BoardDAO {
private DataSource dataSource;
public BoardDAO() {
try {
Context initCtx = new InitialContext();
Context envCtx = (Context)initCtx.lookup( "java:comp/env" );
this.dataSource = (DataSource)envCtx.lookup( "jdbc/mariadb2" );
} catch (Exception e) {
System.out.println( "[에러]: " + e.getMessage() );
}
}
//write
public void boardWrite() {}
//write_ok
public int boardWriteOk( BoardTO to ) {
Connection conn = null;
PreparedStatement pstmt = null;
int flag = 1;
try {
conn = dataSource.getConnection();
String sql = "insert into pds_board1 values (0, ?, ?, ?, ?, ?, ?, ?, 0, ?, now() )";
pstmt = conn.prepareStatement(sql);
pstmt.setString( 1, to.getSubject() );
pstmt.setString( 2, to.getWriter() );
pstmt.setString( 3, to.getMail() );
pstmt.setString( 4, to.getPassword() );
pstmt.setString( 5, to.getContent() );
pstmt.setString( 6, to.getFilename() );
pstmt.setLong( 7, to.getFilesize() );
pstmt.setString( 8, to.getWip() );
int result = pstmt.executeUpdate();
if ( result == 1 ) {
//정상일 때
flag = 0;
}
} catch(SQLException e) {
System.out.println( "error: " + e.getMessage() );
} finally {
if ( pstmt != null ) try { pstmt.close(); } catch ( SQLException e ) {}
if ( conn != null ) try { conn.close(); } catch ( SQLException e ) {}
}
return flag;
}
//list
public ArrayList<BoardTO> boardList() {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
ArrayList<BoardTO> lists = new ArrayList<BoardTO>();
try {
conn = dataSource.getConnection();
String sql = "select seq, subject, writer, filesize, date_format(wdate, '%Y-%m-%d') wdate, hit, datediff(now(), wdate) wgap from pds_board1 order by seq desc";
pstmt = conn.prepareStatement(sql, ResultSet.TYPE_SCROLL_INSENSITIVE, ResultSet.CONCUR_READ_ONLY);
rs = pstmt.executeQuery();
while( rs.next() ) {
BoardTO to = new BoardTO();
String seq = rs.getString( "seq" );
String subject = rs.getString( "subject" );
String writer = rs.getString( "writer" );
long filesize = rs.getLong( "filesize" );
String wdate = rs.getString( "wdate" );
String hit = rs.getString( "hit" );
int wgap = rs.getInt( "wgap" );
to.setSeq(seq);
to.setSubject(subject);
to.setWriter(writer);
to.setFilesize(filesize);
to.setWdate(wdate);
to.setHit(hit);
to.setWgap(wgap);
lists.add(to);
}
} catch(SQLException e) {
System.out.println( "error: " + e.getMessage() );
} finally {
if ( rs != null ) try { rs.close(); } catch ( SQLException e ) {}
if ( pstmt != null ) try { pstmt.close(); } catch ( SQLException e ) {}
if ( conn != null ) try { conn.close(); } catch ( SQLException e ) {}
}
return lists;
}
//view
public BoardTO boardView( BoardTO to ) {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
String file = "";
try {
conn = dataSource.getConnection();
String sql = "update pds_board1 set hit = hit+1 where seq = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setString( 1, to.getSeq() );
rs = pstmt.executeQuery();
sql = "select subject, writer, filename, filesize, mail, wip, wdate, hit, content from pds_board1 where seq = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setString( 1, to.getSeq() );
rs = pstmt.executeQuery();
//데이터를 하나만 가져오니깐 while문 대신 if문을 사용한다.
if ( rs.next() ) {
String subject = rs.getString( "subject" );
String writer = rs.getString( "writer" );
String mail = rs.getString( "mail" );
String wip = rs.getString( "wip" );
String wdate = rs.getString( "wdate" );
String hit = rs.getString( "hit" );
String filename = rs.getString( "filename" );
long filesize = rs.getLong( "filesize" );
if ( filesize != 0 ) {
file = "<a href='./upload/" + filename + "'>" + filename + "</a> (" + filesize + "byte)";
}
String content = rs.getString( "content" ).replaceAll( "\n", "<br>");
to.setSubject(subject);
to.setWriter(writer);
to.setMail(mail);
to.setWip(wip);
to.setWdate(wdate);
to.setHit(hit);
to.setContent(content);
to.setFilename(filename);
to.setFilesize(filesize);
to.setFile(file);
}
} catch(SQLException e) {
System.out.println( "error: " + e.getMessage() );
} finally {
if ( rs != null ) try { rs.close(); } catch ( SQLException e ) {}
if ( pstmt != null ) try { pstmt.close(); } catch ( SQLException e ) {}
if ( conn != null ) try { conn.close(); } catch ( SQLException e ) {}
}
return to;
}
//delete
public BoardTO boardDelete( BoardTO to ) {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
conn = dataSource.getConnection();
String sql = "select subject, writer from pds_board1 where seq = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setString( 1, to.getSeq() );
rs = pstmt.executeQuery();
//데이터를 하나만 가져오니깐 while문 대신 if문을 사용한다.
if ( rs.next() ) {
String subject = rs.getString( "subject" );
String writer = rs.getString( "writer" );
to.setSubject(subject);
to.setWriter(writer);
}
} catch(SQLException e) {
System.out.println( "error: " + e.getMessage() );
} finally {
if ( rs != null ) try { rs.close(); } catch ( SQLException e ) {}
if ( pstmt != null ) try { pstmt.close(); } catch ( SQLException e ) {}
if ( conn != null ) try { conn.close(); } catch ( SQLException e ) {}
}
return to;
}
//delete_ok
public int boardDeleteOk( BoardTO to ) {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
int flag = 2;
try {
conn = dataSource.getConnection();
String sql = "select filename from pds_board1 where seq = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, to.getSeq() );
rs = pstmt.executeQuery();
String filename = null;
if ( rs.next() ) {
filename = rs.getString( "filename" );
}
sql = "delete from pds_board1 where seq = ? and password = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setString( 1, to.getSeq() );
pstmt.setString( 2, to.getPassword() );
int result = pstmt.executeUpdate();
if ( result == 0 ) {
flag = 1;
} else if ( result == 1 ) {
flag = 0;
if ( filename != null ) {
File file = new File( "C:/Java/jsp-workspace/PdsModel1Ex01/WebContent/upload/"+ filename );
file.delete();
}
}
} catch(SQLException e) {
System.out.println( "error: " + e.getMessage() );
} finally {
if ( rs != null ) try { rs.close(); } catch ( SQLException e ) {}
if ( pstmt != null ) try { pstmt.close(); } catch ( SQLException e ) {}
if ( conn != null ) try { conn.close(); } catch ( SQLException e ) {}
}
return flag;
}
//modify
public BoardTO boardModify( BoardTO to ) {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
conn = dataSource.getConnection();
String sql = "select writer, subject, content, mail, filename from pds_board1 where seq = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setString( 1, to.getSeq() );
rs = pstmt.executeQuery();
if ( rs.next() ) {
to.setSubject( rs.getString( "subject" ) );
to.setWriter( rs.getString( "writer" ) );
to.setContent( rs.getString( "content" ) );
if ( rs.getString( "filename" ) != null ) {
to.setFilename( rs.getString( "filename" ) );
} else {
to.setFilename( "" );
}
to.setMail( rs.getString( "mail" ) );
}
} catch(SQLException e) {
System.out.println( "error: " + e.getMessage() );
} finally {
if ( rs != null ) try { rs.close(); } catch ( SQLException e ) {}
if ( pstmt != null ) try { pstmt.close(); } catch ( SQLException e ) {}
if ( conn != null ) try { conn.close(); } catch ( SQLException e ) {}
}
return to;
}
//modify_ok
public int boardModifyOk( BoardTO to ) {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
int flag = 2;
try {
conn = dataSource.getConnection();
String sql = "select filename from pds_board1 where seq = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, to.getSeq());
rs = pstmt.executeQuery();
String oldFilename = null;
if ( rs.next() ) {
oldFilename = rs.getString( "filename" );
}
if ( to.getFilename() != null ) {
//수정에서 첨부파일이 있을 때
sql = "update pds_board1 set subject = ?, content = ?, mail = ?, filename = ?, filesize = ? where seq = ? and password = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setString( 1, to.getSubject() );
pstmt.setString( 2, to.getContent() );
pstmt.setString( 3, to.getMail() );
pstmt.setString( 4, to.getFilename() );
pstmt.setLong( 5, to.getFilesize() );
pstmt.setString( 6, to.getSeq() );
pstmt.setString( 7, to.getPassword() );
} else {
//수정에서 첨부파일이 없을 때
sql = "update pds_board1 set subject = ?, content = ?, mail = ? where seq = ? and password = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setString( 1, to.getSubject() );
pstmt.setString( 2, to.getContent() );
pstmt.setString( 3, to.getMail() );
pstmt.setString( 4, to.getSeq() );
pstmt.setString( 5, to.getPassword() );
}
int result = pstmt.executeUpdate();
if ( result == 0 ) {
flag = 1;
} else if ( result == 1 ) {
flag = 0;
if ( to.getFilename() != null && oldFilename != null ) {
//기존 첨부파일이 있고 추가된 첨부파일이 있을 경우 기존 파일은 삭제한다.
File file = new File( "C:/Java/jsp-workspace/PdsModel1Ex01/WebContent/upload/"+ oldFilename );
file.delete();
}
}
} catch(SQLException e) {
System.out.println( "error: " + e.getMessage() );
} finally {
if ( rs != null ) try { rs.close(); } catch ( SQLException e ) {}
if ( pstmt != null ) try { pstmt.close(); } catch ( SQLException e ) {}
if ( conn != null ) try { conn.close(); } catch ( SQLException e ) {}
}
return flag;
}
}
model2 패키지
-BoardAction.java (인터페이스)
package model2;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public interface BoardAction {
public void execute( HttpServletRequest request, HttpServletResponse response );
}
-ListAction.java
package model2;
import java.util.ArrayList;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import model1.BoardDAO;
import model1.BoardTO;
public class ListAction implements BoardAction {
@Override
public void execute(HttpServletRequest request, HttpServletResponse response) {
System.out.println( "ListAction 호출" );
BoardDAO dao = new BoardDAO();
ArrayList<BoardTO> lists = dao.boardList();
request.setAttribute( "lists", lists );
}
}
-WriteAction.java
package model2;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class WriteAction implements BoardAction {
@Override
public void execute(HttpServletRequest request, HttpServletResponse response) {
System.out.println( "WriteAction 호출" );
}
}
-WriteOkAction.java
package model2;
import java.io.File;
import java.io.IOException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.oreilly.servlet.MultipartRequest;
import com.oreilly.servlet.multipart.DefaultFileRenamePolicy;
import model1.BoardDAO;
import model1.BoardTO;
public class WriteOkAction implements BoardAction {
@Override
public void execute(HttpServletRequest request, HttpServletResponse response) {
try {
System.out.println( "WriteOkAction 호출" );
String uploadPath = "C:/Java/jsp-workspace/PdsModel2Ex01/WebContent/upload";
int maxFileSize = 1024 * 1024 * 10;
String encType = "utf-8";
MultipartRequest multi
= new MultipartRequest( request, uploadPath, maxFileSize, encType, new DefaultFileRenamePolicy() );
String subject = multi.getParameter( "subject" );
String writer = multi.getParameter( "writer" );
//필수입력항목이 아닌경우 아래와 같이 값을 검사하고 저장해야 한다.
String mail = "";
if ( !multi.getParameter( "mail1" ).equals("") && !multi.getParameter( "mail2" ).equals("") ) {
mail = multi.getParameter( "mail1" ) + "@" + multi.getParameter( "mail2" );
}
String password = multi.getParameter( "password" );
String content = multi.getParameter( "content" );
String wip = request.getRemoteAddr(); //환경값만 request사용
String filename = multi.getFilesystemName( "upload" );
File file = multi.getFile( "upload" );
long filesize = 0;
if ( file != null ) {
filesize = file.length();
}
BoardTO to = new BoardTO();
BoardDAO dao = new BoardDAO();
to.setSubject(subject);
to.setWriter(writer);
to.setMail(mail);
to.setPassword(password);
to.setContent(content);
to.setWip(wip);
to.setFilename(filename);
to.setFilesize(filesize);
int flag = dao.boardWriteOk(to);
request.setAttribute( "flag", flag );
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
-ViewAction.java
package model2;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import model1.BoardDAO;
import model1.BoardTO;
public class ViewAction implements BoardAction {
@Override
public void execute(HttpServletRequest request, HttpServletResponse response) {
System.out.println( "ViewAction 호출" );
String seq = request.getParameter( "seq" );
BoardTO to = new BoardTO();
to.setSeq( seq );
BoardDAO dao = new BoardDAO();
to = dao.boardView( to );
request.setAttribute( "to", to );
}
}
-DeleteAction.java
package model2;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import model1.BoardDAO;
import model1.BoardTO;
public class DeleteAction implements BoardAction {
@Override
public void execute(HttpServletRequest request, HttpServletResponse response) {
System.out.println( "DeleteAction 호출" );
String seq = request.getParameter( "seq" );
BoardTO to = new BoardTO();
to.setSeq(seq);
BoardDAO dao = new BoardDAO();
to = dao.boardDelete(to);
request.setAttribute( "to", to );
}
}
-DeleteOkAction.java
package model2;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import model1.BoardDAO;
import model1.BoardTO;
public class DeleteOkAction implements BoardAction {
@Override
public void execute(HttpServletRequest request, HttpServletResponse response) {
System.out.println( "DeleteOkAction 호출" );
String seq = request.getParameter( "seq" );
String password = request.getParameter( "password" );
BoardTO to = new BoardTO();
to.setSeq(seq);
to.setPassword(password);
BoardDAO dao = new BoardDAO();
int flag = dao.boardDeleteOk(to);
request.setAttribute( "flag", flag );
}
}
-ModifyAction.java
package model2;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import model1.BoardDAO;
import model1.BoardTO;
public class ModifyAction implements BoardAction {
@Override
public void execute(HttpServletRequest request, HttpServletResponse response) {
System.out.println( "ModifyAction 호출" );
String seq = request.getParameter( "seq" );
BoardTO to = new BoardTO();
to.setSeq(seq);
BoardDAO dao = new BoardDAO();
to = dao.boardModify(to);
request.setAttribute( "to", to );
}
}
-ModifyOkAction.java
package model2;
import java.io.File;
import java.io.IOException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.oreilly.servlet.MultipartRequest;
import com.oreilly.servlet.multipart.DefaultFileRenamePolicy;
import model1.BoardDAO;
import model1.BoardTO;
public class ModifyOkAction implements BoardAction {
@Override
public void execute(HttpServletRequest request, HttpServletResponse response) {
try {
System.out.println( "ModifyOkAction 호출" );
String uploadPath = "C:/Java/jsp-workspace/PdsModel1Ex01/WebContent/upload";
int maxFileSize = 1024 * 1024 * 10;
String encType = "utf-8";
MultipartRequest multi
= new MultipartRequest( request, uploadPath, maxFileSize, encType, new DefaultFileRenamePolicy() );
String seq = multi.getParameter( "seq" );
String password = multi.getParameter( "password" );
String subject = multi.getParameter( "subject" );
String content = multi.getParameter( "content" );
String mail = "";
if ( !multi.getParameter( "mail1" ).equals("") && !multi.getParameter( "mail2" ).equals("") ) {
mail = multi.getParameter( "mail1" ) + "@" + multi.getParameter( "mail2" );
}
String newFilename = multi.getFilesystemName( "upload" );
File newFile = multi.getFile( "upload" );
long newFilesize = 0;
if ( newFile != null ) {
newFilesize = newFile.length();
}
BoardTO to = new BoardTO();
BoardDAO dao = new BoardDAO();
to.setSeq(seq);
to.setPassword(password);
to.setSubject(subject);
to.setContent(content);
to.setMail(mail);
to.setFilename( newFilename );
to.setFilesize(newFilesize);
int flag = dao.boardModifyOk(to);
request.setAttribute( "flag", flag );
request.setAttribute( "seq", to.getSeq() );
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
servlet 패키지
-controllerEx01.java
package servlet;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import model2.BoardAction;
import model2.DeleteAction;
import model2.DeleteOkAction;
import model2.ListAction;
import model2.ModifyAction;
import model2.ModifyOkAction;
import model2.ViewAction;
import model2.WriteAction;
import model2.WriteOkAction;
@WebServlet("*.do")
public class controllerEx01 extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
this.doProcess(request, response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
this.doProcess(request, response);
}
protected void doProcess(HttpServletRequest request, HttpServletResponse response) {
try {
request.setCharacterEncoding( "utf-8" );
String action = request.getRequestURI().replaceAll( request.getContextPath(), "" );
String url = "";
BoardAction boardAction = null;
if ( action.equals( "/*.do" ) || action.equals( "/list.do" ) ) {
boardAction = new ListAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_list1.jsp";
} else if ( action.equals( "/view.do" ) ) {
boardAction = new ViewAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_view1.jsp";
} else if ( action.equals( "/write.do" ) ) {
boardAction = new WriteAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_write1.jsp";
} else if ( action.equals( "/write_ok.do" ) ) {
boardAction = new WriteOkAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_write1_ok.jsp";
} else if ( action.equals( "/delete.do" ) ) {
boardAction = new DeleteAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_delete1.jsp";
} else if ( action.equals( "/delete_ok.do" ) ) {
boardAction = new DeleteOkAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_delete1_ok.jsp";
} else if ( action.equals( "/modify.do" ) ) {
boardAction = new ModifyAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_modify1.jsp";
} else if ( action.equals( "/modify_ok.do" ) ) {
boardAction = new ModifyOkAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_modify1_ok.jsp";
}
RequestDispatcher dispatcher = request.getRequestDispatcher( url );
dispatcher.forward(request, response);
} catch (UnsupportedEncodingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (ServletException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
views폴더의 jsp파일들
-Board_list1.jsp
<%@page import="model1.BoardTO"%>
<%@page import="model1.BoardDAO"%>
<%@page import="java.util.ArrayList"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
StringBuffer sbHtml = new StringBuffer();
ArrayList<BoardTO> lists = (ArrayList)request.getAttribute( "lists" );
int totalRecord = lists.size();
for ( BoardTO to : lists ) {
sbHtml.append( " <tr> " );
sbHtml.append( " <td> </td> " );
sbHtml.append( " <td>" + to.getSeq() + "</td> " );
sbHtml.append( " <td class='left'> ");
sbHtml.append( " <a href='./view.do?seq=" + to.getSeq() + "'>" + to.getSubject() + "</a> ");
if( to.getWgap() == 0 ) {
sbHtml.append( " <img src='./images/icon_hot.gif' alt='HOT'> ");
}
sbHtml.append( " </td> " );
sbHtml.append( " <td>" + to.getWriter() + "</td> " );
sbHtml.append( " <td>" + to.getWdate() + "</td> " );
sbHtml.append( " <td>" + to.getHit() + "</td> " );
if ( to.getFilesize() != 0 ) {
sbHtml.append( " <td><img src='./images/icon_file.gif' /></td> " );
} else {
sbHtml.append( " <td> </td> " );
}
sbHtml.append( " </tr> " );
}
%>
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0,minimum-scale=1.0,maximum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<title>Insert title here</title>
<link rel="stylesheet" type="text/css" href="./css/board_list.css">
</head>
<body>
<!-- 상단 디자인 -->
<div class="con_title">
<h3>게시판</h3>
<p>HOME > 게시판 > <strong>게시판</strong></p>
</div>
<div class="con_txt">
<div class="contents_sub">
<div class="board_top">
<div class="bold">총 <span class="txt_orange"><%= totalRecord %></span>건</div>
</div>
<!--게시판-->
<div class="board">
<table>
<tr>
<th width="3%"> </th>
<th width="5%">번호</th>
<th>제목</th>
<th width="10%">글쓴이</th>
<th width="17%">등록일</th>
<th width="5%">조회</th>
<th width="3%"> </th>
</tr>
<%= sbHtml %>
</table>
</div>
<!--//게시판-->
<div class="align_right">
<input type="button" value="쓰기" class="btn_write btn_txt01" style="cursor: pointer;" onclick="location.href='./write.do'" />
</div>
</div>
</div>
<!--//하단 디자인 -->
</body>
</html>
-Board_write1.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0,minimum-scale=1.0,maximum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<title>Insert title here</title>
<link rel="stylesheet" type="text/css" href="./css/board_write.css">
<script type="text/javascript">
window.onload = function() {
document.getElementById( 'submit1' ).onclick = function() {
if ( document.wfrm.info.checked == false ) {
alert( '동의를 해주세요' );
return false;
}
if ( document.wfrm.writer.value.trim() == '' ) {
alert( '글쓴이를 입력해주세요' );
return false;
}
if ( document.wfrm.subject.value.trim() == '' ) {
alert( '제목을 입력해주세요' );
return false;
}
if ( document.wfrm.password.value.trim() == '' ) {
alert( '비밀번호를 입력해주세요' );
return false;
}
document.wfrm.submit();
}
}
</script>
</head>
<body>
<!-- 상단 디자인 -->
<div class="con_title">
<h3>게시판</h3>
<p>HOME > 게시판 > <strong>게시판</strong></p>
</div>
<div class="con_txt">
<form action="./write_ok.do" method="post" name="wfrm" enctype="multipart/form-data">
<div class="contents_sub">
<!--게시판-->
<div class="board_write">
<table>
<tr>
<th class="top">글쓴이</th>
<td class="top" colspan="3"><input type="text" name="writer" value="" class="board_view_input_mail" maxlength="5" /></td>
</tr>
<tr>
<th>제목</th>
<td colspan="3"><input type="text" name="subject" value="" class="board_view_input" /></td>
</tr>
<tr>
<th>비밀번호</th>
<td colspan="3"><input type="password" name="password" value="" class="board_view_input_mail"/></td>
</tr>
<tr>
<th>내용</th>
<td colspan="3"><textarea name="content" class="board_editor_area"></textarea></td>
</tr>
<tr>
<th>이메일</th>
<td colspan="3"><input type="text" name="mail1" value="" class="board_view_input_mail"/> @ <input type="text" name="mail2" value="" class="board_view_input_mail"/></td>
</tr>
<tr>
<th>첨부파일</th>
<td colspan="3">
<input type="file" name="upload" value="파일첨부" class="board_view_input" />
</td>
</tr>
</table>
<table>
<tr>
<br />
<td style="text-align:left;border:1px solid #e0e0e0;background-color:f9f9f9;padding:5px">
<div style="padding-top:7px;padding-bottom:5px;font-weight:bold;padding-left:7px;font-family: Gulim,Tahoma,verdana;">※ 개인정보 수집 및 이용에 관한 안내</div>
<div style="padding-left:10px;">
<div style="width:97%;height:95px;font-size:11px;letter-spacing: -0.1em;border:1px solid #c5c5c5;background-color:#fff;padding-left:14px;padding-top:7px;">
1. 수집 개인정보 항목 : 회사명, 담당자명, 메일 주소, 전화번호, 홈페이지 주소, 팩스번호, 주소 <br />
2. 개인정보의 수집 및 이용목적 : 제휴신청에 따른 본인확인 및 원활한 의사소통 경로 확보 <br />
3. 개인정보의 이용기간 : 모든 검토가 완료된 후 3개월간 이용자의 조회를 위하여 보관하며, 이후 해당정보를 지체 없이 파기합니다. <br />
4. 그 밖의 사항은 개인정보취급방침을 준수합니다.
</div>
</div>
<div style="padding-top:7px;padding-left:5px;padding-bottom:7px;font-family: Gulim,Tahoma,verdana;">
<input type="checkbox" name="info" value="1" class="input_radio"> 개인정보 수집 및 이용에 대해 동의합니다.
</div>
</td>
</tr>
</table>
</div>
<div class="btn_area">
<div class="align_left">
<input type="button" value="목록" class="btn_list btn_txt02" style="cursor: pointer;" onclick="location.href='./list.do'" />
</div>
<div class="align_right">
<input type="button" id="submit1" value="쓰기" class="btn_write btn_txt01" style="cursor: pointer;" />
</div>
</div>
<!--//게시판-->
</div>
</form>
</div>
<!-- 하단 디자인 -->
</body>
</html>
-Board_write1_ok.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
request.setCharacterEncoding( "utf-8" );
int flag = (Integer)request.getAttribute( "flag" );
out.println( " <script type='text/javascript'> " );
if( flag == 0 ) {
out.println( " alert('글쓰기에 성공했습니다.'); " );
out.println( " location.href='./list.do'" );
} else {
out.println( " alert('글쓰기에 실패했습니다.'); " );
out.println( " history.back(); " );
}
out.println( " </script> " );
%>
-Board_view1.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@page import="model1.BoardTO"%>
<%
request.setCharacterEncoding( "utf-8" );
BoardTO to = (BoardTO)request.getAttribute( "to" );
String seq = to.getSeq();
String subject = to.getSubject();
String writer = to.getWriter();
String mail = to.getMail();
String wip = to.getWip();
String wdate = to.getWdate();
String hit = to.getHit();
String content = to.getContent();
String filename = to.getFilename();
long filesize = to.getFilesize();
String file = to.getFile();
%>
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0,minimum-scale=1.0,maximum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<title>Insert title here</title>
<link rel="stylesheet" type="text/css" href="./css/board_view.css">
</head>
<body>
<!-- 상단 디자인 -->
<div class="con_title">
<h3>게시판</h3>
<p>HOME > 게시판 > <strong>게시판</strong></p>
</div>
<div class="con_txt">
<div class="contents_sub">
<!--게시판-->
<div class="board_view">
<table>
<tr>
<th width="10%">제목</th>
<td width="60%"><%= subject %></td>
<th width="10%">등록일</th>
<td width="20%"><%= wdate %></td>
</tr>
<tr>
<th>글쓴이</th>
<td><%= writer %>(<%= mail %>)(<%= wip %>)</td>
<th>조회</th>
<td><%= hit %></td>
</tr>
<tr>
<th>첨부 파일</th>
<td><%= file %></td>
</tr>
<tr>
<td colspan="4" height="200" valign="top" style="padding: 20px; line-height: 160%"><%= content %></td>
</tr>
</table>
</div>
<div class="btn_area">
<div class="align_left">
<input type="button" value="목록" class="btn_list btn_txt02" style="cursor: pointer;" onclick="location.href='./list.do'" />
</div>
<div class="align_right">
<input type="button" value="수정" class="btn_list btn_txt02" style="cursor: pointer;" onclick="location.href='./modify.do?seq=<%= seq %>'" />
<input type="button" value="삭제" class="btn_list btn_txt02" style="cursor: pointer;" onclick="location.href='./delete.do?seq=<%= seq %>'" />
<input type="button" value="쓰기" class="btn_write btn_txt01" style="cursor: pointer;" onclick="location.href='./write.do'" />
</div>
</div>
<!--//게시판-->
</div>
</div>
<!-- 하단 디자인 -->
</body>
</html>
-Board_delete1.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@page import="model1.BoardTO"%>
<%
request.setCharacterEncoding( "utf-8" );
BoardTO to = (BoardTO)request.getAttribute( "to" );
String seq = to.getSeq();
String subject = to.getSubject();
String writer = to.getWriter();
%>
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0,minimum-scale=1.0,maximum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<title>Insert title here</title>
<link rel="stylesheet" type="text/css" href="./css/board_write.css">
<script type="text/javascript">
window.onload = function() {
document.getElementById( 'submit1' ).onclick = function() {
if( document.dfrm.password.value.trim() == '' ) {
alert( '비밀번호를 입력해주세요' );
return false;
}
document.dfrm.submit();
}
}
</script>
</head>
<body>
<!-- 상단 디자인 -->
<div class="con_title">
<h3>게시판</h3>
<p>HOME > 게시판 > <strong>게시판</strong></p>
</div>
<div class="con_txt">
<form action="./delete_ok.do" method="post" name="dfrm">
<input type="hidden" name="seq" value="<%= seq %>">
<div class="contents_sub">
<!--게시판-->
<div class="board_write">
<table>
<tr>
<th class="top">글쓴이</th>
<td class="top" colspan="3"><input type="text" name="writer" value="<%= writer %>" class="board_view_input_mail" maxlength="5" readonly/></td>
</tr>
<tr>
<th>제목</th>
<td colspan="3"><input type="text" name="subject" value="<%= subject %>" class="board_view_input" readonly/></td>
</tr>
<tr>
<th>비밀번호</th>
<td colspan="3"><input type="password" name="password" value="" class="board_view_input_mail"/></td>
</tr>
</table>
</div>
<div class="btn_area">
<div class="align_left">
<input type="button" value="목록" class="btn_list btn_txt02" style="cursor: pointer;" onclick="location.href='./list.do'" />
<input type="button" value="보기" class="btn_list btn_txt02" style="cursor: pointer;" onclick="location.href='./view.do?seq=<%=seq %>'" />
</div>
<div class="align_right">
<input type="button" id="submit1" value="삭제" class="btn_write btn_txt01" style="cursor: pointer;" />
</div>
</div>
<!--//게시판-->
</div>
</form>
</div>
<!-- 하단 디자인 -->
</body>
</html>
-Board_delete1_ok.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@page import="model1.BoardTO"%>
<%@page import="model1.BoardDAO"%>
<%
request.setCharacterEncoding( "utf-8" );
int flag = (Integer)request.getAttribute( "flag" );
out.println( " <script type='text/javascript'> " );
if( flag == 0 ) {
out.println( " alert('글삭제에 성공했습니다.'); " );
out.println( " location.href='./list.do' " );
} else if ( flag == 1 ) {
out.println( " alert('비밀번호가 틀립니다.'); " );
out.println( " history.back(); " );
} else {
out.println( " alert('글삭제에 실패했습니다.'); " );
out.println( " history.back(); " );
}
out.println( " </script> " );
%>
-Board_modify1.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@page import="model1.BoardTO"%>
<%@page import="model1.BoardDAO"%>
<%
request.setCharacterEncoding( "utf-8" );
BoardTO to = (BoardTO)request.getAttribute( "to" );
String seq = to.getSeq();
String writer = to.getWriter();
String subject = to.getSubject();
String content = to.getContent();
String mail[] = null;
if ( to.getMail().equals("") ) {
mail = new String[] {"", ""};
} else {
mail = to.getMail().split("@");
}
String filename = to.getFilename();
%>
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,initial-scale=1.0,minimum-scale=1.0,maximum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<title>Insert title here</title>
<link rel="stylesheet" type="text/css" href="./css/board_write.css">
<script type="text/javascript">
window.onload = function() {
document.getElementById( 'submit2' ).onclick = function() {
if ( document.mfrm.subject.value.trim() == '' ) {
alert( '제목을 입력해주세요' );
return false;
}
if ( document.mfrm.password.value.trim() == '' ) {
alert( '비밀번호를 입력해주세요' );
return false;
}
if ( document.mfrm.content.value.trim() == '' ) {
alert( '내용을 입력해주세요' );
return false;
}
document.mfrm.submit();
}
}
</script>
</head>
<body>
<!-- 상단 디자인 -->
<div class="con_title">
<h3>게시판</h3>
<p>HOME > 게시판 > <strong>게시판</strong></p>
</div>
<div class="con_txt">
<form action="./modify_ok.do" method="post" name="mfrm" enctype="multipart/form-data">
<input type="hidden" name="seq" value="<%= seq %>">
<div class="contents_sub">
<!--게시판-->
<div class="board_write">
<table>
<tr>
<th class="top">글쓴이</th>
<td class="top" colspan="3"><input type="text" name="writer" value="<%= writer %>" class="board_view_input_mail" maxlength="5" readonly/></td>
</tr>
<tr>
<th>제목</th>
<td colspan="3"><input type="text" name="subject" value="<%= subject %>" class="board_view_input" /></td>
</tr>
<tr>
<th>비밀번호</th>
<td colspan="3"><input type="password" name="password" value="" class="board_view_input_mail"/></td>
</tr>
<tr>
<th>내용</th>
<td colspan="3"><textarea name="content" class="board_editor_area"><%= content %></textarea></td>
</tr>
<tr>
<th>이메일</th>
<td colspan="3"><input type="text" name="mail1" value="<%= mail[0] %>" class="board_view_input_mail"/> @ <input type="text" name="mail2" value="<%= mail[1] %>" class="board_view_input_mail"/></td>
</tr>
<tr>
<th>첨부파일</th>
<td colspan="3">
기존 파일명 : <%=filename %><br /><br />
<input type="file" name="upload" value="" class="board_view_input" />
</td>
</tr>
</table>
</div>
<div class="btn_area">
<div class="align_left">
<input type="button" value="목록" class="btn_list btn_txt02" style="cursor: pointer;" onclick="location.href='./list.do'" />
<input type="button" value="보기" class="btn_list btn_txt02" style="cursor: pointer;" onclick="location.href='./view.do?seq=<%=seq %>'" />
</div>
<div class="align_right">
<input type="button" id="submit2" value="수정" class="btn_write btn_txt01" style="cursor: pointer;" />
</div>
</div>
<!--//게시판-->
</div>
</form>
</div>
<!-- 하단 디자인 -->
</body>
</html>
-Board_modify1_ok.jsp
<%@page import="java.sql.ResultSet"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@page import="model1.BoardTO"%>
<%@page import="model1.BoardDAO"%>
<%@ page import="com.oreilly.servlet.multipart.DefaultFileRenamePolicy" %>
<%@ page import="com.oreilly.servlet.MultipartRequest" %>
<%@ page import="java.io.File" %>
<%
request.setCharacterEncoding( "utf-8" );
int flag = (Integer)request.getAttribute( "flag" );
String seq = (String)request.getAttribute( "seq" );
out.println( " <script type='text/javascript'> " );
if( flag == 0 ) {
out.println( " alert('글수정에 성공했습니다.'); " );
out.println( " location.href='./view.do?seq=" + seq + "'" );
} else if ( flag == 1 ) {
out.println( " alert('비밀번호가 틀립니다.'); " );
out.println( " history.back(); " );
} else {
out.println( " alert('글수정에 실패했습니다.'); " );
out.println( " history.back(); " );
}
out.println( " </script> " );
%>
[출처]
kic캠퍼스
jsp웹프로그래밍 - 최범균