지구정복
[Spring] 01/29 | Spring(기본사용법 복습, 파일업로드, json형식으로 출력하기, 데이터베이스 연동(DataSource이용, JdbcTemplate이용, 우편번호검색기)) 본문
[Spring] 01/29 | Spring(기본사용법 복습, 파일업로드, json형식으로 출력하기, 데이터베이스 연동(DataSource이용, JdbcTemplate이용, 우편번호검색기))
eeaarrtthh 2021. 1. 29. 17:391. Spring
1. 기본사용법 복습
새로운 스프링 레거시 프로젝트를 만든다.



그리고 빌드패스에서 자바라이브러리 버전 변경, facets에서 자바 버전 1.8로 변경한다.
그리고 pom.xml에서도 버전을 변경해준다.

index페이지를 시작하면 바로 페이지가 넘어가도록 만들어보자.
-HomeController.java
package com.exam.web01;
import java.text.DateFormat;
import java.util.Date;
import java.util.Locale;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
/**
* Handles requests for the application home page.
*/
@Controller
public class HomeController {
private static final Logger logger = LoggerFactory.getLogger(HomeController.class);
/**
* Simply selects the home view to render by returning its name.
*/
@RequestMapping(value = "index.do", method = RequestMethod.GET)
public String home(Locale locale, Model model) {
logger.info("Welcome home! The client locale is {}.", locale);
Date date = new Date();
DateFormat dateFormat = DateFormat.getDateTimeInstance(DateFormat.LONG, DateFormat.LONG, locale);
String formattedDate = dateFormat.format(date);
model.addAttribute("serverTime", formattedDate );
return "home";
}
}
-index.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
//페이지 자동으로 이동시키는 코드
response.sendRedirect( "index.do" );
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<ul>
<li><a href="index.do">index.do</a>
</ul>
</body>
</html>

또한 url에 http://localhost:8080/프로젝트명/~.jsp를 사용했다면
http://localhost:8080/프로젝트명/ 만 써도 특정 jsp파일이 실행되도록 설정해보자.
-web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.5" xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee https://java.sun.com/xml/ns/javaee/web-app_2_5.xsd">
<!-- url에 경로까지만 치면 특정 jsp파일로 이동시켜주는 코드 -->
<welcome-file-list>
<welcome-file>index.do</welcome-file>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
<!-- The definition of the Root Spring Container shared by all Servlets and Filters -->
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/spring/root-context.xml</param-value>
</context-param>
<!-- Creates the Spring Container shared by all Servlets and Filters -->
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
<filter>
<filter-name>encodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>utf-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>encodingFilter</filter-name>
<url-pattern>*.do</url-pattern>
</filter-mapping>
<!-- Processes application requests -->
<servlet>
<servlet-name>appServlet</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/spring/appServlet/servlet-context.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>appServlet</servlet-name>
<url-pattern>*.do</url-pattern>
</servlet-mapping>
</web-app>
경로까지만 입력하고 엔터치면 자동으로 index.do로 이동한다.


2. 파일업로드 구현하기
먼저 cos.jar를 다운받자. pom.xml에 아래코드를 추가한다.
<!-- https://mvnrepository.com/artifact/servlets.com/cos -->
<dependency>
<groupId>servlets.com</groupId>
<artifactId>cos</artifactId>
<version>05Nov2002</version>
</dependency>
-write.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<form action="write_ok.do" method="post" enctype="multipart/form-data">
파일 : <input type="file" name="upload" />
<input type="submit" value="파일전송" />
</form>
</body>
</html>
-HomeController.java
package com.exam.web01;
import java.io.IOException;
import java.text.DateFormat;
import java.util.Date;
import java.util.Locale;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import com.oreilly.servlet.MultipartRequest;
import com.oreilly.servlet.multipart.DefaultFileRenamePolicy;
@Controller
public class HomeController {
@RequestMapping( value="write.do" )
public String write() {
System.out.println( "write() 호출" );
return "write";
}
@RequestMapping( value="write_ok.do" )
public String write_ok(HttpServletRequest request, HttpServletResponse response) {
System.out.println( "write_ok() 호출" );
String uploadPath = "C:\\Java\\spring-workspace\\SpringWebEx01\\src\\main\\webapp\\upload";
int maxFileSize = 1024 * 1024 * 2;
String encType = "utf-8";
MultipartRequest multi = null;
try {
multi = new MultipartRequest(request, uploadPath, maxFileSize,
encType, new DefaultFileRenamePolicy() );
System.out.println( multi.getOriginalFileName("upload") );
System.out.println( multi.getFilesystemName("upload") );
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "write_ok";
}
}

만약에 파일말고 데이터를 받는다면 아래와 같이 "data"로 보내고 받아준다.
-write.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<form action="write_ok.do" method="post" enctype="multipart/form-data">
데이터 : <input type="text" name="data" /><br><br>
파일 : <input type="file" name="upload" />
<input type="submit" value="파일전송" />
</form>
</body>
</html>
-HomeController.java
package com.exam.web01;
import java.io.IOException;
import java.text.DateFormat;
import java.util.Date;
import java.util.Locale;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import com.oreilly.servlet.MultipartRequest;
import com.oreilly.servlet.multipart.DefaultFileRenamePolicy;
@Controller
public class HomeController {
@RequestMapping( value="write.do" )
public String write() {
System.out.println( "write() 호출" );
return "write";
}
@RequestMapping( value="write_ok.do" )
public String write_ok(HttpServletRequest request, HttpServletResponse response) {
System.out.println( "write_ok() 호출" );
String uploadPath = "C:\\Java\\spring-workspace\\SpringWebEx01\\src\\main\\webapp\\upload";
int maxFileSize = 1024 * 1024 * 2;
String encType = "utf-8";
MultipartRequest multi = null;
try {
multi = new MultipartRequest(request, uploadPath, maxFileSize,
encType, new DefaultFileRenamePolicy() );
System.out.println( multi.getOriginalFileName("upload") );
System.out.println( multi.getFilesystemName("upload") );
//file외에 다른 것을 받을 경우 "data"란 이름을 받는다.
System.out.println( multi.getParameter( "data" ) );
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "write_ok";
}
}
다른 방법으로 cos.jar외에 다른 라이브러리를 사용하는 것이다.
1. cos.jar
2. common-io / common-fileupload
common-io와 common-fileupload 라이브러리를 사용해보자.
아래 라이브러리 코드를 pom.xml에 붙여넣기 한다.
<!--common-io -->
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.8.0</version>
</dependency>
<!-- common-fileupload -->
<!-- https://mvnrepository.com/artifact/commons-fileupload/commons-fileupload -->
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.4</version>
</dependency>
파일의 크기 설정은 webapp/WEB-INF/spring/appServlet/servlet-context에서 한다.
1040576이 1MB이고, property의 value에 * 부호를 사용하지 못하므로 계산된 결과를 넣는다.
또한 common-io와 common-fileupload 라이브러리를 사용하면 업로드된 파일을 개발자가 설정한 폴더로 바로 옮기는 것이 아니라 톰캣내부 버퍼 공간에 저장했다고 옮기게 된다.
이때 이 임시공간의 크기를 설정하는 것이 maxInMemorySize이다.
-servlet-context
<?xml version="1.0" encoding="UTF-8"?>
<beans:beans xmlns="http://www.springframework.org/schema/mvc"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:beans="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/mvc https://www.springframework.org/schema/mvc/spring-mvc.xsd
http://www.springframework.org/schema/beans https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd">
<!-- DispatcherServlet Context: defines this servlet's request-processing infrastructure -->
<!-- Enables the Spring MVC @Controller programming model -->
<annotation-driven />
<!-- Handles HTTP GET requests for /resources/** by efficiently serving up static resources in the ${webappRoot}/resources directory -->
<resources mapping="/resources/**" location="/resources/" />
<!-- Resolves views selected for rendering by @Controllers to .jsp resources in the /WEB-INF/views directory -->
<beans:bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<beans:property name="prefix" value="/WEB-INF/views/" />
<beans:property name="suffix" value=".jsp" />
</beans:bean>
<!-- Upload component 설정 -->
<beans:bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver">
<beans:property name="maxUploadSize" value="1040576" />
<beans:property name="maxInMemorySize" value="1040576" />
<beans:property name="defaultEncoding" value="utf-8" />
</beans:bean>
<context:component-scan base-package="com.exam.web01" />
</beans:beans>
-HomeController.java
package com.exam.web01;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.text.DateFormat;
import java.util.Date;
import java.util.Locale;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.multipart.MultipartFile;
import com.oreilly.servlet.MultipartRequest;
import com.oreilly.servlet.multipart.DefaultFileRenamePolicy;
@Controller
public class HomeController {
@RequestMapping( value="write.do" )
public String write() {
System.out.println( "write() 호출" );
return "write";
}
@RequestMapping( value="write_ok1.do" )
public String write_ok1(HttpServletRequest request, HttpServletResponse response) {
System.out.println( "write_ok() 호출" );
String uploadPath = "C:\\Java\\spring-workspace\\SpringWebEx01\\src\\main\\webapp\\upload";
int maxFileSize = 1024 * 1024 * 2;
String encType = "utf-8";
MultipartRequest multi = null;
try {
multi = new MultipartRequest(request, uploadPath, maxFileSize,
encType, new DefaultFileRenamePolicy() );
System.out.println( multi.getOriginalFileName("upload") );
System.out.println( multi.getFilesystemName("upload") );
//file외에 다른 것을 받을 경우 "data"란 이름을 받는다.
System.out.println( multi.getParameter( "data" ) );
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "write_ok";
}
@RequestMapping( value="write_ok2.do" )
public String write_ok2( @RequestParam("upload") MultipartFile multipartFile ) {
System.out.println( "업로드 이름 : " + multipartFile.getName() );
System.out.println( "파일 이름 : " + multipartFile.getOriginalFilename() );
System.out.println( "파일 사이즈 : " + multipartFile.getSize() );
FileOutputStream fos = null;
String uploadPath = "C:\\Java\\spring-workspace\\SpringWebEx01\\src\\main\\webapp\\upload";
try {
fos = new FileOutputStream( uploadPath + "\\" + multipartFile.getOriginalFilename() );
fos.write( multipartFile.getBytes() );
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
if( fos != null ) try { fos.close(); } catch( IOException e ) {}
}
return "write_ok";
}
}

파일이름이 중복되면 오버라이팅된다. 따라서 이를 위해 파일이름변경이 필요하다 DefaultRenamePolicy알고리즘을 넣어야한다. 이 알고리즘은 예를 들어 파일업로드 시간이 다른데 파일 이름이 같은경우 나중에 올린 파일이름 뒤에 숫자를 붙여주거나 하는 식이다.
3. view페이지를 json형식으로 출력하기
맨 위와 같은 방법으로 새로운 프로젝트를 만든다.
simple-json 라이브러리를 복사해서 pom.xml에 붙여넣는다.
<!-- https://mvnrepository.com/artifact/com.googlecode.json-simple/json-simple -->
<dependency>
<groupId>com.googlecode.json-simple</groupId>
<artifactId>json-simple</artifactId>
<version>1.1.1</version>
</dependency>
-json1.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8" trimDirectiveWhitespaces="true" %>
<%@ page import="org.json.simple.JSONArray" %>
<%@ page import="org.json.simple.JSONObject" %>
<%
JSONArray jsonArray = new JSONArray();
JSONObject obj1 = new JSONObject();
obj1.put( "name", "name1" );
obj1.put( "publischer", "publischer1" );
obj1.put( "author", "author1" );
obj1.put( "price", "price1" );
JSONObject obj2 = new JSONObject();
obj2.put( "name", "name2" );
obj2.put( "publischer", "publischer2" );
obj2.put( "author", "author2" );
obj2.put( "price", "price2" );
jsonArray.add( obj1 );
jsonArray.add( obj2 );
out.println( jsonArray );
%>
-servlet-context.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans:beans xmlns="http://www.springframework.org/schema/mvc"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:beans="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/mvc https://www.springframework.org/schema/mvc/spring-mvc.xsd
http://www.springframework.org/schema/beans https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd">
<!-- DispatcherServlet Context: defines this servlet's request-processing infrastructure -->
<!-- Enables the Spring MVC @Controller programming model -->
<annotation-driven />
<!-- Handles HTTP GET requests for /resources/** by efficiently serving up static resources in the ${webappRoot}/resources directory -->
<resources mapping="/resources/**" location="/resources/" />
<!-- Resolves views selected for rendering by @Controllers to .jsp resources in the /WEB-INF/views directory -->
<beans:bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<beans:property name="prefix" value="/WEB-INF/views/" />
<beans:property name="suffix" value=".jsp" />
</beans:bean>
<context:component-scan base-package="com.exam.web01" />
</beans:beans>
위에 json1.jsp에서 json데이터를 만들어서 출력해도되고 아래처럼 Controller에서 만들어서 출력할 수 있다.
-HomeController.java
package com.exam.web01;
import java.util.HashMap;
import java.util.Locale;
import java.util.Map;
import org.json.simple.JSONValue;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller
public class HomeController {
@RequestMapping(value = "/json1.do" )
public String json1(Locale locale, Model model) {
return "json1";
}
@RequestMapping(value = "/json2.do" )
@ResponseBody
public String json2(Locale locale, Model model) {
Map<String, String> map = new HashMap<String, String>();
map.put( "name", "name1" );
map.put( "publischer", "publischer1" );
map.put( "author", "author1" );
map.put( "price", "price1" );
return JSONValue.toJSONString( map );
}
}
-index.jsp(실행jsp)
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<ul>
<li><a href="json1.do">json1.do</a>
<li><a href="json2.do">json2.do</a>
</ul>
</body>
</html>

또한 리스트형태로 json을 만들어서 출력할 수 있다.
-HomeController.java
package com.exam.web01;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import org.json.simple.JSONValue;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller
public class HomeController {
@RequestMapping(value = "/json1.do" )
public String json1(Locale locale, Model model) {
return "json1";
}
@RequestMapping(value = "/json2.do" )
@ResponseBody
public String json2(Locale locale, Model model) {
List<Map> lists = new ArrayList<Map>();
Map<String, String> map1 = new HashMap<String, String>();
map1.put( "name", "name1" );
map1.put( "publischer", "publischer1" );
map1.put( "author", "author1" );
map1.put( "price", "price1" );
Map<String, String> map2 = new HashMap<String, String>();
map2.put( "name", "name2" );
map2.put( "publischer", "publischer2" );
map2.put( "author", "author2" );
map2.put( "price", "price2" );
lists.add( map1 );
lists.add( map2 );
return JSONValue.toJSONString( lists );
}
}

4. 데이터베이스 연동하기
4.1 DataSource 이용
다시 새로운 프로젝트를 만든다.
-pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.exam</groupId>
<artifactId>web03</artifactId>
<name>SpringWebEx03</name>
<packaging>war</packaging>
<version>1.0.0-BUILD-SNAPSHOT</version>
<properties>
<java-version>1.8</java-version>
<org.springframework-version>5.2.8.RELEASE</org.springframework-version>
<org.aspectj-version>1.9.5</org.aspectj-version>
<org.slf4j-version>1.7.3</org.slf4j-version>
</properties>
<dependencies>
<!-- Spring -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>${org.springframework-version}</version>
<exclusions>
<!-- Exclude Commons Logging in favor of SLF4j -->
<exclusion>
<groupId>commons-logging</groupId>
<artifactId>commons-logging</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>${org.springframework-version}</version>
</dependency>
<!-- AspectJ -->
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjrt</artifactId>
<version>${org.aspectj-version}</version>
</dependency>
<!-- Logging -->
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>${org.slf4j-version}</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>jcl-over-slf4j</artifactId>
<version>${org.slf4j-version}</version>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-log4j12</artifactId>
<version>${org.slf4j-version}</version>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.15</version>
<exclusions>
<exclusion>
<groupId>javax.mail</groupId>
<artifactId>mail</artifactId>
</exclusion>
<exclusion>
<groupId>javax.jms</groupId>
<artifactId>jms</artifactId>
</exclusion>
<exclusion>
<groupId>com.sun.jdmk</groupId>
<artifactId>jmxtools</artifactId>
</exclusion>
<exclusion>
<groupId>com.sun.jmx</groupId>
<artifactId>jmxri</artifactId>
</exclusion>
</exclusions>
<scope>runtime</scope>
</dependency>
<!-- @Inject -->
<dependency>
<groupId>javax.inject</groupId>
<artifactId>javax.inject</artifactId>
<version>1</version>
</dependency>
<!-- Servlet -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>servlet-api</artifactId>
<version>2.5</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>javax.servlet.jsp</groupId>
<artifactId>jsp-api</artifactId>
<version>2.1</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
<!-- Test -->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.7</version>
<scope>test</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/servlets.com/cos -->
<dependency>
<groupId>servlets.com</groupId>
<artifactId>cos</artifactId>
<version>05Nov2002</version>
</dependency>
<!-- https://mvnrepository.com/artifact/commons-io/commons-io -->
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.8.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/commons-fileupload/commons-fileupload -->
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.4</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.googlecode.json-simple/json-simple -->
<dependency>
<groupId>com.googlecode.json-simple</groupId>
<artifactId>json-simple</artifactId>
<version>1.1.1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.mariadb.jdbc/mariadb-java-client -->
<dependency>
<groupId>org.mariadb.jdbc</groupId>
<artifactId>mariadb-java-client</artifactId>
<version>2.7.1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.springframework/spring-jdbc -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>${org.springframework-version}</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<artifactId>maven-eclipse-plugin</artifactId>
<version>2.9</version>
<configuration>
<additionalProjectnatures>
<projectnature>org.springframework.ide.eclipse.core.springnature</projectnature>
</additionalProjectnatures>
<additionalBuildcommands>
<buildcommand>org.springframework.ide.eclipse.core.springbuilder</buildcommand>
</additionalBuildcommands>
<downloadSources>true</downloadSources>
<downloadJavadocs>true</downloadJavadocs>
</configuration>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>2.5.1</version>
<configuration>
<source>1.6</source>
<target>1.6</target>
<compilerArgument>-Xlint:all</compilerArgument>
<showWarnings>true</showWarnings>
<showDeprecation>true</showDeprecation>
</configuration>
</plugin>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>exec-maven-plugin</artifactId>
<version>1.2.1</version>
<configuration>
<mainClass>org.test.int1.Main</mainClass>
</configuration>
</plugin>
</plugins>
</build>
</project>
-web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.5" xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee https://java.sun.com/xml/ns/javaee/web-app_2_5.xsd">
<!-- The definition of the Root Spring Container shared by all Servlets and Filters -->
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/spring/root-context.xml</param-value>
</context-param>
<!-- Creates the Spring Container shared by all Servlets and Filters -->
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
<filter>
<filter-name>encodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>utf-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>encodingFilter</filter-name>
<url-pattern>*.do</url-pattern>
</filter-mapping>
<!-- Processes application requests -->
<servlet>
<servlet-name>appServlet</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/spring/appServlet/servlet-context.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>appServlet</servlet-name>
<url-pattern>*.do</url-pattern>
</servlet-mapping>
</web-app>
-root-context.xml (데이터베이스 연결설정을 작성한다.)
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans https://www.springframework.org/schema/beans/spring-beans.xsd">
<!-- Root Context: defines shared resources visible to all other web components -->
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="org.mariadb.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3307/sample" />
<property name="username" value="root" />
<property name="password" value="!123456" />
</bean>
</beans>
-HomeController.java
여기서 autowired는 주입하려는 객체의 빈을 자동으로 찾아줘서 주입시켜주는 어노테이션 기능이다.
찾는 순서는 먼저 같은 타입 -> bean의 이름 순으로 찾는다.
package com.exam.web03;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Locale;
import javax.sql.DataSource;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class HomeController {
@Autowired
private DataSource dataSource;
@RequestMapping(value = "/jdbc1.do" )
public String jdbc1(Locale locale, Model model) {
System.out.println( "dataSource : " + dataSource );
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
conn = dataSource.getConnection();
String sql = "select now() as now";
pstmt = conn.prepareStatement(sql);
rs = pstmt.executeQuery();
if( rs.next() ) {
System.out.println( "현재 시간: " + rs.getString( "now" ) );
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
if( rs != null ) try { rs.close(); } catch( SQLException e ) {}
if( pstmt != null ) try { pstmt.close(); } catch( SQLException e ) {}
if( conn != null ) try { conn.close(); } catch( SQLException e ) {}
}
return "jdbc1";
}
}
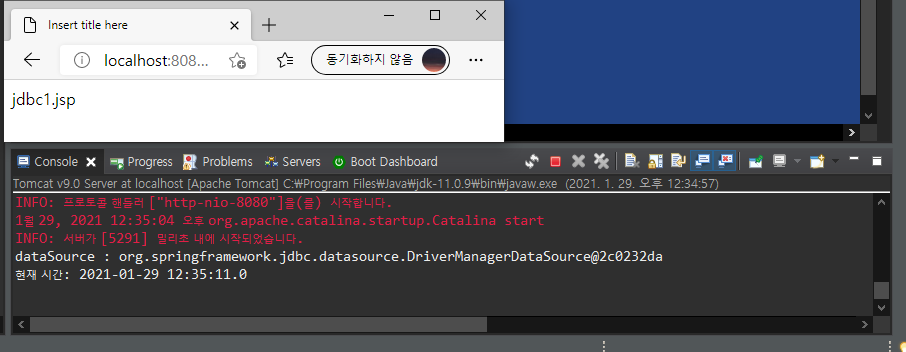
4.2 JdbcTemplate 이용
다른 방법으로 데이터베이스에 연동해보자.
(참고 : blog.naver.com/rnalsttnn2/222186074127 )
jdbcTemplate란 스프링에서 제공하는 SQL 연산들을 수행할 수 있도록 도와주는 JDBC 코드용 기본 템플릿이다.
jdbcTemplate의 기능에는 JDBC드라이버로드, 데이터베이스 연결, sql query, 데이터베이스 연결해제 등이 있다.
사용법은 다음과 같다.
1. pom에 dependency 추가
2. servlet-context.xml에 bean생성
3. Controller.java에 jdbcTemplate 선언하고 setter 메서드 추가
4. contant 클래스 만들고 public static jdbcTemplate 선언
5. dao에 jdbcTemplate 이용하여 메서드 생성
주로 3가지 메서드를 이용하여 게시판을 만들 수 있다.
- update() : 주로 sql 연산을 통해 데이터베이스를 갱신시켜줄 때 사용한다.
- queryForObject() : select쿼리를 실행하여 한 개의 객체를 받아올 때 사용한다.
- query() : 많은 결과 값(로우값)을 처리할 수 있는 메서드이다.
query( 1. sql문, 2. new BeanPropertyRowMapper<각 로우의 타입>(각 로우의타입.class), 3. 인자값 );
1. sql문 : 말 그대로 sql문이고 preparedStatement도 사용가능하다.
2. 는 1의 결과가 리턴되는 타입이다. resultSet으로 사용할 수 있다.
3. 은 생략가능하며 1에서 preparedStatement로 사용되었을 경우 ? 값을 넣으면 된다.
-root-context.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans https://www.springframework.org/schema/beans/spring-beans.xsd">
<!-- Root Context: defines shared resources visible to all other web components -->
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="org.mariadb.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3307/sample" />
<property name="username" value="root" />
<property name="password" value="!123456" />
</bean>
<bean id="jdbcTemplate" class="org.springframework.jdbc.core.JdbcTemplate">
<constructor-arg ref="dataSource" />
<property name="dataSource" ref="dataSource" />
</bean>
</beans>
-Homecontroller.java
package com.exam.web03;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Locale;
import javax.sql.DataSource;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class HomeController {
@Autowired
private DataSource dataSource;
@Autowired
private JdbcTemplate jdbcTemplate;
@RequestMapping(value = "/jdbc1.do" )
public String jdbc1(Locale locale, Model model) {
System.out.println( "dataSource : " + dataSource );
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
conn = dataSource.getConnection();
String sql = "select now() as now";
pstmt = conn.prepareStatement(sql);
rs = pstmt.executeQuery();
if( rs.next() ) {
System.out.println( "현재 시간: " + rs.getString( "now" ) );
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
if( rs != null ) try { rs.close(); } catch( SQLException e ) {}
if( pstmt != null ) try { pstmt.close(); } catch( SQLException e ) {}
if( conn != null ) try { conn.close(); } catch( SQLException e ) {}
}
return "jdbc1";
}
@RequestMapping(value = "/jdbc2.do" )
public String jdbc2(Locale locale, Model model) {
System.out.println( "jdbcTemplate : " + jdbcTemplate );
String result = jdbcTemplate.queryForObject("select now()", String.class );
System.out.println( "result : " + result );
return "jdbc1";
}
}
웹브라우저에서 주소값을 jdbc2.do를 입력하면 아래와 같은 결과가 나온다.

이번에는 DAO를 만들어서 실행시켜보자.
src/main/java에 SampleDAO.java를 만든다.
package com.exam.web03;
import org.springframework.jdbc.core.JdbcTemplate;
public class SampleDAO {
private JdbcTemplate jdbcTemplate;
public SampleDAO( JdbcTemplate jdbcTemplate ) {
this.jdbcTemplate = jdbcTemplate;
}
public String selectNow() {
System.out.println( "jdbcTemplate : " + jdbcTemplate );
return "";
}
}
-Homecontroller.java
package com.exam.web03;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Locale;
import javax.sql.DataSource;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class HomeController {
@Autowired
private DataSource dataSource;
@Autowired
private JdbcTemplate jdbcTemplate;
@RequestMapping(value = "/jdbc1.do" )
public String jdbc1(Locale locale, Model model) {
System.out.println( "dataSource : " + dataSource );
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
conn = dataSource.getConnection();
String sql = "select now() as now";
pstmt = conn.prepareStatement(sql);
rs = pstmt.executeQuery();
if( rs.next() ) {
System.out.println( "현재 시간: " + rs.getString( "now" ) );
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
if( rs != null ) try { rs.close(); } catch( SQLException e ) {}
if( pstmt != null ) try { pstmt.close(); } catch( SQLException e ) {}
if( conn != null ) try { conn.close(); } catch( SQLException e ) {}
}
return "jdbc1";
}
@RequestMapping(value = "/jdbc2.do" )
public String jdbc2(Locale locale, Model model) {
System.out.println( "jdbcTemplate : " + jdbcTemplate );
String result = jdbcTemplate.queryForObject("select now()", String.class );
System.out.println( "result : " + result );
return "jdbc1";
}
@RequestMapping(value = "/jdbc3.do" )
public String jdbc3(Locale locale, Model model) {
SampleDAO dao = new SampleDAO(jdbcTemplate);
System.out.println( dao.selectNow() );
return "jdbc1";
}
}
웹브라우저에서 jdbc3.do를 입력하면 아래와 같은 결과가 나온다.

이번에는 DAO에서 생성자로 직접 jdbcTemplate객체를 주지 말고 Autowired로 사용해보자.
-SampleDAO.java
package com.exam.web03;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Repository;
@Repository
public class SampleDAO {
@Autowired
private JdbcTemplate jdbcTemplate;
/*
* public SampleDAO( JdbcTemplate jdbcTemplate ) { this.jdbcTemplate =
* jdbcTemplate; }
*/
public String selectNow() {
System.out.println( "jdbcTemplate : " + jdbcTemplate );
return "";
}
}
-HomeController.java
package com.exam.web03;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Locale;
import javax.sql.DataSource;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class HomeController {
@Autowired
private DataSource dataSource;
@Autowired
private JdbcTemplate jdbcTemplate;
@Autowired
private SampleDAO dao;
@RequestMapping(value = "/jdbc1.do" )
public String jdbc1(Locale locale, Model model) {
System.out.println( "dataSource : " + dataSource );
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
conn = dataSource.getConnection();
String sql = "select now() as now";
pstmt = conn.prepareStatement(sql);
rs = pstmt.executeQuery();
if( rs.next() ) {
System.out.println( "현재 시간: " + rs.getString( "now" ) );
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
if( rs != null ) try { rs.close(); } catch( SQLException e ) {}
if( pstmt != null ) try { pstmt.close(); } catch( SQLException e ) {}
if( conn != null ) try { conn.close(); } catch( SQLException e ) {}
}
return "jdbc1";
}
@RequestMapping(value = "/jdbc2.do" )
public String jdbc2(Locale locale, Model model) {
System.out.println( "jdbcTemplate : " + jdbcTemplate );
String result = jdbcTemplate.queryForObject("select now()", String.class );
System.out.println( "result : " + result );
return "jdbc1";
}
@RequestMapping(value = "/jdbc3.do" )
public String jdbc3(Locale locale, Model model) {
//SampleDAO dao = new SampleDAO(jdbcTemplate);
System.out.println( dao.selectNow() );
return "jdbc1";
}
}
다시 브라우저에서 jdbc3.do를 입력하면 아래와 같이 결과가 나온다.

실습) 우편번호 검색기 만들기

1. DataSource 방식
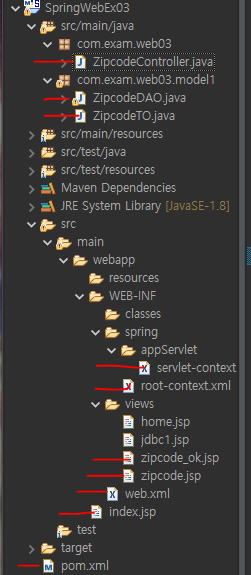
-pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.exam</groupId>
<artifactId>web03</artifactId>
<name>SpringWebEx03</name>
<packaging>war</packaging>
<version>1.0.0-BUILD-SNAPSHOT</version>
<properties>
<java-version>1.8</java-version>
<org.springframework-version>5.2.8.RELEASE</org.springframework-version>
<org.aspectj-version>1.9.5</org.aspectj-version>
<org.slf4j-version>1.7.3</org.slf4j-version>
</properties>
<dependencies>
<!-- Spring -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>${org.springframework-version}</version>
<exclusions>
<!-- Exclude Commons Logging in favor of SLF4j -->
<exclusion>
<groupId>commons-logging</groupId>
<artifactId>commons-logging</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>${org.springframework-version}</version>
</dependency>
<!-- AspectJ -->
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjrt</artifactId>
<version>${org.aspectj-version}</version>
</dependency>
<!-- Logging -->
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>${org.slf4j-version}</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>jcl-over-slf4j</artifactId>
<version>${org.slf4j-version}</version>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-log4j12</artifactId>
<version>${org.slf4j-version}</version>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.15</version>
<exclusions>
<exclusion>
<groupId>javax.mail</groupId>
<artifactId>mail</artifactId>
</exclusion>
<exclusion>
<groupId>javax.jms</groupId>
<artifactId>jms</artifactId>
</exclusion>
<exclusion>
<groupId>com.sun.jdmk</groupId>
<artifactId>jmxtools</artifactId>
</exclusion>
<exclusion>
<groupId>com.sun.jmx</groupId>
<artifactId>jmxri</artifactId>
</exclusion>
</exclusions>
<scope>runtime</scope>
</dependency>
<!-- @Inject -->
<dependency>
<groupId>javax.inject</groupId>
<artifactId>javax.inject</artifactId>
<version>1</version>
</dependency>
<!-- Servlet -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>servlet-api</artifactId>
<version>2.5</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>javax.servlet.jsp</groupId>
<artifactId>jsp-api</artifactId>
<version>2.1</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
<!-- Test -->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.7</version>
<scope>test</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/servlets.com/cos -->
<dependency>
<groupId>servlets.com</groupId>
<artifactId>cos</artifactId>
<version>05Nov2002</version>
</dependency>
<!-- https://mvnrepository.com/artifact/commons-io/commons-io -->
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.8.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/commons-fileupload/commons-fileupload -->
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.4</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.googlecode.json-simple/json-simple -->
<dependency>
<groupId>com.googlecode.json-simple</groupId>
<artifactId>json-simple</artifactId>
<version>1.1.1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.mariadb.jdbc/mariadb-java-client -->
<dependency>
<groupId>org.mariadb.jdbc</groupId>
<artifactId>mariadb-java-client</artifactId>
<version>2.7.1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.springframework/spring-jdbc -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>${org.springframework-version}</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<artifactId>maven-eclipse-plugin</artifactId>
<version>2.9</version>
<configuration>
<additionalProjectnatures>
<projectnature>org.springframework.ide.eclipse.core.springnature</projectnature>
</additionalProjectnatures>
<additionalBuildcommands>
<buildcommand>org.springframework.ide.eclipse.core.springbuilder</buildcommand>
</additionalBuildcommands>
<downloadSources>true</downloadSources>
<downloadJavadocs>true</downloadJavadocs>
</configuration>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>2.5.1</version>
<configuration>
<source>1.6</source>
<target>1.6</target>
<compilerArgument>-Xlint:all</compilerArgument>
<showWarnings>true</showWarnings>
<showDeprecation>true</showDeprecation>
</configuration>
</plugin>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>exec-maven-plugin</artifactId>
<version>1.2.1</version>
<configuration>
<mainClass>org.test.int1.Main</mainClass>
</configuration>
</plugin>
</plugins>
</build>
</project>
-web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.5" xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee https://java.sun.com/xml/ns/javaee/web-app_2_5.xsd">
<welcome-file-list>
<welcome-file>zipcode.do</welcome-file>
<welcome-file>zipcode.jsp</welcome-file>
</welcome-file-list>
<!-- The definition of the Root Spring Container shared by all Servlets and Filters -->
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/spring/root-context.xml</param-value>
</context-param>
<!-- Creates the Spring Container shared by all Servlets and Filters -->
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
<filter>
<filter-name>encodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>utf-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>encodingFilter</filter-name>
<url-pattern>*.do</url-pattern>
</filter-mapping>
<!-- Processes application requests -->
<servlet>
<servlet-name>appServlet</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/spring/appServlet/servlet-context.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>appServlet</servlet-name>
<url-pattern>*.do</url-pattern>
</servlet-mapping>
</web-app>
-root-context.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans https://www.springframework.org/schema/beans/spring-beans.xsd">
<!-- Root Context: defines shared resources visible to all other web components -->
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="org.mariadb.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3307/sample" />
<property name="username" value="root" />
<property name="password" value="!123456" />
</bean>
<!--
<bean id="jdbcTemplate" class="org.springframework.jdbc.core.JdbcTemplate">
<constructor-arg ref="dataSource" />
<property name="dataSource" ref="dataSource" />
</bean>
-->
</beans>
-servlet-context.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans:beans xmlns="http://www.springframework.org/schema/mvc"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:beans="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/mvc https://www.springframework.org/schema/mvc/spring-mvc.xsd
http://www.springframework.org/schema/beans https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd">
<!-- DispatcherServlet Context: defines this servlet's request-processing infrastructure -->
<!-- Enables the Spring MVC @Controller programming model -->
<annotation-driven />
<!-- Handles HTTP GET requests for /resources/** by efficiently serving up static resources in the ${webappRoot}/resources directory -->
<resources mapping="/resources/**" location="/resources/" />
<!-- Resolves views selected for rendering by @Controllers to .jsp resources in the /WEB-INF/views directory -->
<beans:bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<beans:property name="prefix" value="/WEB-INF/views/" />
<beans:property name="suffix" value=".jsp" />
</beans:bean>
<context:component-scan base-package="com.exam.web03" />
</beans:beans>
-views/zipcode.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<form action="zipcode_ok.do" method="post">
동이름 : <input type="text" name="dong" />
<input type="submit" value="동이름 검색" />
</form>
</body>
</html>
-views/zipcode_ok.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<%= request.getAttribute( "html" ) %>
</body>
</html>
-src/main/java/com.exam.web03.model1/ZipcodeTO.java
package com.exam.web03.model1;
public class ZipcodeTO {
private String zipcode;
private String sido;
private String gugun;
private String dong;
private String ri;
private String bunji;
public String getZipcode() {
return zipcode;
}
public String getSido() {
return sido;
}
public String getGugun() {
return gugun;
}
public String getDong() {
return dong;
}
public String getRi() {
return ri;
}
public String getBunji() {
return bunji;
}
public void setZipcode(String zipcode) {
this.zipcode = zipcode;
}
public void setSido(String sido) {
this.sido = sido;
}
public void setGugun(String gugun) {
this.gugun = gugun;
}
public void setDong(String dong) {
this.dong = dong;
}
public void setRi(String ri) {
this.ri = ri;
}
public void setBunji(String bunji) {
this.bunji = bunji;
}
}
-src/main/java/com.exam.web03.model1/ZipcodeDAO.java
package com.exam.web03.model1;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import javax.sql.DataSource;
import org.springframework.beans.factory.annotation.Autowired;
public class ZipcodeDAO {
private DataSource dataSource;
StringBuffer html = new StringBuffer();
public ZipcodeDAO( DataSource dataSource ) {
this.dataSource = dataSource;
}
public StringBuffer SearchDong( String strDong ) {
if ( strDong != null ) {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
conn = this.dataSource.getConnection();
String sql = "select zipcode, sido, gugun, dong, ri, bunji, seq from zipcode where dong like ?";
pstmt = conn.prepareStatement(sql);
pstmt.setString( 1, strDong + "%" );
rs = pstmt.executeQuery();
html.append( "<table border='1' width='900'>" );
while( rs.next() ) {
html.append( "<tr width='100'>" );
html.append( " <td>" + rs.getString( 1 ) + "<br>" + "</td>" );
html.append( " <td>" + rs.getString( 2 ) + "<br>" + "</td>" );
html.append( " <td>" + rs.getString( 3 ) + "<br>" + "</td>" );
html.append( " <td>" + rs.getString( 4 ) + "<br>" + "</td>" );
html.append( " <td>" + rs.getString( 5 ) + "<br>" + "</td>" );
html.append( " <td>" + rs.getString( 6 ) + "<br>" + "</td>" );
html.append( " <td>" + rs.getString( 7 ) + "<br>" + "</td>" );
html.append( "</tr>" );
}
html.append( "</table>" );
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
if( rs != null ) try { rs.close(); } catch( SQLException e) {}
if( pstmt != null ) try { pstmt.close(); } catch( SQLException e) {}
if( conn != null ) try { conn.close(); } catch( SQLException e) {}
}
}
return html;
}
}
-src/main/java/com.exam.web03/ZipcodeController.java
package com.exam.web03;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.sql.DataSource;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import com.exam.web03.model1.ZipcodeDAO;
@Controller
public class ZipcodeController {
@Autowired
private DataSource dataSource;
@RequestMapping( value="/zipcode.do" )
public String zipcode( HttpServletRequest request, HttpServletResponse response ) {
return "zipcode";
}
@RequestMapping( value="/zipcode_ok.do" )
public String zipcode_ok( HttpServletRequest request, HttpServletResponse response ) {
String strDong = request.getParameter( "dong" );
ZipcodeDAO dao = new ZipcodeDAO( dataSource );
String html = dao.SearchDong(strDong).toString();
request.setAttribute( "html", html );
return "zipcode_ok";
}
}
-index.jsp(실행jsp)
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
response.sendRedirect( "zipcode.do" );
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<ul>
<li><a href="zipcode.do">zipcode.do</a>
</ul>
</body>
</html>
2. JdbcTemplate 방식

-pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.exam</groupId>
<artifactId>web03</artifactId>
<name>SpringWebEx03</name>
<packaging>war</packaging>
<version>1.0.0-BUILD-SNAPSHOT</version>
<properties>
<java-version>1.8</java-version>
<org.springframework-version>5.2.8.RELEASE</org.springframework-version>
<org.aspectj-version>1.9.5</org.aspectj-version>
<org.slf4j-version>1.7.3</org.slf4j-version>
</properties>
<dependencies>
<!-- Spring -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>${org.springframework-version}</version>
<exclusions>
<!-- Exclude Commons Logging in favor of SLF4j -->
<exclusion>
<groupId>commons-logging</groupId>
<artifactId>commons-logging</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>${org.springframework-version}</version>
</dependency>
<!-- AspectJ -->
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjrt</artifactId>
<version>${org.aspectj-version}</version>
</dependency>
<!-- Logging -->
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>${org.slf4j-version}</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>jcl-over-slf4j</artifactId>
<version>${org.slf4j-version}</version>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-log4j12</artifactId>
<version>${org.slf4j-version}</version>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.15</version>
<exclusions>
<exclusion>
<groupId>javax.mail</groupId>
<artifactId>mail</artifactId>
</exclusion>
<exclusion>
<groupId>javax.jms</groupId>
<artifactId>jms</artifactId>
</exclusion>
<exclusion>
<groupId>com.sun.jdmk</groupId>
<artifactId>jmxtools</artifactId>
</exclusion>
<exclusion>
<groupId>com.sun.jmx</groupId>
<artifactId>jmxri</artifactId>
</exclusion>
</exclusions>
<scope>runtime</scope>
</dependency>
<!-- @Inject -->
<dependency>
<groupId>javax.inject</groupId>
<artifactId>javax.inject</artifactId>
<version>1</version>
</dependency>
<!-- Servlet -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>servlet-api</artifactId>
<version>2.5</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>javax.servlet.jsp</groupId>
<artifactId>jsp-api</artifactId>
<version>2.1</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
<!-- Test -->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.7</version>
<scope>test</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/servlets.com/cos -->
<dependency>
<groupId>servlets.com</groupId>
<artifactId>cos</artifactId>
<version>05Nov2002</version>
</dependency>
<!-- https://mvnrepository.com/artifact/commons-io/commons-io -->
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.8.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/commons-fileupload/commons-fileupload -->
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.4</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.googlecode.json-simple/json-simple -->
<dependency>
<groupId>com.googlecode.json-simple</groupId>
<artifactId>json-simple</artifactId>
<version>1.1.1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.mariadb.jdbc/mariadb-java-client -->
<dependency>
<groupId>org.mariadb.jdbc</groupId>
<artifactId>mariadb-java-client</artifactId>
<version>2.7.1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.springframework/spring-jdbc -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>${org.springframework-version}</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<artifactId>maven-eclipse-plugin</artifactId>
<version>2.9</version>
<configuration>
<additionalProjectnatures>
<projectnature>org.springframework.ide.eclipse.core.springnature</projectnature>
</additionalProjectnatures>
<additionalBuildcommands>
<buildcommand>org.springframework.ide.eclipse.core.springbuilder</buildcommand>
</additionalBuildcommands>
<downloadSources>true</downloadSources>
<downloadJavadocs>true</downloadJavadocs>
</configuration>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>2.5.1</version>
<configuration>
<source>1.6</source>
<target>1.6</target>
<compilerArgument>-Xlint:all</compilerArgument>
<showWarnings>true</showWarnings>
<showDeprecation>true</showDeprecation>
</configuration>
</plugin>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>exec-maven-plugin</artifactId>
<version>1.2.1</version>
<configuration>
<mainClass>org.test.int1.Main</mainClass>
</configuration>
</plugin>
</plugins>
</build>
</project>
-web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.5" xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee https://java.sun.com/xml/ns/javaee/web-app_2_5.xsd">
<welcome-file-list>
<welcome-file>zipcode.do</welcome-file>
<welcome-file>zipcode.jsp</welcome-file>
</welcome-file-list>
<!-- The definition of the Root Spring Container shared by all Servlets and Filters -->
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/spring/root-context.xml</param-value>
</context-param>
<!-- Creates the Spring Container shared by all Servlets and Filters -->
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
<filter>
<filter-name>encodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>utf-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>encodingFilter</filter-name>
<url-pattern>*.do</url-pattern>
</filter-mapping>
<!-- Processes application requests -->
<servlet>
<servlet-name>appServlet</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/spring/appServlet/servlet-context.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>appServlet</servlet-name>
<url-pattern>*.do</url-pattern>
</servlet-mapping>
</web-app>
-root-context.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans https://www.springframework.org/schema/beans/spring-beans.xsd">
<!-- Root Context: defines shared resources visible to all other web components -->
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="org.mariadb.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3307/sample" />
<property name="username" value="root" />
<property name="password" value="!123456" />
</bean>
<bean id="jdbcTemplate" class="org.springframework.jdbc.core.JdbcTemplate">
<constructor-arg ref="dataSource" />
<property name="dataSource" ref="dataSource" />
</bean>
</beans>
-servlet-context.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans:beans xmlns="http://www.springframework.org/schema/mvc"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:beans="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/mvc https://www.springframework.org/schema/mvc/spring-mvc.xsd
http://www.springframework.org/schema/beans https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd">
<!-- DispatcherServlet Context: defines this servlet's request-processing infrastructure -->
<!-- Enables the Spring MVC @Controller programming model -->
<annotation-driven />
<!-- Handles HTTP GET requests for /resources/** by efficiently serving up static resources in the ${webappRoot}/resources directory -->
<resources mapping="/resources/**" location="/resources/" />
<!-- Resolves views selected for rendering by @Controllers to .jsp resources in the /WEB-INF/views directory -->
<beans:bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<beans:property name="prefix" value="/WEB-INF/views/" />
<beans:property name="suffix" value=".jsp" />
</beans:bean>
<context:component-scan base-package="com.exam.web03" />
</beans:beans>
-views/zipcode.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<form action="zipcode_ok.do" method="post">
동이름 : <input type="text" name="dong" />
<input type="submit" value="동이름 검색" />
</form>
</body>
</html>
-views/zipcode_ok.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<%= request.getAttribute( "html" ) %>
</body>
</html>
-src/main/java/com.exam.jdbc01.model1/ZipcodeTO.java
package com.exam.web03.model1;
public class ZipcodeTO {
private String zipcode;
private String sido;
private String gugun;
private String dong;
private String ri;
private String bunji;
public String getZipcode() {
return zipcode;
}
public String getSido() {
return sido;
}
public String getGugun() {
return gugun;
}
public String getDong() {
return dong;
}
public String getRi() {
return ri;
}
public String getBunji() {
return bunji;
}
public void setZipcode(String zipcode) {
this.zipcode = zipcode;
}
public void setSido(String sido) {
this.sido = sido;
}
public void setGugun(String gugun) {
this.gugun = gugun;
}
public void setDong(String dong) {
this.dong = dong;
}
public void setRi(String ri) {
this.ri = ri;
}
public void setBunji(String bunji) {
this.bunji = bunji;
}
}
-src/main/java/com.exam.jdbc01.model1/ZipcodeDAO.java
package com.exam.jdbc01.model1;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import org.springframework.dao.DataAccessException;
import org.springframework.jdbc.core.BeanPropertyRowMapper;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.jdbc.core.PreparedStatementCreator;
import org.springframework.jdbc.core.ResultSetExtractor;
public class ZipcodeDAO {
private JdbcTemplate jdbcTemplate;
private StringBuffer html = new StringBuffer();
public ZipcodeDAO( JdbcTemplate jdbcTemplate ) {
this.jdbcTemplate = jdbcTemplate;
}
public StringBuffer SearchDong( String strDong ) {
if ( strDong != null ) {
String sql = "select zipcode, sido, gugun, dong, ri, bunji, seq from zipcode where dong like ?";
jdbcTemplate.query(new PreparedStatementCreator() {
@Override
public PreparedStatement createPreparedStatement(Connection con) throws SQLException {
PreparedStatement pstmt = null;
pstmt = con.prepareStatement(sql);
pstmt.setString(1, strDong + "%" );
return pstmt;
}
}, new ResultSetExtractor<StringBuffer>() {
@Override
public StringBuffer extractData(ResultSet rs) throws SQLException, DataAccessException {
html.append( "<table border='1' width='900'>" );
while( rs.next() ) {
html.append( "<tr width='100'>" );
html.append( " <td>" + rs.getString( 1 ) + "<br>" + "</td>" );
html.append( " <td>" + rs.getString( 2 ) + "<br>" + "</td>" );
html.append( " <td>" + rs.getString( 3 ) + "<br>" + "</td>" );
html.append( " <td>" + rs.getString( 4 ) + "<br>" + "</td>" );
html.append( " <td>" + rs.getString( 5 ) + "<br>" + "</td>" );
html.append( " <td>" + rs.getString( 6 ) + "<br>" + "</td>" );
html.append( " <td>" + rs.getString( 7 ) + "<br>" + "</td>" );
html.append( "</tr>" );
}
html.append( "</table>" );
return html;
}
});
/*
ArrayList<ZipcodeTO> lists =
(ArrayList<ZipcodeTO>)jdbcTemplate.query(
sql, new BeanPropertyRowMapper<ZipcodeTO>(ZipcodeTO.class), strDong+"%" );
html.append( "<table border='1' width='900'>" );
for( ZipcodeTO list : lists ) {
html.append( "<tr width='100'>" );
html.append( " <td>" + list.getZipcode() + "<br>" + "</td>" );
html.append( " <td>" + list.getSido() + "<br>" + "</td>" );
html.append( " <td>" + list.getGugun() + "<br>" + "</td>" );
html.append( " <td>" + list.getDong() + "<br>" + "</td>" );
html.append( " <td>" + list.getBunji() + "<br>" + "</td>" );
html.append( " <td>" + list.getRi() + "<br>" + "</td>" );
html.append( "</tr>" );
}
html.append( "</table>" );
*/
}
return html;
}
}
-src/main/java/com.exam.jdbc01/HomeController.java
package com.exam.jdbc01;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import com.exam.jdbc01.model1.ZipcodeDAO;
@Controller
public class HomeController {
@Autowired
private JdbcTemplate jdbcTemplate;
@RequestMapping(value = "zipcode.do")
public String zipcode( HttpServletRequest request, HttpServletResponse response ) {
return "zipcode";
}
@RequestMapping(value = "zipcode_ok.do")
public String zipcode_ok( HttpServletRequest request, HttpServletResponse response ) {
String strDong = request.getParameter( "dong" );
ZipcodeDAO dao = new ZipcodeDAO( jdbcTemplate );
String html = dao.SearchDong(strDong).toString();
request.setAttribute( "html", html );
return "zipcode_ok";
}
}
-index.jsp(실행jsp)
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
response.sendRedirect( "zipcode.do" );
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<ul>
<li><a href="zipcode.do">zipcode.do</a>
</ul>
</body>
</html>