일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 | 31 |
- 맛집
- 코딩테스트
- Kafka
- bigdata engineering
- 여행
- 프로그래머스
- 용인맛집
- Data Engineering
- BigData
- apache iceberg
- 영어
- Data Engineer
- bigdata engineer
- java
- 자바
- HIVE
- hadoop
- 코딩
- 알고리즘
- 코엑스맛집
- Trino
- Spark
- 백준
- 삼성역맛집
- Apache Kafka
- Linux
- pyspark
- 개발
- 코테
- Iceberg
- Today
- Total
지구정복
[JavaScript-jQuery, Ajax] 1/15 | jQuery UI 사용하기(DatePicker, Dialog, Menu, Tabs, Tab으로 구구단만들기, Tooltip), Model2 게시판 jQuery UI으로 구현하기 본문
[JavaScript-jQuery, Ajax] 1/15 | jQuery UI 사용하기(DatePicker, Dialog, Menu, Tabs, Tab으로 구구단만들기, Tooltip), Model2 게시판 jQuery UI으로 구현하기
noohhee 2021. 1. 15. 18:111. jQuery UI 사용하기(이어서)
ㅇDatePicker
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="./css/ui-darkness/jquery-ui.css" />
<style type="text/css">
body {font-size: 80% }
</style>
<script type="text/javascript" src="./js/jquery-3.5.1.js"></script>
<script type="text/javascript" src="./js/jquery-ui.js"></script>
<script type="text/javascript">
$(document).ready( function () {
$('#datepicker1').datepicker();
})
</script>
</head>
<body>
<div>
<!-- HTML5에서 제공하는 달력 -->
<input type="date"/>
</div>
<br><hr><br>
<div>
<input type="text" id="datepicker1" readonly="readonly"/>
</div>
</body>
</html>

위처럼 팝업되는 달력말고 아예 달력이 바로 보이도록 출력해보자.
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="./css/ui-darkness/jquery-ui.css" />
<style type="text/css">
body {font-size: 80% }
</style>
<script type="text/javascript" src="./js/jquery-3.5.1.js"></script>
<script type="text/javascript" src="./js/jquery-ui.js"></script>
<script type="text/javascript">
$(document).ready( function () {
$('#datepicker1').datepicker();
$('#datepicker2').datepicker();
})
</script>
</head>
<body>
<div>
<!-- HTML5에서 제공하는 달력 -->
<input type="date"/>
</div>
<br><hr><br>
<div>
<!-- 팝업방식 -->
<input type="text" id="datepicker1" readonly="readonly"/>
</div>
<br><hr><br>
<div id="datepicker2">
<!-- 인라인방식 -->
</div>
</body>
</html>

html5에서 제공하는 달력은 엣지브라우저에서 사용할 수 있고 브라우저가 옛 버전이면 실행되지 않는다.
날짜형식지정
이번에는 날짜 형식을 지정해보자.
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="./css/ui-darkness/jquery-ui.css" />
<style type="text/css">
body {font-size: 80% }
</style>
<script type="text/javascript" src="./js/jquery-3.5.1.js"></script>
<script type="text/javascript" src="./js/jquery-ui.js"></script>
<script type="text/javascript">
$(document).ready( function () {
$('#datepicker').datepicker();
$('#format').on('change', function() {
$('#datepicker').datepicker( 'option', 'dateFormat', $(this).val() );
})
})
</script>
</head>
<body>
<p>Date : <input type="text" id="datepicker" size="30"></p>
<p>Format options:<br>
<select id="format">
<option value="mm/dd/yy">Default - mm/dd/yy</option>
<option value="yy-mm-dd">ISO 8601 - yy-mm-dd</option>
<option value="d M, y">Short - d M, y</option>
<option value="d MM, y">Medium - d MM, y</option>
<option value="DD, d MM, yy">Full - DD, d MM, yy</option>
<option value="'day' d 'of' MM 'in the year' yy">With text - 'day' d 'of' MM 'in the year' yy</option>
</select>
</p>
</body>
</html>

애니메이션
애니메이션 효과를 추가해보자. bounce효과를 추가해본다.
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="./css/ui-darkness/jquery-ui.css" />
<style type="text/css">
body {font-size: 80% }
</style>
<script type="text/javascript" src="./js/jquery-3.5.1.js"></script>
<script type="text/javascript" src="./js/jquery-ui.js"></script>
<script type="text/javascript">
$(document).ready( function () {
$('#datepicker').datepicker({
dataFormat: 'yy-mm-dd',
showAnim: 'bounce'
});
$('#format').on('change', function() {
$('#datepicker').datepicker( 'option', 'dateFormat', $(this).val() );
})
})
</script>
</head>
<body>
<p>Date : <input type="text" id="datepicker" size="30"></p>
<p>Format options:<br>
<select id="format">
<option value="mm/dd/yy">Default - mm/dd/yy</option>
<option value="yy-mm-dd">ISO 8601 - yy-mm-dd</option>
<option value="d M, y">Short - d M, y</option>
<option value="d MM, y">Medium - d MM, y</option>
<option value="DD, d MM, yy">Full - DD, d MM, yy</option>
<option value="'day' d 'of' MM 'in the year' yy">With text - 'day' d 'of' MM 'in the year' yy</option>
</select>
</p>
</body>
</html>

여러달 한꺼번에 출력
이번에는 여러 달이 한꺼번에 나올 수 있도록 만들어보자.
속성값은 numberOfMonths 이다. 또한 버튼을 추가할 수 있다.
$('#datepicker').datepicker({
numberOfMonths: 3,
dataFormat: 'yy-mm-dd',
showAnim: 'bounce',
showButtonPanel: 'true'
});

ㅇDialog
창을 띄울 수 있다.
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="./css/ui-darkness/jquery-ui.css" />
<style type="text/css">
body {font-size: 80% }
</style>
<script type="text/javascript" src="./js/jquery-3.5.1.js"></script>
<script type="text/javascript" src="./js/jquery-ui.js"></script>
<script type="text/javascript">
$(document).ready( function () {
$('#btn1').button().on('click', function() {
//window.open
//open( url, 창이름, 속성값들 );
open( 'https://m.daum.net', 'winopen', 'width=640, height=960');
})
})
</script>
</head>
<body>
<button id="btn1">창열기</button>
<br><hr>
</body>
</html>

이번에는 직접 창을 만들어서 띄워보자.
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="./css/ui-darkness/jquery-ui.css" />
<style type="text/css">
body {font-size: 80% }
</style>
<script type="text/javascript" src="./js/jquery-3.5.1.js"></script>
<script type="text/javascript" src="./js/jquery-ui.js"></script>
<script type="text/javascript">
$(document).ready( function () {
$('#btn1').button().on('click', function() {
//window.open
//open( url, 창이름, 속성값들 );
open( 'https://m.daum.net', 'winopen', 'width=640, height=960');
})
$('#dialog').dialog({
width: 500,
height: 300,
autoOpen: false, //브라우저 킬 때 자동실행을 끈다.
modal: true, //창 열면 뒷 배경이 어두워지고 클릭이 안되는 기능
resizable: false
});
$('#btn2').button().on('click', function() {
$('#dialog').dialog( 'open' );
})
})
</script>
</head>
<body>
<button id="btn1">창열기1</button>
<button id="btn2">창열기2</button>
<br><hr>
<div id="dialog" title="jQuery Dialog">
<h1>Hello jQuery</h1>
</div>
</body>
</html>

창에 버튼추가
창 안에 버튼을 추가해보자.
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="./css/ui-darkness/jquery-ui.css" />
<style type="text/css">
body {font-size: 80% }
</style>
<script type="text/javascript" src="./js/jquery-3.5.1.js"></script>
<script type="text/javascript" src="./js/jquery-ui.js"></script>
<script type="text/javascript">
$(document).ready( function () {
$('#btn1').button().on('click', function() {
//window.open
//open( url, 창이름, 속성값들 );
open( 'https://m.daum.net', 'winopen', 'width=640, height=960');
})
$('#dialog').dialog({
width: 500,
height: 300,
autoOpen: false, //브라우저 킬 때 자동실행을 끈다.
modal: true, //창 열면 뒷 배경이 어두워지고 클릭이 안되는 기능
resizable: false,
buttons:{
'취소': function() {
alert( '취소' );
$(this).dialog( 'close' );
},
'확인': function() {
alert( '확인' );
$(this).dialog( 'close' );
}
}
});
$('#btn2').button().on('click', function() {
$('#dialog').dialog( 'open' );
})
})
</script>
</head>
<body>
<button id="btn1">창열기1</button>
<button id="btn2">창열기2</button>
<br><hr>
<div id="dialog" title="jQuery Dialog">
<h1>Hello jQuery</h1>
<h1>Hello jQuery</h1>
<h1>Hello jQuery</h1>
</div>
</body>
</html>

애니메이션 효과
또한 창이 열릴 때와 닫힐 때 애니메이션 효과를 줄 수 있다.
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="./css/ui-darkness/jquery-ui.css" />
<style type="text/css">
body {font-size: 80% }
</style>
<script type="text/javascript" src="./js/jquery-3.5.1.js"></script>
<script type="text/javascript" src="./js/jquery-ui.js"></script>
<script type="text/javascript">
$(document).ready( function () {
$('#btn1').button().on('click', function() {
//window.open
//open( url, 창이름, 속성값들 );
open( 'https://m.daum.net', 'winopen', 'width=640, height=960');
})
$('#dialog').dialog({
width: 500,
height: 300,
autoOpen: false, //브라우저 킬 때 자동실행을 끈다.
modal: true, //창 열면 뒷 배경이 어두워지고 클릭이 안되는 기능
resizable: false,
buttons:{
'취소': function() {
alert( '취소' );
$(this).dialog( 'close' );
},
'확인': function() {
alert( '확인' );
$(this).dialog( 'close' );
}
},
show: {
effect: 'blind',
duration: 1000
},
hide: {
effect: 'explode',
duration: 1000
}
});
$('#btn2').button().on('click', function() {
$('#dialog').dialog( 'open' );
})
})
</script>
</head>
<body>
<button id="btn1">창열기1</button>
<button id="btn2">창열기2</button>
<br><hr>
<div id="dialog" title="jQuery Dialog">
<h1>Hello jQuery</h1>
<h1>Hello jQuery</h1>
<h1>Hello jQuery</h1>
</div>
</body>
</html>

이번에는 modal 속성을 없애고 창닫기 버튼을 만들어서 열린 dialog창을 닫아보자.
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="./css/ui-darkness/jquery-ui.css" />
<style type="text/css">
body {font-size: 80% }
</style>
<script type="text/javascript" src="./js/jquery-3.5.1.js"></script>
<script type="text/javascript" src="./js/jquery-ui.js"></script>
<script type="text/javascript">
$(document).ready( function () {
$('#btn1').button().on('click', function() {
//window.open
//open( url, 창이름, 속성값들 );
open( 'https://m.daum.net', 'winopen', 'width=640, height=960');
})
$('#dialog').dialog({
width: 500,
height: 300,
autoOpen: false, //브라우저 킬 때 자동실행을 끈다.
//modal: true, //창 열면 뒷 배경이 어두워지고 클릭이 안되는 기능
resizable: false,
buttons:{
'취소': function() {
alert( '취소' );
$(this).dialog( 'close' );
},
'확인': function() {
alert( '확인' );
$(this).dialog( 'close' );
}
},
show: {
effect: 'blind',
duration: 1000
},
hide: {
effect: 'explode',
duration: 1000
}
});
$('#btn2').button().on('click', function() {
$('#dialog').dialog( 'open' );
})
$('#btn3').button().on('click', function() {
$('#dialog').dialog( 'close' );
})
})
</script>
</head>
<body>
<button id="btn1">창열기1</button>
<button id="btn2">창열기2</button>
<button id="btn3">창닫기2</button>
<br><hr>
<div id="dialog" title="jQuery Dialog">
<h1>Hello jQuery</h1>
<h1>Hello jQuery</h1>
<h1>Hello jQuery</h1>
</div>
</body>
</html>

다이얼로그로 유저목록창 만들기
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="./css/ui-darkness/jquery-ui.css" />
<style type="text/css">
body {font-size: 80% }
label, input { display:block; }
input.text { margin-bottom:12px; width:95%; padding: .4em; }
fieldset { padding:0; border:0; margin-top:25px; }
h1 { font-size: 1.2em; margin: .6em 0; }
div#users-contain { width: 350px; margin: 20px 0; }
div#users-contain table { margin: 1em 0; border-collapse: collapse; width: 100%; }
div#users-contain table td, div#users-contain table th { border: 1px solid #eee; padding: .6em 10px; text-align: left; }
.ui-dialog .ui-state-error { padding: .3em; }
.validateTips { border: 1px solid transparent; padding: 0.3em; }
</style>
<script type="text/javascript" src="./js/jquery-3.5.1.js"></script>
<script type="text/javascript" src="./js/jquery-ui.js"></script>
<script type="text/javascript">
$(document).ready( function () {
let i = 0;
$('#dialog-form').dialog({
autoOpen: false,
modal: true,
width: 400,
height: 400,
buttons: {
'취소': function() {
$(this).dialog('close');
},
'추가': function() {
let html = '<tr id="tr'+i+'">';
html += ' <td>' + $('#name').val() + '</td>';
html += ' <td>' + $('#email').val() + '</td>';
html += ' <td>' + $('#password').val() + '</td>';
html += ' <td>';
html += ' <button index="'+ ++i +'">삭제</button>';
html += ' </td>';
html += '</tr>';
$('#users tbody').append( html );
$('button[index="'+i+'"]' ).button().on('click', function() {
let a = $(this).attr( 'index')
console.log( a );
$(this).parent().parent().remove();
})
$(this).dialog('close');
}
}
})
$('#create-user').button().on('click', function() {
$('#dialog-form').dialog('open');
})
//
});
</script>
</head>
<body>
<div id="users-contain" class="ui-widget">
<h1>Existing Users:</h1>
<table id="users" class="ui-widget ui-widget-content">
<thead>
<tr class="ui-widget-header ">
<th>Name</th>
<th>Email</th>
<th>Password</th>
<th>Button</th>
</tr>
</thead>
<tbody>
</tbody>
</table>
</div>
<button id="create-user">Create new user</button>
<div id="dialog-form" title="Create new user">
<p class="validateTips">All form fields are required.</p>
<form>
<fieldset>
<label for="name">Name</label>
<input type="text" name="name" id="name" value="" class="text ui-widget-content ui-corner-all">
<label for="email">Email</label>
<input type="text" name="email" id="email" value="" class="text ui-widget-content ui-corner-all">
<label for="password">Password</label>
<input type="password" name="password" id="password" value="" class="text ui-widget-content ui-corner-all">
<!-- Allow form submission with keyboard without duplicating the dialog button -->
<input type="submit" tabindex="-1" style="position:absolute; top:-1000px">
</fieldset>
</form>
</div>
</body>
</html>
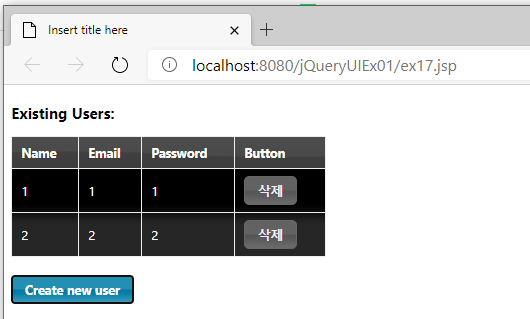
ㅇMenu
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="./css/ui-darkness/jquery-ui.css" />
<style type="text/css">
body {font-size: 80% }
.ui-menu { width: 200px }
</style>
<script type="text/javascript" src="./js/jquery-3.5.1.js"></script>
<script type="text/javascript" src="./js/jquery-ui.js"></script>
<script type="text/javascript">
$(document).ready( function () {
$('#menu').menu();
//
});
</script>
</head>
<body>
<ul id="menu">
<li><div class="ui-state-disabled">Toys (n/a)</div></li>
<li><div>Books</div></li>
<li><div>Clothing</div></li>
<li><div>Electronics</div>
<ul>
<li><div>Home Entertainment</div></li>
<li><div>Car Hifi</div></li>
<li><div>Utilities</div></li>
</ul>
</li>
<li><div>Movies</div></li>
<li><div>Music</div>
<ul>
<li><div>Rock</div>
<ul>
<li><div>Alternative</div></li>
<li><div>Classic</div></li>
</ul>
</li>
<li><div>Jazz</div>
<ul>
<li><div>Freejazz</div></li>
<li><div>Big Band</div></li>
<li><div>Modern</div></li>
</ul>
</li>
<li><div>Pop</div></li>
</ul>
</li>
<li><div class="ui-state-disabled">Specials (n/a)</div></li>
</ul>
</body>
</html>

메뉴 아이콘 추가하기
이번엔 메뉴에 아이콘을 추가해보자.
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="./css/ui-darkness/jquery-ui.css" />
<style type="text/css">
body {font-size: 80% }
.ui-menu { width: 150px; }
</style>
<script type="text/javascript" src="./js/jquery-3.5.1.js"></script>
<script type="text/javascript" src="./js/jquery-ui.js"></script>
<script type="text/javascript">
$(document).ready( function () {
$( "#menu" ).menu();
//
});
</script>
</head>
<body>
<ul id="menu">
<li>
<div><span class="ui-icon ui-icon-disk"></span>Save</div>
</li>
<li>
<div><span class="ui-icon ui-icon-zoomin"></span>Zoom In</div>
</li>
<li>
<div><span class="ui-icon ui-icon-zoomout"></span>Zoom Out</div>
</li>
<li class="ui-state-disabled">
<div><span class="ui-icon ui-icon-print"></span>Print...</div>
</li>
<li>
<div>Playback</div>
<ul>
<li>
<div><span class="ui-icon ui-icon-seek-start"></span>Prev</div>
</li>
<li>
<div><span class="ui-icon ui-icon-stop"></span>Stop</div>
</li>
<li>
<div><span class="ui-icon ui-icon-play"></span>Play</div>
</li>
<li>
<div><span class="ui-icon ui-icon-seek-end"></span>Next</div>
</li>
</ul>
</li>
<li>
<div>Learn more about this menu</div>
</li>
</ul>
</body>
</html>

ㅇTabs
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="./css/ui-darkness/jquery-ui.css" />
<style type="text/css">
body {font-size: 80% }
.ui-tabs { width: 80% }
</style>
<script type="text/javascript" src="./js/jquery-3.5.1.js"></script>
<script type="text/javascript" src="./js/jquery-ui.js"></script>
<script type="text/javascript">
$(document).ready( function () {
$('#tabs').tabs();
//
});
</script>
</head>
<body>
<div id="tabs">
<ul>
<li><a href="#tabs-1">Nunc tincidunt</a></li>
<li><a href="#tabs-2">Proin dolor</a></li>
<li><a href="#tabs-3">Aenean lacinia</a></li>
</ul>
<div id="tabs-1">
<p>Proin elit arcu, rutrum commodo, vehicula tempus, commodo a, risus. Curabitur nec arcu. Donec sollicitudin mi sit amet mauris. Nam elementum quam ullamcorper ante. Etiam aliquet massa et lorem. Mauris dapibus lacus auctor risus. Aenean tempor ullamcorper leo. Vivamus sed magna quis ligula eleifend adipiscing. Duis orci. Aliquam sodales tortor vitae ipsum. Aliquam nulla. Duis aliquam molestie erat. Ut et mauris vel pede varius sollicitudin. Sed ut dolor nec orci tincidunt interdum. Phasellus ipsum. Nunc tristique tempus lectus.</p>
</div>
<div id="tabs-2">
<p>Morbi tincidunt, dui sit amet facilisis feugiat, odio metus gravida ante, ut pharetra massa metus id nunc. Duis scelerisque molestie turpis. Sed fringilla, massa eget luctus malesuada, metus eros molestie lectus, ut tempus eros massa ut dolor. Aenean aliquet fringilla sem. Suspendisse sed ligula in ligula suscipit aliquam. Praesent in eros vestibulum mi adipiscing adipiscing. Morbi facilisis. Curabitur ornare consequat nunc. Aenean vel metus. Ut posuere viverra nulla. Aliquam erat volutpat. Pellentesque convallis. Maecenas feugiat, tellus pellentesque pretium posuere, felis lorem euismod felis, eu ornare leo nisi vel felis. Mauris consectetur tortor et purus.</p>
</div>
<div id="tabs-3">
<p>Mauris eleifend est et turpis. Duis id erat. Suspendisse potenti. Aliquam vulputate, pede vel vehicula accumsan, mi neque rutrum erat, eu congue orci lorem eget lorem. Vestibulum non ante. Class aptent taciti sociosqu ad litora torquent per conubia nostra, per inceptos himenaeos. Fusce sodales. Quisque eu urna vel enim commodo pellentesque. Praesent eu risus hendrerit ligula tempus pretium. Curabitur lorem enim, pretium nec, feugiat nec, luctus a, lacus.</p>
<p>Duis cursus. Maecenas ligula eros, blandit nec, pharetra at, semper at, magna. Nullam ac lacus. Nulla facilisi. Praesent viverra justo vitae neque. Praesent blandit adipiscing velit. Suspendisse potenti. Donec mattis, pede vel pharetra blandit, magna ligula faucibus eros, id euismod lacus dolor eget odio. Nam scelerisque. Donec non libero sed nulla mattis commodo. Ut sagittis. Donec nisi lectus, feugiat porttitor, tempor ac, tempor vitae, pede. Aenean vehicula velit eu tellus interdum rutrum. Maecenas commodo. Pellentesque nec elit. Fusce in lacus. Vivamus a libero vitae lectus hendrerit hendrerit.</p>
</div>
</div>
</body>
</html>

탭추가하기
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="./css/ui-darkness/jquery-ui.css" />
<style type="text/css">
body {font-size: 80% }
.ui-tabs { width: 80% }
</style>
<script type="text/javascript" src="./js/jquery-3.5.1.js"></script>
<script type="text/javascript" src="./js/jquery-ui.js"></script>
<script type="text/javascript">
$(document).ready( function () {
$('#tabs').tabs();
$('#btn').button().on('click', function() {
// li에 추가
$('#tabs').tabs().find('.ui-tabs-nav').append('<li><a href="#tabs-4">etc</a></li>');
// div에 추가
$('#tabs').tabs().append('<div id="tabs-4"><p>내용구문</p></div>');
$('#tabs').tabs('refresh');
})
//
});
</script>
</head>
<body>
<button id="btn">추가</button>
<br><hr>
<div id="tabs">
<ul>
<li><a href="#tabs-1">Nunc tincidunt</a></li>
<li><a href="#tabs-2">Proin dolor</a></li>
<li><a href="#tabs-3">Aenean lacinia</a></li>
</ul>
<div id="tabs-1">
<p>Proin elit arcu, rutrum commodo, vehicula tempus, commodo a, risus. Curabitur nec arcu. Donec sollicitudin mi sit amet mauris. Nam elementum quam ullamcorper ante. Etiam aliquet massa et lorem. Mauris dapibus lacus auctor risus. Aenean tempor ullamcorper leo. Vivamus sed magna quis ligula eleifend adipiscing. Duis orci. Aliquam sodales tortor vitae ipsum. Aliquam nulla. Duis aliquam molestie erat. Ut et mauris vel pede varius sollicitudin. Sed ut dolor nec orci tincidunt interdum. Phasellus ipsum. Nunc tristique tempus lectus.</p>
</div>
<div id="tabs-2">
<p>Morbi tincidunt, dui sit amet facilisis feugiat, odio metus gravida ante, ut pharetra massa metus id nunc. Duis scelerisque molestie turpis. Sed fringilla, massa eget luctus malesuada, metus eros molestie lectus, ut tempus eros massa ut dolor. Aenean aliquet fringilla sem. Suspendisse sed ligula in ligula suscipit aliquam. Praesent in eros vestibulum mi adipiscing adipiscing. Morbi facilisis. Curabitur ornare consequat nunc. Aenean vel metus. Ut posuere viverra nulla. Aliquam erat volutpat. Pellentesque convallis. Maecenas feugiat, tellus pellentesque pretium posuere, felis lorem euismod felis, eu ornare leo nisi vel felis. Mauris consectetur tortor et purus.</p>
</div>
<div id="tabs-3">
<p>Mauris eleifend est et turpis. Duis id erat. Suspendisse potenti. Aliquam vulputate, pede vel vehicula accumsan, mi neque rutrum erat, eu congue orci lorem eget lorem. Vestibulum non ante. Class aptent taciti sociosqu ad litora torquent per conubia nostra, per inceptos himenaeos. Fusce sodales. Quisque eu urna vel enim commodo pellentesque. Praesent eu risus hendrerit ligula tempus pretium. Curabitur lorem enim, pretium nec, feugiat nec, luctus a, lacus.</p>
<p>Duis cursus. Maecenas ligula eros, blandit nec, pharetra at, semper at, magna. Nullam ac lacus. Nulla facilisi. Praesent viverra justo vitae neque. Praesent blandit adipiscing velit. Suspendisse potenti. Donec mattis, pede vel pharetra blandit, magna ligula faucibus eros, id euismod lacus dolor eget odio. Nam scelerisque. Donec non libero sed nulla mattis commodo. Ut sagittis. Donec nisi lectus, feugiat porttitor, tempor ac, tempor vitae, pede. Aenean vehicula velit eu tellus interdum rutrum. Maecenas commodo. Pellentesque nec elit. Fusce in lacus. Vivamus a libero vitae lectus hendrerit hendrerit.</p>
</div>
</div>
</body>
</html>

실습) 탭으로 구구단 만들기
-내코드 (전체단 한꺼번에 출력)
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="./css/ui-darkness/jquery-ui.css" />
<style type="text/css">
body {font-size: 80% }
.ui-tabs { width: 80% }
</style>
<script type="text/javascript" src="./js/jquery-3.5.1.js"></script>
<script type="text/javascript" src="./js/jquery-ui.js"></script>
<script type="text/javascript">
$(document).ready( function () {
let html = '<ul>';
for( let i=1; i<=9; i++ ) {
html += ' <li><a href="#t-'+ i +'">'+ i + '단' + '</a></li>';
}
html += '</ul>';
for( let i=1; i<=9; i++ ) {
html += '<div id="t-'+ i +'">';
for( let j=1; j<=9; j++ ) {
html += ' <p>'+ i + ' X ' + j + ' = ' + (i*j) +'</p>';
}
html += '</div>';
}
$('#tabs').html( html );
$('#tabs').tabs();
//
});
</script>
</head>
<body>
<div id="tabs"></div>
</body>
</html>

-강사님 코드 (버튼 클릭하면 단이 생기는 코드)
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="./css/ui-darkness/jquery-ui.css" />
<style type="text/css">
body { font-size: 80%; }
.ui-tabs { width: 80%; }
</style>
<script type="text/javascript" src="./js/jquery-3.5.1.js"></script>
<script type="text/javascript" src="./js/jquery-ui.js"></script>
<script type="text/javascript">
$( document ).ready( function() {
$( '#btn1' ).button().on( 'click', function() {
let dan = 1;
$( '#tabs' ).tabs().find( '.ui-tabs-nav' )
.append( '<li><a href="#tabs-' + dan + '">' + dan + ' 단</a></li>' );
let html = '<div id="tabs-' + dan + '"><p>';
for( let i=1 ; i<=9 ; i++ ) {
html += i + ' X ' + dan + ' = ' + (i*dan) + '<br />';
}
html += '</p></div>';
$( '#tabs' ).tabs().append( html );
$( '#tabs' ).tabs( 'refresh' );
});
});
</script>
</head>
<body>
<button id="btn1">1단 보이기</button>
<br /><hr /><br />
<div id="tabs">
<ul>
</ul>
</div>
</body>
</html>

ㅇTooltip
텍스트상자에 마우스 갖다대면 설명이 뜨는 것을 말한다.
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="./css/ui-darkness/jquery-ui.css" />
<style type="text/css">
body {font-size: 80% }
label {
display: inline-block;
width: 5em;
}
</style>
<script type="text/javascript" src="./js/jquery-3.5.1.js"></script>
<script type="text/javascript" src="./js/jquery-ui.js"></script>
<script type="text/javascript">
$(document).ready( function () {
//$( document ).tooltip();
$('p').tooltip();
//
});
</script>
</head>
<body>
<p><a href="#" title="That's what this widget is">Tooltips</a> can be attached to any element. When you hover
the element with your mouse, the title attribute is displayed in a little box next to the element, just like a native tooltip.</p>
<p>But as it's not a native tooltip, it can be styled. Any themes built with
<a href="http://jqueryui.com/themeroller/" title="ThemeRoller: jQuery UI's theme builder application">ThemeRoller</a>
will also style tooltips accordingly.</p>
<p>Tooltips are also useful for form elements, to show some additional information in the context of each field.</p>
<p><label for="age">Your age:</label><input id="age" title="We ask for your age only for statistical purposes."></p>
<p>Hover the field to see the tooltip.</p>
</body>
</html>

도움말나오게하기
이번엔 각 텍스트상자마다 모두 도움말이 나오도록 만들어보자.
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="./css/ui-darkness/jquery-ui.css" />
<style type="text/css">
body {font-size: 80% }
label {
display: inline-block; width: 5em;
}
fieldset div {
margin-bottom: 2em;
}
fieldset .help {
display: inline-block;
}
.ui-tooltip {
width: 210px;
}
</style>
<script type="text/javascript" src="./js/jquery-3.5.1.js"></script>
<script type="text/javascript" src="./js/jquery-ui.js"></script>
<script type="text/javascript">
$( document ).ready( function() {
var tooltips = $( '[title]' ).tooltip({
position: {
my: 'left top',
at: 'right+5 top-5',
collision: 'none'
}
})
$( '<button>' )
.text( 'Show help' )
.button()
.on( 'click', function() {
tooltips.tooltip( 'open' );
})
.insertAfter( 'form' );
//
});
</script>
</head>
<body>
<form>
<fieldset>
<div>
<label for="firstname">Firstname</label>
<input id="firstname" name="firstname" title="Please provide your firstname.">
</div>
<div>
<label for="lastname">Lastname</label>
<input id="lastname" name="lastname" title="Please provide your lastname.">
</div>
<div>
<label for="address">Address</label>
<input id="address" name="address" title="Please provide your address.">
</div>
</fieldset>
</form>
</body>
</html>

2. Model2 게시판 jQuery UI으로 구현하기
먼저 기존 웹 프로젝트인 Model2Ex03을 불러온다.
그리고 jQuery UI에서 사용한 js폴더와 css폴더를 모두 복사해온다.

그리고 웹콘텐츠에 board_ui.jsp를 만들고 아래의 내용을 작성한다.
-board_ui.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="./css/cupertino/jquery-ui.css">
<style type="text/css">
body { font-size: 70%; }
#accordion > div > div:last-child { text-align: right; }
#accordion-resizer {
margin: 0 60px;
max-width: 1500px;
}
#btngroup {
text-align: right;
}
#btngroup button {
margin: 3px;
padding: 3px;
width: 100px;
}
label.header {
font-size: 10pt;
margin-right: 5px;
}
input.text {
width: 80%;
margin-bottom: 12px;
padding: 0.4em;
}
fieldset {
margin-left: 15px;
margin-top: 15px;
border: 0;
}
</style>
<script type="text/javascript" src="./js/jquery-3.5.1.js"></script>
<script type="text/javascript" src="./js/jquery-ui.js"></script>
<script type="text/javascript">
$( document ).ready( function() {
$( '#writeDialog' ).css( 'display', 'none' );
$( '#modifyDialog' ).css( 'display', 'none' );
$( '#deleteDialog' ).css( 'display', 'none' );
var readServer = function() {
$( '#accordion' ).accordion({
collapsible: 'true',
heightStyle: 'content'
});
$( 'button.action' ).button();
};
readServer();
$( document ).on( 'click', 'button.action', function() {
if( $( this).attr( 'action' ) == 'write' ) {
$( '#writeDialog' ).dialog ({
width: 700,
height: 500,
modal: true,
buttons: {
'글쓰기': function() {
$( this ).dialog( 'close' );
},
'취소': function() {
$( this ).dialog( 'close' );
}
},
close: function() {
}
});
} else if( $( this ).attr( 'action' ) == 'modify' ) {
$( '#modifyDialog' ).dialog({
width: 700,
height: 500,
modal: true,
buttons: {
'수정': function() {
$( this ).dialog( 'close' );
},
'취소': function() {
$( this ).dialog( 'close' );
}
},
close: function() {
}
});
} else if( $( this ).attr( 'action' ) == 'delete' ) {
$( '#deleteDialog' ).dialog({
width: 700,
height: 200,
modal: true,
buttons: {
'삭제': function() {
$( this ).dialog( 'close' );
},
'취소': function() {
$( this ).dialog( 'close' );
}
},
close: function() {
}
});
} else {
}
});
});
</script>
</head>
<body>
<div id="accordion-resizer">
<hr noshade="noshade" />
<div id="accordion">
<h3>1 : 글쓴이(0) new</h3>
<div>
<div>2016-02-01</div>
<div>제목 1</div>
<br />
<hr noshade="noshade" />
<div>내용 1</div>
<br />
<hr noshade="noshade" />
<br />
<div>
<button idx="1" action="modify" class="action">수정</button>
<button idx="1" action="delete" class="action">삭제</button>
</div>
</div>
<h3>2 : 글쓴이(0) new</h3>
<div>
<div>2016-02-01</div>
<div>제목 2</div>
<br />
<hr noshade="noshade" />
<div>내용 2</div>
<br />
<hr noshade="noshade" />
<br />
<div>
<button idx="2" action="modify" class="action">수정</button>
<button idx="2" action="delete" class="action">삭제</button>
</div>
</div>
</div>
<hr noshade="noshade" />
<div id="btngroup">
<button action="write" class="action">글쓰기</button>
</div>
</div>
<div id="writeDialog" title="글쓰기">
<fieldset>
<div>
<label for="subject" class="header">제 목</label>
<input type="text" id="w_subject" class="text ui-widget-content ui-corner-all"/>
</div>
<div>
<label for="writer" class="header">이 름</label>
<input type="text" id="w_writer" class="text ui-widget-content ui-corner-all"/>
</div>
<div>
<label for="mail" class="header">메 일</label>
<input type="text" id="w_mail" class="text ui-widget-content ui-corner-all"/>
</div>
<div>
<label for="password" class="header">비밀 번호</label>
<input type="password" id="w_password" class="text ui-widget-content ui-corner-all"/>
</div>
<div>
<label for="content" class="header">본 문</label>
<br /><br />
<textarea rows="15" cols="100" id="w_content" class="text ui-widget-content ui-corner-all"></textarea>
</div>
</fieldset>
</div>
<div id="modifyDialog" title="글수정">
<fieldset>
<div>
<label for="subject" class="header">제 목</label>
<input type="text" id="m_subject" class="text ui-widget-content ui-corner-all"/>
</div>
<div>
<label for="writer" class="header">이 름</label>
<input type="text" id="m_writer" class="text ui-widget-content ui-corner-all" readonly="readonly"/>
</div>
<div>
<label for="mail" class="header">메 일</label>
<input type="text" id="m_mail" class="text ui-widget-content ui-corner-all"/>
</div>
<div>
<label for="password" class="header">비밀 번호</label>
<input type="password" id="m_password" class="text ui-widget-content ui-corner-all"/>
</div>
<div>
<label for="content" class="header">본 문</label>
<br /><br />
<textarea rows="15" cols="100" id="m_content" class="text ui-widget-content ui-corner-all"></textarea>
</div>
</fieldset>
</div>
<div id="deleteDialog" title="글삭제">
<fieldset>
<div>
<label for="subject" class="header">제 목</label>
<input type="text" id="d_subject" class="text ui-widget-content ui-corner-all" readonly="readonly"/>
</div>
<div>
<label for="password" class="header">비밀 번호</label>
<input type="password" id="d_password" class="text ui-widget-content ui-corner-all"/>
</div>
</fieldset>
</div>
</body>
</html>
위 디자인을 실행시키면 jQueryUI가 적용된 게시판이 보이고 이를 구현하려면 웹2.0으로 해야되기때문에
Ajax를 이용해야 한다.
아래는 게시판 기능별 데이터 프로토콜이다.
-데이터 프로토콜
페이지 전송내용 처리페이지
ㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡ
write_ok -> 게시판내용 -> board_write1_ok.jsp
modify_ok { board_modify1_ok.jsp
delete_ok <- "flag": "" <- board_delete1_ok.jsp
}
// ~_ok에서 데이터를 넘겨주면 처리페이지에서 flag값을 리턴해준다.
view -> seq <- board_view1.jsp
modify { board_modify1.jsp
delete "flag: "", board_delete1.jsp
"data":{
"subject":"",
"writer":"",
....
}
}
// 그냥 view, modify, delete에서 seq를 넘겨주면 처리페이지에서는 flag와 데이터값을 리턴해준다.
list -> { -> board_list1.jsp
<- "flag: "", <-
"data":{
"subject":"",
"writer":"",
....
}
}
그리고 WEB-INF에 json이란 폴더를 만들고 아래와 같이 views 파일을 모두 넣어준다.

그리고 controllerEx01.java파일을 아래와 같이 수정한다.
-controllerEx01.java
package servlet;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import model2.BoardAction;
import model2.DeleteAction;
import model2.DeleteOkAction;
import model2.ListAction;
import model2.ModifyAction;
import model2.ModifyOkAction;
import model2.ViewAction;
import model2.WriteAction;
import model2.WriteOkAction;
/**
* Servlet implementation class ControllerEx01
*/
@WebServlet( { "*.do", "*.json" } )
public class controllerEx01 extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
this.doProcess(request, response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
this.doProcess(request, response);
}
protected void doProcess(HttpServletRequest request, HttpServletResponse response) {
try {
request.setCharacterEncoding( "utf-8" );
String action= request.getRequestURI().replaceAll( request.getContextPath(), "" );
String url = "";
BoardAction boardAction = null;
if ( action == null || action.equals("/*.do") || action.equals( "/list.do" ) ) {
boardAction = new ListAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_list1.jsp";
} else if ( action.equals( "/view.do" ) ) {
boardAction = new ViewAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_view1.jsp";
} else if ( action.equals( "/write.do" ) ) {
boardAction = new WriteAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_write1.jsp";
} else if ( action.equals( "/write_ok.do" ) ) {
boardAction = new WriteOkAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_write1_ok.jsp";
} else if ( action.equals( "/modify.do" ) ) {
boardAction = new ModifyAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_modify1.jsp";
} else if ( action.equals( "/modify_ok.do" ) ) {
boardAction = new ModifyOkAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_modify1_ok.jsp";
} else if ( action.equals( "/delete.do" ) ) {
boardAction = new DeleteAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_delete1.jsp";
} else if ( action.equals( "/delete_ok.do" ) ) {
boardAction = new DeleteOkAction();
boardAction.execute(request, response);
url = "/WEB-INF/views/board_delete1_ok.jsp";
//새로작성
} else if ( action == null || action.equals("/*.json") || action.equals( "/list.json" ) ) {
boardAction = new ListAction();
boardAction.execute(request, response);
url = "/WEB-INF/json/board_list1.jsp";
} else if ( action.equals( "/view.json" ) ) {
boardAction = new ViewAction();
boardAction.execute(request, response);
url = "/WEB-INF/json/board_view1.jsp";
} else if ( action.equals( "/write.json" ) ) {
boardAction = new WriteAction();
boardAction.execute(request, response);
url = "/WEB-INF/json/board_write1.jsp";
} else if ( action.equals( "/write_ok.json" ) ) {
boardAction = new WriteOkAction();
boardAction.execute(request, response);
url = "/WEB-INF/json/board_write1_ok.jsp";
} else if ( action.equals( "/modify.json" ) ) {
boardAction = new ModifyAction();
boardAction.execute(request, response);
url = "/WEB-INF/json/board_modify1.jsp";
} else if ( action.equals( "/modify_ok.json" ) ) {
boardAction = new ModifyOkAction();
boardAction.execute(request, response);
url = "/WEB-INF/json/board_modify1_ok.jsp";
} else if ( action.equals( "/delete.json" ) ) {
boardAction = new DeleteAction();
boardAction.execute(request, response);
url = "/WEB-INF/json/board_delete1.jsp";
} else if ( action.equals( "/delete_ok.json" ) ) {
boardAction = new DeleteOkAction();
boardAction.execute(request, response);
url = "/WEB-INF/json/board_delete1_ok.jsp";
}
RequestDispatcher dispatcher = request.getRequestDispatcher( url );
dispatcher.forward(request, response);
} catch (UnsupportedEncodingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (ServletException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
그리고 json라이브러리를 lib폴더에 넣어준다.

-board_list1.jsp
<%@ page language="java" contentType="text/json; charset=UTF-8"
pageEncoding="UTF-8" trimDirectiveWhitespaces="true"%>
<%@ page import="model1.BoardTO" %>
<%@ page import="java.util.ArrayList" %>
<%@ page import="org.json.simple.JSONArray" %>
<%@ page import="org.json.simple.JSONObject" %>
<%
ArrayList<BoardTO> lists = (ArrayList)request.getAttribute( "lists" );
JSONObject result = new JSONObject();
result.put( "flag", 0 ); //0이면 정상처리
JSONArray jsonArray = new JSONArray();
for( BoardTO to : lists ) {
JSONObject jsonObject = new JSONObject();
jsonObject.put( "seq", to.getSeq() );
jsonObject.put( "subject", to.getSubject() );
jsonObject.put( "writer", to.getWriter() );
jsonObject.put( "wdate", to.getWdate() );
jsonObject.put( "hit", to.getHit() );
jsonObject.put( "wgap", to.getWgap() );
jsonArray.add( jsonObject );
}
result.put( "data", jsonArray );
out.println( result );
%>
웹브라우저에서 아래처럼 주소창에 입력한다.

-board_view.jsp
<%@ page language="java" contentType="text/json; charset=UTF-8"
pageEncoding="UTF-8" trimDirectiveWhitespaces="ture"%>
<%@ page import="model1.BoardTO" %>
<%@ page import="org.json.simple.JSONObject" %>
<%
request.setCharacterEncoding( "utf-8" );
BoardTO to = (BoardTO)request.getAttribute( "to" );
JSONObject result = new JSONObject();
result.put( "flag", 0 ); //0이면 정상처리
JSONObject jsonObject = new JSONObject();
jsonObject.put( "seq", to.getSeq() );
jsonObject.put( "subject", to.getSubject() );
jsonObject.put( "writer", to.getWriter() );
jsonObject.put( "mail", to.getMail() );
jsonObject.put( "wip", to.getWip() );
jsonObject.put( "wdate", to.getWdate() );
jsonObject.put( "hit", to.getHit() );
jsonObject.put( "content", to.getContent() );
result.put( "data", jsonObject );
out.println( result );
%>
-board_write1_ok.jsp(json폴더)
<%@ page language="java" contentType="text/json; charset=UTF-8"
pageEncoding="UTF-8" trimDirectiveWhitespaces="true"%>
<%@ page import="org.json.simple.JSONObject" %>
<%
request.setCharacterEncoding("utf-8");
int flag = (Integer)request.getAttribute( "flag" );
JSONObject result = new JSONObject();
result.put( "flag", flag );
out.println( result );
%>
-board_ui.jsp (웹콘텐츠에 있는 jsp파일)
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="./css/cupertino/jquery-ui.css">
<style type="text/css">
body { font-size: 80%; }
#w_mail1, #w_mail2 { width: 200px; }
#m_mail1, #m_mail2 { width: 200px; }
#accordion > div > div:last-child { text-align: left; }
#accordion-resizer {
margin: 0 60px;
max-width: 1500px;
}
#btngroup {
text-align: right;
}
#btngroup button {
margin: 3px;
padding: 3px;
width: 100px;
}
label.header {
font-size: 10pt;
margin-right: 5px;
}
input.text {
width: 80%;
margin-bottom: 12px;
padding: 0.4em;
}
fieldset {
margin-left: 15px;
margin-top: 15px;
border: 0;
}
</style>
<script type="text/javascript" src="./js/jquery-3.5.1.js"></script>
<script type="text/javascript" src="./js/jquery-ui.js"></script>
<script type="text/javascript">
$( document ).ready( function() {
$( '#writeDialog' ).css( 'display', 'none' );
$( '#modifyDialog' ).css( 'display', 'none' );
$( '#deleteDialog' ).css( 'display', 'none' );
$( '#accordion' ).accordion({
collapsible: 'true',
heightStyle: 'content'
});
$( 'button.action' ).button();
//list and view
const readServer = function() {
$.ajax({
url: 'list.json',
type: 'get',
dataType: 'json',
success: function( json ) {
$('#accordion').empty();
$.each( json.data, function( index, item) {
let title = '<h3>'+item.seq+' : '+ item.writer +'('+ item.hit +') new</h3>';
let html = '<div>';
html += '<div>'+ item.wdate +'</div>';
html += ' <div>'+ item.subject +'</div><br/>';
html += ' <br/>';
html += ' <hr noshade="noshade" />';
html += ' <div>'+ item.content +'</div>';
html += ' <br />';
html += ' <hr noshade="noshade" />';
html += ' <br />';
html += ' <div>';
html += ' <button idx="'+item.seq+'" action="modify" class="action">수정</button>';
html += ' <button idx="'+item.seq+'" action="delete" class="action">삭제</button>';
html += ' </div>';
html += '</div>';
$( '#accordion' ).append( title );
$( '#accordion' ).append( html );
})
$('#accordion').accordion( 'refresh' );
},
error: function() {
alert( '서버 에러' );
}
})
};
//writeOk
const writeOkServer = function() {
$.ajax({
url: 'write_ok.json',
type: 'get',
data: {
subject: $('#w_subject').val(),
writer: $('#w_writer').val(),
mail1: $('#w_mail1').val(),
mail2: $('#w_mail2').val(),
password: $('#w_password').val(),
content: $('#w_content').val()
},
dataType: 'json',
success: function( json ) {
if( json.flag == 0 ) {
alert( '글쓰기에 성공했습니다.' );
$( '#writeDialog').dialog('close');
readServer(); //글쓰기 이후에 다시 글목록창 불러주기
}
},
error: function() {
alert( '서버 에러' );
}
})
}
//delete
const deleteServer = function() {
let seq = $('h3[aria-selected="true"]').text().substring(0,3);
$.ajax({
url: 'delete.json?seq='+seq,
type: 'get',
dataType: 'json',
success: function( json ) {
let html = '<fieldset>';
html += '<div>';
html += ' <label for="subject" class="header">제 목</label>';
html += ' <input type="text" value="'+json.data.subject+'" id="d_subject" class="text ui-widget-content ui-corner-all" readonly="readonly"/>';
html += '</div>';
html += '<div>';
html += ' <label for="password" class="header">비밀 번호</label>';
html += ' <input type="password" id="d_password" class="text ui-widget-content ui-corner-all"/>';
html += '</div>';
html += '</fieldset>';
$('#deleteDialog').html( html );
},
error: function() {
alert( '서버 에러' );
}
})
}
//deleteOk
const deleteOkServer = function() {
let seq = $('h3[aria-selected="true"]').text().substring(0,3);
let password = $('#d_password').val();
$.ajax({
url: 'delete_ok.json?seq='+seq+'&password='+password,
type: 'get',
dataType: 'json',
success: function( json ) {
if( json.flag == 0 ) {
alert( '글삭제에 성공했습니다.' );
$( '#deleteDialog' ).dialog( 'close' );
readServer();
} else {
alert( '비밀번호가 틀렸습니다.' );
}
},
error: function() {
alert( '서버 에러' );
}
})
}
//modify
const modifyServer = function() {
let seq = $('h3[aria-selected="true"]').text().substring(0,3);
$.ajax({
url: 'modify.json?seq='+seq,
type: 'get',
dataType: 'json',
success: function( json ) {
let html = '<fieldset>';
html += '<div>';
html += ' <label for="subject" class="header">제 목</label>';
html += ' <input type="text" id="m_subject" value="'+json.data.subject+'" class="text ui-widget-content ui-corner-all"/>';
html += '</div>';
html += '<div>';
html += ' <label for="writer" class="header">이 름</label>';
html += ' <input type="text" id="m_writer" value="'+json.data.writer+'" class="text ui-widget-content ui-corner-all" readonly="readonly"/>';
html += '</div>';
html += '<div>';
html += ' <label for="mail" class="header">메 일</label>';
html += ' <input type="text" id="m_mail1" value="'+json.data.mail1+'" class="text ui-widget-content ui-corner-all"/> @ ';
html += ' <input type="text" id="m_mail2" value="'+json.data.mail2+'" class="text ui-widget-content ui-corner-all"/>';
html += '</div>';
html += '<div>';
html += ' <label for="password" class="header">비밀 번호</label>';
html += ' <input type="password" id="m_password" class="text ui-widget-content ui-corner-all"/>';
html += '</div>';
html += '<div>';
html += ' <label for="content" class="header">본 문</label>';
html += '<br /><br />';
html += '<textarea rows="15" cols="100" id="m_content" class="text ui-widget-content ui-corner-all">'+json.data.content+'</textarea>';
html += '</div>';
html += '</fieldset>';
$('#modifyDialog').html( html );
},
error: function() {
alert( '서버 에러' );
}
})
}
//modifyOk
const modifyOkServer = function() {
let seq = $('h3[aria-selected="true"]').text().substring(0,3);
let password = $('#m_password').val();
$.ajax({
url: 'modify_ok.json',
type: 'get',
data: {
seq: seq,
password: password,
subject: $('#m_subject').val(),
content: $('#m_content').val(),
mail1: $('#m_mail1').val(),
mail2: $('#m_mail2').val(),
writer: $('#m_writer').val(),
},
dataType: 'json',
success: function( json ) {
if( json.flag == 0 ) {
alert( '글수정에 성공했습니다.' );
$( '#modifyDialog' ).dialog( 'open' );
readServer();
} else {
alert( '비밀번호가 틀렸습니다.' );
}
},
error: function() {
alert( '서버 에러' );
}
})
}
readServer();
$( document ).on( 'click', 'button.action', function() {
if( $( this).attr( 'action' ) == 'write' ) {
$( '#writeDialog' ).dialog ({
width: 800,
height: 700,
modal: true,
buttons: {
'글쓰기': function() {
writeOkServer();
},
'취소': function() {
$( this ).dialog( 'close' );
}
},
close: function() {
}
});
} else if( $( this ).attr( 'action' ) == 'modify' ) {
modifyServer();
$( '#modifyDialog' ).dialog({
width: 800,
height: 700,
modal: true,
buttons: {
'수정': function() {
modifyOkServer();
},
'취소': function() {
$( this ).dialog( 'close' );
}
},
close: function() {
}
});
} else if( $( this ).attr( 'action' ) == 'delete' ) {
deleteServer();
$( '#deleteDialog' ).dialog({
width: 700,
height: 300,
modal: true,
buttons: {
'삭제': function() {
deleteOkServer();
},
'취소': function() {
$( this ).dialog( 'close' );
}
},
close: function() {
}
});
} else {
}
});
});
</script>
</head>
<body>
<div id="accordion-resizer">
<hr noshade="noshade" />
<div id="accordion">
</div>
<hr noshade="noshade" />
<div id="btngroup">
<button action="write" class="action">글쓰기</button>
</div>
</div>
<div id="writeDialog" title="글쓰기">
<fieldset>
<div>
<label for="subject" class="header">제 목</label>
<input type="text" id="w_subject" class="text ui-widget-content ui-corner-all"/>
</div>
<div>
<label for="writer" class="header">이 름</label>
<input type="text" id="w_writer" class="text ui-widget-content ui-corner-all"/>
</div>
<div>
<label for="mail" class="header">메 일</label>
<input type="text" id="w_mail1" class="text ui-widget-content ui-corner-all"/> @
<input type="text" id="w_mail2" class="text ui-widget-content ui-corner-all"/>
</div>
<div>
<label for="password" class="header">비밀 번호</label>
<input type="password" id="w_password" class="text ui-widget-content ui-corner-all"/>
</div>
<div>
<label for="content" class="header">본 문</label>
<br /><br />
<textarea rows="15" cols="100" id="w_content" class="text ui-widget-content ui-corner-all"></textarea>
</div>
</fieldset>
</div>
<div id="modifyDialog" title="글수정">
<!-- <fieldset>
<div>
<label for="subject" class="header">제 목</label>
<input type="text" id="m_subject" class="text ui-widget-content ui-corner-all"/>
</div>
<div>
<label for="writer" class="header">이 름</label>
<input type="text" id="m_writer" class="text ui-widget-content ui-corner-all" readonly="readonly"/>
</div>
<div>
<label for="mail" class="header">메 일</label>
<input type="text" id="m_mail" class="text ui-widget-content ui-corner-all"/>
</div>
<div>
<label for="password" class="header">비밀 번호</label>
<input type="password" id="m_password" class="text ui-widget-content ui-corner-all"/>
</div>
<div>
<label for="content" class="header">본 문</label>
<br /><br />
<textarea rows="15" cols="100" id="m_content" class="text ui-widget-content ui-corner-all"></textarea>
</div>
</fieldset> -->
</div>
<div id="deleteDialog" title="글삭제">
<!-- <fieldset>
<div>
<label for="subject" class="header">제 목</label>
<input type="text" id="d_subject" class="text ui-widget-content ui-corner-all" readonly="readonly"/>
</div>
<div>
<label for="password" class="header">비밀 번호</label>
<input type="password" id="d_password" class="text ui-widget-content ui-corner-all"/>
</div>
</fieldset> -->
</div>
</body>
</html>
추가로 modify와 delete를 만들어보자.
-board_delete1.jsp (json폴더)
<%@ page language="java" contentType="text/json; charset=UTF-8"
pageEncoding="UTF-8" trimDirectiveWhitespaces="true"%>
<%@ page import="model1.BoardTO" %>
<%@ page import="org.json.simple.JSONObject" %>
<%
request.setCharacterEncoding( "utf-8" );
BoardTO to = (BoardTO)request.getAttribute( "to" );
JSONObject result = new JSONObject();
JSONObject jsonObject = new JSONObject();
jsonObject.put( "seq", to.getSeq() );
jsonObject.put( "subject", to.getSubject() );
jsonObject.put( "writer", to.getWriter() );
result.put( "data", jsonObject );
out.println( result );
%>
-board_delete1_ok.jsp (json폴더)
<%@ page language="java" contentType="text/json; charset=UTF-8"
pageEncoding="UTF-8" trimDirectiveWhitespaces="true"%>
<%@ page import="org.json.simple.JSONObject" %>
<%
request.setCharacterEncoding( "utf-8" );
int flag = (Integer)request.getAttribute( "flag" );
JSONObject result = new JSONObject();
result.put( "flag", flag );
out.println( result );
%>
-board_modify1.jsp (json폴더)
<%@ page language="java" contentType="text/json; charset=UTF-8"
pageEncoding="UTF-8" trimDirectiveWhitespaces="false"%>
<%@ page import="model1.BoardTO" %>
<%@ page import="org.json.simple.JSONObject" %>
<%
request.setCharacterEncoding( "utf-8" );
BoardTO to = (BoardTO)request.getAttribute( "to" );
JSONObject result = new JSONObject();
JSONObject jsonObject = new JSONObject();
jsonObject.put( "seq", to.getSeq() );
jsonObject.put( "writer", to.getWriter() );
jsonObject.put( "subject", to.getSubject() );
jsonObject.put( "content", to.getContent() );
String mail[] = null;
if ( to.getMail().equals("") ) {
mail = new String[] { "", "" };
jsonObject.put( "mail1", mail[0] );
jsonObject.put( "mail2", mail[1] );
} else {
jsonObject.put( "mail1", to.getMail().split( "@" )[0] );
jsonObject.put( "mail2", to.getMail().split( "@" )[1] );
}
result.put( "data", jsonObject );
out.println( result );
%>
-board_modify1_ok.jsp(json폴더)
<%@ page language="java" contentType="text/json; charset=UTF-8"
pageEncoding="UTF-8" trimDirectiveWhitespaces="true"%>
<%@ page import="org.json.simple.JSONObject" %>
<%
request.setCharacterEncoding( "utf-8" );
int flag = (Integer)request.getAttribute( "flag" );
String seq = (String)request.getAttribute( "seq" );
JSONObject result = new JSONObject();
result.put( "flag", flag );
out.println( result );
%>